springboot+thymeleaf+mybatis框架实现删除
时间: 2023-09-07 21:14:34 浏览: 274
要使用SpringBoot+Thymeleaf+MyBatis框架实现删除功能,需要进行以下步骤:
1. 在MyBatis的mapper.xml文件中编写删除语句,例如:
```xml
<delete id="deleteUserById" parameterType="int">
delete from user where id=#{id}
</delete>
```
2. 在对应的Mapper接口中声明该方法:
```java
void deleteUserById(int id);
```
3. 在业务逻辑层(Service)中调用Mapper接口中声明的方法:
```java
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public void deleteUserById(int id) {
userMapper.deleteUserById(id);
}
}
```
4. 在控制器层(Controller)中注入业务逻辑层,并调用相应方法:
```java
@Controller
public class UserController {
@Autowired
private UserService userService;
@RequestMapping("/deleteUser")
public String deleteUser(int id) {
userService.deleteUserById(id);
return "redirect:/userList"; // 重定向到用户列表页面
}
}
```
5. 在Thymeleaf模板中编写删除按钮的HTML代码,并为其添加删除请求的URL:
```html
<form action="/deleteUser" method="post">
<input type="hidden" th:field="${user.id}" />
<button type="submit">删除</button>
</form>
```
在这个例子中,我们需要将用户的id作为隐藏域提交到控制器中,从而实现删除指定id的用户。
这样,就可以实现SpringBoot+Thymeleaf+MyBatis框架下的删除功能了。
阅读全文
相关推荐
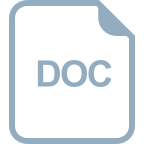
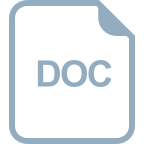
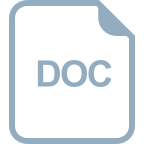
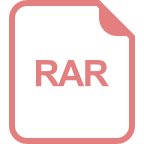
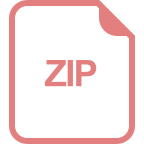
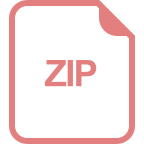
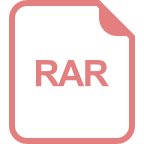
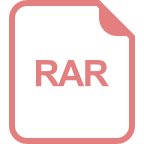
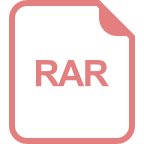
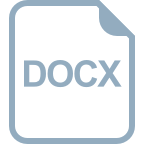
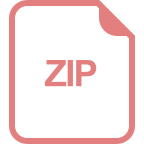
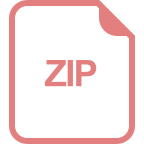
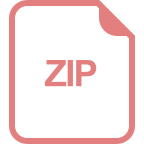
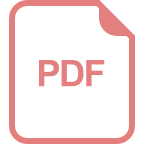
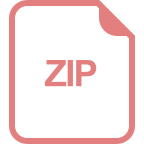
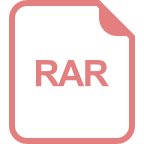
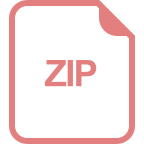
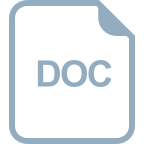
