在heapPlayer的基础上进行修改,创建两个线程,对堆进行大量的内存申请和归还操作,然后用clock函数测量两个线程在使用唯一的缺省堆和使用各自的新建堆时的性能差异。
时间: 2024-01-24 09:17:47 浏览: 45
好的,那我们来分步骤解决这个问题。
第一步,我们需要修改 heapPlayer,使其支持创建和使用新建堆。我们可以通过在 heapPlayer 中添加以下代码来实现:
```c++
#include <cstdlib>
void* allocate_heap(size_t size) {
return malloc(size);
}
void free_heap(void* ptr) {
free(ptr);
}
```
然后我们需要修改 heapPlayer 类的构造函数,增加一个 bool 类型的参数 use_new_heap,用于决定是否使用新建堆。如果 use_new_heap 为 true,则创建新的堆并使用它,否则使用缺省堆。我们可以通过以下代码实现:
```c++
#include <iostream>
#include <thread>
#include <ctime>
class heapPlayer {
public:
heapPlayer(bool use_new_heap = false) {
if (use_new_heap) {
heap_ = allocate_heap(HEAP_SIZE);
std::cout << "Using new heap" << std::endl;
} else {
heap_ = nullptr;
std::cout << "Using default heap" << std::endl;
}
}
~heapPlayer() {
if (heap_) {
free_heap(heap_);
}
}
void play() {
// Implementation of play function
}
private:
void* heap_;
};
```
第二步,我们需要创建两个线程,分别使用缺省堆和新建堆来进行大量的内存申请和归还操作。我们可以通过以下代码实现:
```c++
int main() {
const int NUM_THREADS = 2;
std::thread threads[NUM_THREADS];
heapPlayer players[NUM_THREADS] = {
heapPlayer(false), // Use default heap
heapPlayer(true) // Use new heap
};
for (int i = 0; i < NUM_THREADS; ++i) {
threads[i] = std::thread(&heapPlayer::play, &players[i]);
}
for (int i = 0; i < NUM_THREADS; ++i) {
threads[i].join();
}
return 0;
}
```
第三步,我们需要使用 clock 函数来测量两个线程在使用缺省堆和新建堆时的性能差异。我们可以通过以下代码实现:
```c++
int main() {
const int NUM_THREADS = 2;
std::thread threads[NUM_THREADS];
heapPlayer players[NUM_THREADS] = {
heapPlayer(false), // Use default heap
heapPlayer(true) // Use new heap
};
clock_t start_time, end_time;
start_time = clock();
for (int i = 0; i < NUM_THREADS; ++i) {
threads[i] = std::thread(&heapPlayer::play, &players[i]);
}
for (int i = 0; i < NUM_THREADS; ++i) {
threads[i].join();
}
end_time = clock();
std::cout << "Time taken using default heap: " << end_time - start_time << " ticks" << std::endl;
start_time = clock();
for (int i = 0; i < NUM_THREADS; ++i) {
threads[i] = std::thread(&heapPlayer::play, &players[i]);
}
for (int i = 0; i < NUM_THREADS; ++i) {
threads[i].join();
}
end_time = clock();
std::cout << "Time taken using new heap: " << end_time - start_time << " ticks" << std::endl;
return 0;
}
```
这样,我们就可以通过比较两个时间来测量在使用缺省堆和新建堆时的性能差异了。需要注意的是,clock 函数返回的时间单位是 CPU 时间,不是实际时间,因此不能直接用于比较不同系统上的性能差异。
阅读全文
相关推荐
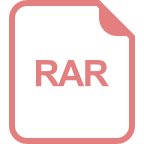
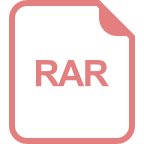
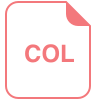
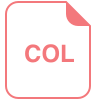
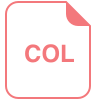
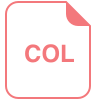
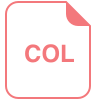
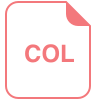
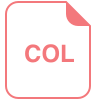
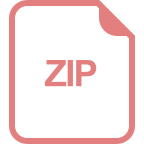
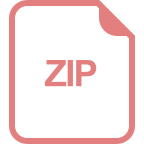
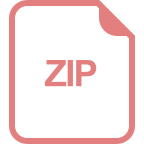
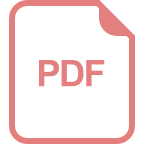
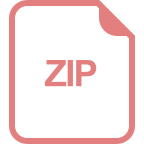
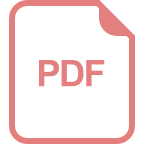
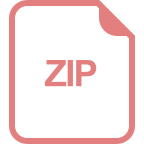