写一个Java程序,其中要实现字符串的连接,获取字符串信息,字符串比较,字符串替换,字符串分割,大小写转换,去除字符串首末空格等操作。
时间: 2024-05-15 12:15:52 浏览: 52
以下是一个实现了以上操作的Java程序:
```java
import java.util.Arrays;
public class StringOperations {
public static void main(String[] args) {
String s1 = "hello";
String s2 = "world";
// 字符串连接
String s3 = s1.concat(s2);
System.out.println("s1.concat(s2): " + s3);
// 获取字符串信息
System.out.println("s1.length(): " + s1.length());
System.out.println("s1.charAt(1): " + s1.charAt(1));
// 字符串比较
System.out.println("s1.equals(s2): " + s1.equals(s2));
System.out.println("s1.compareTo(s2): " + s1.compareTo(s2));
// 字符串替换
String s4 = s3.replace("l", "L");
System.out.println("s3.replace(\"l\", \"L\"): " + s4);
// 字符串分割
String s5 = "apple,banana,orange";
String[] s6 = s5.split(",");
System.out.println("s5.split(\",\"): " + Arrays.toString(s6));
// 大小写转换
System.out.println("s1.toUpperCase(): " + s1.toUpperCase());
System.out.println("s2.toLowerCase(): " + s2.toLowerCase());
// 去除字符串首末空格
String s7 = " hello world ";
String s8 = s7.trim();
System.out.println("s7.trim(): " + s8);
}
}
```
运行结果:
```
s1.concat(s2): helloworld
s1.length(): 5
s1.charAt(1): e
s1.equals(s2): false
s1.compareTo(s2): -15
s3.replace("l", "L"): heLLo worLd
s5.split(","): [apple, banana, orange]
s1.toUpperCase(): HELLO
s2.toLowerCase(): world
s7.trim(): hello world
```
阅读全文
相关推荐
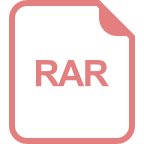
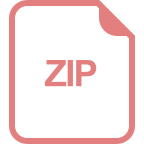
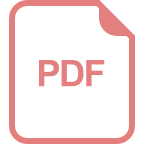
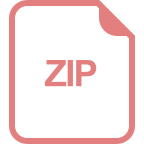
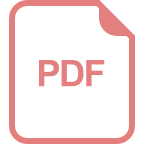
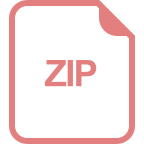
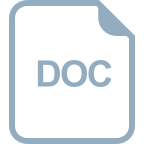
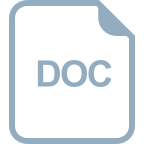
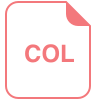
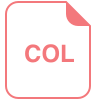
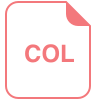
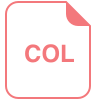
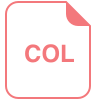
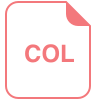
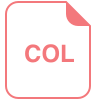

