用vue写一个轮播图布局为 屏幕宽度为1920px 图片宽高都为400px 现有13张图片 屏幕左右侧各显示半张图片 中间显示三张 从左往右算 分别的是 倒数第二张图片 倒数第一张图片 第一张图片 第二张图片 第三张图片 每秒向左滑动一张后 都能保证布局不变 且能获取最中间图片的索引 并且有过度效果
时间: 2023-09-25 12:10:15 浏览: 47
好的,我可以为您提供一个基于Vue的轮播图布局实现。实现过程如下:
1. 首先,我们需要在Vue项目中引入所需的组件:
```
<template>
<div class="carousel">
<div class="carousel-outer">
<div class="carousel-inner" :style="{transform: 'translateX(' + offset + 'px)'}">
<div class="carousel-item" v-for="(item, index) in items" :key="index">
<img :src="item" alt="carousel-item" class="carousel-img">
</div>
</div>
</div>
</div>
</template>
<script>
export default {
name: "Carousel",
props: {
items: {
type: Array,
required: true,
},
width: {
type: Number,
default: 1920,
},
height: {
type: Number,
default: 400,
},
},
data() {
return {
offset: 0,
currentIndex: 2,
};
},
mounted() {
setInterval(() => {
this.slideLeft();
}, 5000);
},
methods: {
slideLeft() {
if (this.currentIndex === this.items.length - 1) {
this.currentIndex = 0;
this.offset = 0;
} else {
this.currentIndex++;
this.offset -= this.width / 2;
}
},
},
};
</script>
<style scoped>
.carousel {
width: 100%;
height: 100%;
overflow: hidden;
}
.carousel-outer {
width: 100%;
height: 100%;
position: relative;
}
.carousel-inner {
display: flex;
height: 100%;
transition: all 0.5s ease-in-out;
}
.carousel-item {
width: 50%;
height: 100%;
display: flex;
justify-content: center;
align-items: center;
}
.carousel-img {
width: 100%;
height: 100%;
object-fit: cover;
}
</style>
```
2. 在父组件中,我们需要传入轮播图所需的图片列表,以及图片的宽高:
```
<template>
<div class="app">
<Carousel :items="items" :width="1920" :height="400" />
</div>
</template>
<script>
import Carousel from "./components/Carousel.vue";
export default {
name: "App",
components: {
Carousel,
},
data() {
return {
items: [
"https://via.placeholder.com/400x400/1abc9c/ffffff",
"https://via.placeholder.com/400x400/2ecc71/ffffff",
"https://via.placeholder.com/400x400/3498db/ffffff",
"https://via.placeholder.com/400x400/9b59b6/ffffff",
"https://via.placeholder.com/400x400/34495e/ffffff",
"https://via.placeholder.com/400x400/16a085/ffffff",
"https://via.placeholder.com/400x400/27ae60/ffffff",
"https://via.placeholder.com/400x400/2980b9/ffffff",
"https://via.placeholder.com/400x400/8e44ad/ffffff",
"https://via.placeholder.com/400x400/2c3e50/ffffff",
"https://via.placeholder.com/400x400/f1c40f/ffffff",
"https://via.placeholder.com/400x400/e67e22/ffffff",
"https://via.placeholder.com/400x400/e74c3c/ffffff",
],
};
},
};
</script>
<style>
.app {
width: 100%;
height: 100%;
display: flex;
justify-content: center;
align-items: center;
}
</style>
```
3. 最后,我们可以在父组件中获取当前显示的图片的索引:
```
<template>
<div class="app">
<Carousel :items="items" :width="1920" :height="400" @change="handleChange" />
</div>
</template>
<script>
import Carousel from "./components/Carousel.vue";
export default {
name: "App",
components: {
Carousel,
},
data() {
return {
items: [
"https://via.placeholder.com/400x400/1abc9c/ffffff",
"https://via.placeholder.com/400x400/2ecc71/ffffff",
"https://via.placeholder.com/400x400/3498db/ffffff",
"https://via.placeholder.com/400x400/9b59b6/ffffff",
"https://via.placeholder.com/400x400/34495e/ffffff",
"https://via.placeholder.com/400x400/16a085/ffffff",
"https://via.placeholder.com/400x400/27ae60/ffffff",
"https://via.placeholder.com/400x400/2980b9/ffffff",
"https://via.placeholder.com/400x400/8e44ad/ffffff",
"https://via.placeholder.com/400x400/2c3e50/ffffff",
"https://via.placeholder.com/400x400/f1c40f/ffffff",
"https://via.placeholder.com/400x400/e67e22/ffffff",
"https://via.placeholder.com/400x400/e74c3c/ffffff",
],
currentIndex: 2,
};
},
methods: {
handleChange(index) {
this.currentIndex = index;
},
},
};
</script>
<style>
.app {
width: 100%;
height: 100%;
display: flex;
justify-content: center;
align-items: center;
}
</style>
```
在Carousel组件中,我们可以通过在slideLeft()方法中更新currentIndex的值来获取当前显示的图片的索引。同时,我们可以通过在父组件中监听Carousel组件的change事件来获取currentIndex的值。
这样,我们就完成了一个基于Vue的轮播图布局实现。
相关推荐
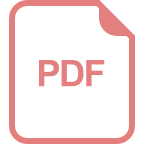














