用c++编写小写字母转换为大写字母
时间: 2024-01-21 11:17:49 浏览: 87
以下是一个简单的 C++ 代码示例,用于将输入的小写字母转换为大写字母:
```cpp
#include <iostream>
#include <cctype>
using namespace std;
int main() {
char c;
cout << "Enter a lowercase letter: ";
cin >> c;
if (islower(c)) {
c = toupper(c);
cout << "The uppercase letter is: " << c << endl;
} else {
cout << "You did not enter a lowercase letter." << endl;
}
return 0;
}
```
该程序首先提示用户输入一个小写字母,然后使用 `islower()` 函数检查用户输入的字符是否为小写字母。如果是,使用 `toupper()` 函数将其转换为大写字母,并输出结果。否则,程序将提示用户重新输入。
相关问题
c++编写程序将小写字母转换为大写字母
在C++中,你可以使用标准库提供的函数`std::toupper`来自头文件`<cctype>`来实现小写字母转大写字母的功能。下面是一个简单的示例:
```cpp
#include <iostream>
#include <cctype> // 引入 toupper 函数
int main() {
std::string input = "hello world"; // 输入的小写字母字符串
std::string output;
for (char& charInString : input) { // 遍历每个字符
if (islower(charInString)) { // 检查是否为小写字母
charInString = toupper(charInString); // 转换为大写字母
}
output += charInString; // 添加到输出字符串中
}
std::cout << "原字符串: " << input << "\n";
std::cout << "大写字符串: " << output << "\n";
return 0;
}
```
在这个程序中,我们遍历输入字符串中的每一个字符,如果它是小写字母(通过`islower`函数检查),我们就使用`toupper`函数将其转换为大写字母。然后把结果添加到`output`字符串中。
用C++编写程序,输入大小写字母混合的字符串,将大写字母转换为小写字母,将小写字母转换为大写字母。然后,输出转换以后的字符串。
在C++中,你可以使用标准库中的`<cctype>`头文件提供的`tolower()`和`toupper()`函数来实现字符的大小写转换。下面是一个简单的示例程序:
```cpp
#include <iostream>
#include <string>
#include <cctype> // 包含tolower()和toupper()
std::string convertCase(const std::string& input) {
std::string output;
for (char c : input) {
if (islower(c)) { // 如果是小写字母
output += toupper(c);
} else if (isupper(c)) { // 如果是大写字母
output += tolower(c);
} else { // 其他字符不变
output += c;
}
}
return output;
}
int main() {
std::string str;
std::cout << "请输入一个大小写字母混合的字符串: ";
std::getline(std::cin, str); // 使用getline获取带空格的字符串
std::string convertedStr = convertCase(str);
std::cout << "转换后的字符串: " << convertedStr << std::endl;
return 0;
}
```
在这个程序中,我们首先读取用户输入的字符串,然后遍历每个字符,如果它是小写字母,就转换为大写;如果是大写字母,就转换为小写;其他字符保持原样。最后,返回转换后的字符串并输出。
阅读全文
相关推荐
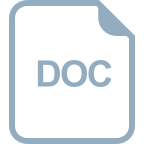
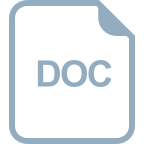
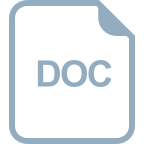



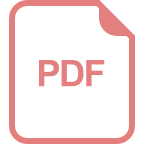









