视差图转为深度,并用open3d显示三维点云效果
时间: 2023-10-02 13:07:34 浏览: 639
要将视差图转换为深度图,可以使用以下公式:
depth = baseline * focal_length / disparity
其中,baseline是双目摄像头的基线距离,focal_length是相机的焦距,disparity是视差值。
然后,您可以使用Open3D库将深度图转换为三维点云,并将其可视化。以下是一个简单的示例代码:
```python
import cv2
import numpy as np
import open3d as o3d
# Load left and right images
img_left = cv2.imread("left.png", cv2.IMREAD_GRAYSCALE)
img_right = cv2.imread("right.png", cv2.IMREAD_GRAYSCALE)
# Compute disparity map
stereo = cv2.StereoBM_create(numDisparities=16, blockSize=15)
disparity = stereo.compute(img_left, img_right)
# Compute depth map
baseline = 0.1 # baseline distance in meters
focal_length = 1000 # focal length in pixels
depth = baseline * focal_length / disparity
# Convert depth map to point cloud
rows, cols = depth.shape
c, r = np.meshgrid(np.arange(cols), np.arange(rows), sparse=True)
x = np.multiply(c - cols/2, depth) / focal_length
y = np.multiply(r - rows/2, depth) / focal_length
z = depth
points = np.dstack((x, y, z)).reshape(-1, 3)
# Create Open3D point cloud
pcd = o3d.geometry.PointCloud()
pcd.points = o3d.utility.Vector3dVector(points)
# Visualize point cloud
o3d.visualization.draw_geometries([pcd])
```
在这个例子中,我们假设有两张名为“left.png”和“right.png”的左右图像。我们首先使用OpenCV的StereoBM算法计算视差图,然后使用上述公式将其转换为深度图。然后,我们使用深度图计算每个像素的三维坐标,并将结果存储在一个numpy数组中。最后,我们使用Open3D创建一个点云对象,并使用“draw_geometries”方法可视化它。
请注意,这只是一个简单的示例代码,可能需要根据您的具体应用程序进行修改。
阅读全文
相关推荐
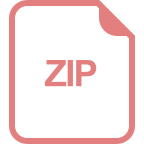

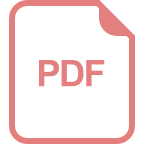
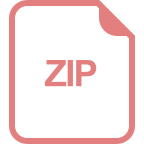
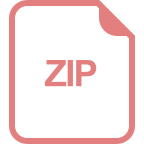
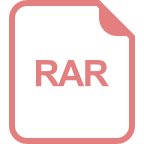
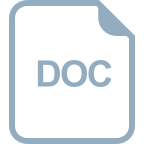
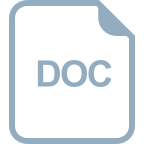
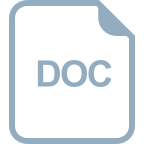





