输入5个字符串(长度小于10),比较大小,将最大串和最小串连接,大串在前,小串在后 用c语言
时间: 2023-08-31 18:33:33 浏览: 70
### 回答1:
以下是用 C 语言实现的代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[5][11];
int i, max = 0, min = 0;
// 输入 5 个字符串
for (i = 0; i < 5; i++) {
printf("请输入第 %d 个字符串:", i+1);
scanf("%s", str[i]);
// 更新最大和最小串的下标
if (strlen(str[i]) > strlen(str[max])) {
max = i;
}
if (strlen(str[i]) < strlen(str[min])) {
min = i;
}
}
// 拼接字符串
char result[22];
strcpy(result, str[max]);
strcat(result, str[min]);
// 输出结果
printf("最大串和最小串拼接后的字符串为:%s\n", result);
return 0;
}
```
这个程序会先让用户输入 5 个字符串,同时记录下最大和最小串的下标,然后将其拼接起来并输出。需要注意的是,为了避免越界,我们在定义字符串数组时需要多分配一些空间。此外,为了方便起见,我们假设输入的所有字符串长度都小于等于 10。
### 回答2:
请问需要用C语言编写一个函数,输入5个字符串并比较它们的大小,然后将最大的字符串与最小的字符串连接起来,大串在前,小串在后,最后输出连接后的字符串对吗?
如果是这样的话,可以按照以下流程编写代码实现:
1. 首先,你需要声明5个字符串变量,用于储存输入的5个字符串。假设这些变量分别为str1、str2、str3、str4、str5。
2. 使用scanf函数分别输入这5个字符串,确保每个字符串的长度都小于10。
3. 定义一个字符串数组变量,用于储存最大和最小的字符串。假设这个数组变量为result,大小为20(最大字符串长度的两倍,即10*2)。
4. 首先,将result数组清空,可以使用memset函数将数组中的每个元素设置为0。
5. 通过比较字符串的长度,找到最大和最小的字符串。使用strlen函数获取每个字符串的长度。比较出最大和最小的字符串。
6. 将最大的字符串连接到result数组的前半部分,最小的字符串连接到result数组的后半部分。可以使用strcat函数将字符串连接到result数组中。
7. 最后,使用printf函数输出result数组的值。这就是连接后的字符串。
下面是一个示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str1[10], str2[10], str3[10], str4[10], str5[10];
char result[20];
// 输入5个字符串
printf("请输入5个字符串(长度小于10):\n");
scanf("%s %s %s %s %s", str1, str2, str3, str4, str5);
memset(result, 0, sizeof(result)); // 清空result数组
// 找到最大和最小字符串并连接起来
if (strlen(str1) > strlen(str2)) {
strcpy(result, str1);
strcat(result, str2);
} else {
strcpy(result, str2);
strcat(result, str1);
}
if (strlen(result) < strlen(str3)) {
strcat(result, str3);
} else {
strcat(result, result);
strcpy(result, str3);
}
if (strlen(result) < strlen(str4)) {
strcat(result, str4);
} else {
strcat(result, result);
strcpy(result, str4);
}
if (strlen(result) < strlen(str5)) {
strcat(result, str5);
} else {
strcat(result, result);
strcpy(result, str5);
}
printf("连接后的字符串为:%s\n", result);
return 0;
}
```
以上就是根据你给出的要求编写的一个C语言程序,能够实现输入5个字符串并比较它们的大小,然后将最大的字符串与最小的字符串连接起来,大串在前,小串在后。并输出连接后的字符串。
### 回答3:
请参考以下C语言代码实现:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[5][10];
char maxStr[10], minStr[10]; // 用于保存最大串和最小串
int i;
printf("请输入5个字符串(长度小于10):\n");
for (i = 0; i < 5; i++) {
scanf("%s", str[i]);
}
// 假设第一个字符串既是最大串又是最小串
strcpy(maxStr, str[0]);
strcpy(minStr, str[0]);
for (i = 1; i < 5; i++) {
// 比较当前字符串和最大串的大小
if (strcmp(str[i], maxStr) > 0) {
strcpy(maxStr, str[i]);
}
// 比较当前字符串和最小串的大小
if (strcmp(str[i], minStr) < 0) {
strcpy(minStr, str[i]);
}
}
printf("最大串和最小串连接后的结果为:%s%s\n", maxStr, minStr);
return 0;
}
```
此代码首先使用一个二维字符数组`str`保存5个字符串,然后使用`strcmp()`函数比较字符串大小,找到最大串和最小串,然后使用`strcpy()`函数将最大串和最小串分别复制到`maxStr`和`minStr`中,并最后输出连接后的结果。
相关推荐
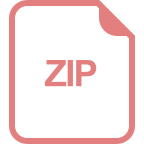
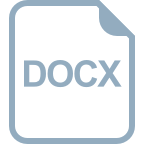
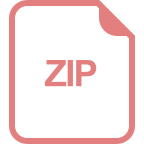














