保存datagriview修改的数据到数据库
时间: 2023-12-20 13:06:59 浏览: 181
保存 DataGridView 中修改的数据到数据库需要以下步骤:
1. 获取 DataGridView 中修改的数据。
可以通过以下代码获取 DataGridView 中修改的数据:
```csharp
foreach (DataGridViewRow row in dataGridView1.Rows)
{
if (row.IsNewRow) continue;
if (row.Cells[0].Value != null)
{
int id = Convert.ToInt32(row.Cells[0].Value);
string name = row.Cells[1].Value.ToString();
int age = Convert.ToInt32(row.Cells[2].Value);
// TODO: 将数据保存到数据库中
}
}
```
2. 将修改的数据保存到数据库中。
可以使用 ADO.NET 或 Entity Framework 等技术将修改的数据保存到数据库中。以下是使用 ADO.NET 的示例代码:
```csharp
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
foreach (DataGridViewRow row in dataGridView1.Rows)
{
if (row.IsNewRow) continue;
if (row.Cells[0].Value != null)
{
int id = Convert.ToInt32(row.Cells[0].Value);
string name = row.Cells[1].Value.ToString();
int age = Convert.ToInt32(row.Cells[2].Value);
// 更新数据
SqlCommand command = new SqlCommand("UPDATE [Table] SET [Name] = @name, [Age] = @age WHERE [ID] = @id", connection);
command.Parameters.AddWithValue("@name", name);
command.Parameters.AddWithValue("@age", age);
command.Parameters.AddWithValue("@id", id);
command.ExecuteNonQuery();
}
else
{
// 插入数据
string name = row.Cells[1].Value.ToString();
int age = Convert.ToInt32(row.Cells[2].Value);
SqlCommand command = new SqlCommand("INSERT INTO [Table] ([Name], [Age]) VALUES (@name, @age)", connection);
command.Parameters.AddWithValue("@name", name);
command.Parameters.AddWithValue("@age", age);
command.ExecuteNonQuery();
}
}
}
```
以上代码中,假设数据库表名为 Table,连接字符串为 connectionString。在更新数据时,使用 UPDATE 语句更新指定 ID 的数据;在插入数据时,使用 INSERT INTO 语句插入新数据。注意要使用参数化查询,避免 SQL 注入攻击。
阅读全文
相关推荐
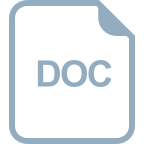
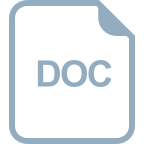
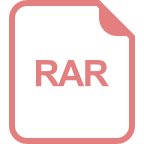
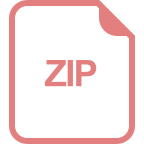
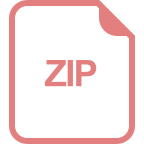
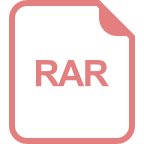
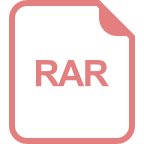


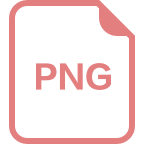
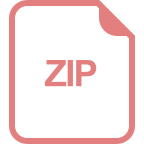
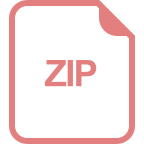
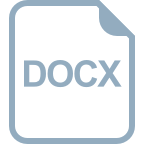