c语言1.编写程序:输入10个学生的计算机软件基础课程成绩,求平均成绩、最高分,并用选择法对成绩由小到大进行排序。
时间: 2024-01-22 16:20:27 浏览: 100
以下是一份用C语言编写的程序,实现了输入10个学生的成绩,求平均成绩、最高分,并用选择法对成绩由小到大进行排序的功能。
```c
#include <stdio.h>
#define N 10
int main() {
float scores[N], avg_score = 0, max_score = 0;
int i, j, max_index;
// 输入成绩
printf("请输入%d个学生的成绩:\n", N);
for (i = 0; i < N; i++) {
scanf("%f", &scores[i]);
// 统计平均成绩和最高分
avg_score += scores[i];
if (scores[i] > max_score) {
max_score = scores[i];
}
}
avg_score /= N;
// 选择法排序
for (i = 0; i < N - 1; i++) {
max_index = i;
for (j = i + 1; j < N; j++) {
if (scores[j] < scores[max_index]) {
max_index = j;
}
}
// 交换位置
if (max_index != i) {
float temp = scores[i];
scores[i] = scores[max_index];
scores[max_index] = temp;
}
}
// 输出结果
printf("平均成绩为:%.2f\n", avg_score);
printf("最高分为:%.2f\n", max_score);
printf("排序后的成绩为:");
for (i = 0; i < N; i++) {
printf("%.2f ", scores[i]);
}
printf("\n");
return 0;
}
```
程序首先定义了常量N为10,表示有10个学生。然后定义了一个长度为N的float类型数组scores,用于存储学生的成绩。
程序通过for循环输入10个学生的成绩,并在输入每个成绩时统计平均成绩和最高分。
程序接下来使用选择法对成绩由小到大进行排序,最后输出平均成绩、最高分和排序后的成绩。
阅读全文
相关推荐
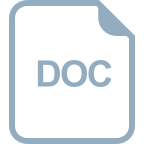
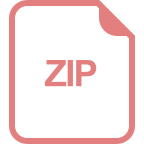
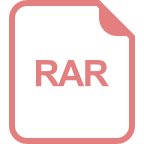
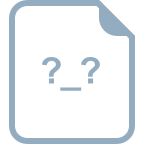
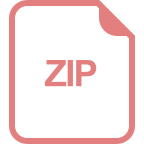
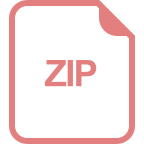
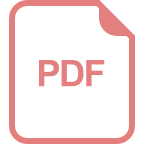
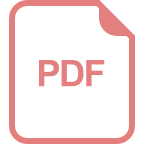
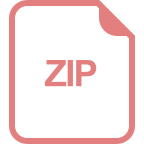