微信小程序圆环进度
时间: 2023-06-21 08:10:04 浏览: 53
要在微信小程序中实现圆环进度,可以使用 `<canvas>` 标签来绘制圆形和弧形,在 JS 中动态设置其属性来实现进度的变化。
以下是一个简单的实现示例:
1. 在 wxml 文件中添加 `<canvas>` 标签,并设置其属性:
```html
<canvas canvas-id="progress" style="width: 200rpx; height: 200rpx;"></canvas>
```
2. 在 js 文件中获取 `<canvas>` 标签,并获取其上下文对象:
```javascript
const ctx = wx.createCanvasContext('progress');
```
3. 在 js 文件中定义一个函数,用于绘制圆形和弧形:
```javascript
function drawCircle(x, y, r, width, startAngle, endAngle, color) {
ctx.beginPath();
ctx.arc(x, y, r, startAngle, endAngle, false);
ctx.setLineWidth(width);
ctx.setStrokeStyle(color);
ctx.stroke();
}
```
4. 在 js 文件中定义一个函数,用于更新进度:
```javascript
function updateProgress(progress) {
// 清空画布
ctx.clearRect(0, 0, 200, 200);
// 绘制底部圆环
drawCircle(100, 100, 90, 10, 0, 2 * Math.PI, '#ccc');
// 绘制进度圆环
drawCircle(100, 100, 90, 10, -Math.PI / 2, -Math.PI / 2 + progress * 2 * Math.PI, '#3ea6ff');
// 绘制进度文字
ctx.setFontSize(30);
ctx.setFillStyle('#3ea6ff');
ctx.setTextAlign('center');
ctx.setTextBaseline('middle');
ctx.fillText((progress * 100).toFixed(0) + '%', 100, 100);
// 绘制完成
ctx.draw();
}
```
5. 在 js 文件中调用 `updateProgress()` 函数来更新进度:
```javascript
// 更新进度为 50%
updateProgress(0.5);
```
以上是一个简单的圆环进度实现示例,你可以根据需求进行修改和优化。
相关推荐
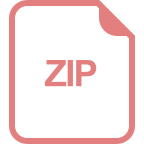














