python在文本文档的每一行末尾加上特定的数字
时间: 2024-02-22 20:55:12 浏览: 86
可以使用Python的文件操作来实现在文本文档的每一行末尾加上特定的数字。具体的实现步骤如下:
1. 打开要操作的文本文件,可以使用Python内置的open函数来实现:
```python
file = open("filename.txt", "r+")
```
2. 读取文件中的每一行,可以使用文件对象的readlines()方法:
```python
lines = file.readlines()
```
3. 对每一行末尾加上特定的数字,可以使用字符串的拼接操作:
```python
for i in range(len(lines)):
lines[i] = lines[i].strip() + "特定数字\n"
```
4. 将修改后的内容写回文件中,可以使用文件对象的writelines()方法:
```python
file.seek(0)
file.writelines(lines)
file.close()
```
上述代码中,需要将 "filename.txt" 替换成实际的文件名,特定数字替换成要添加的数字。同时,代码中的 "r+" 表示以读写方式打开文件。
相关问题
python 输出txt文档的指定行的下一行的内容
在Python中,你可以使用内置的`open()`函数打开文本文件,并通过索引来访问特定行。如果你想获取某一行的下一行内容,你需要先读取该行,然后跳过到下一行再读取。这里是一个简单的例子:
```python
def read_next_line(file_path, line_number):
with open(file_path, 'r') as file:
# 逐行读取,每读一行就增加行数
lines = enumerate(file, start=1)
try:
current_line = next(lines) # 获取指定行
next_line = next(lines) # 跳过当前行,获取下一行
return current_line[1], next_line[1] # 返回当前行和下一行的内容
except StopIteration: # 如果文件较小,指定行之后就没有更多行了
return None, None
# 使用方法
file_path = 'example.txt'
line_number_to_read = 5 # 指定要读取的行号,从1开始计数
current_line, next_line = read_next_line(file_path, line_number_to_read)
if current_line is not None:
print(f"第 {line_number_to_read} 行的内容: {current_line.strip()}")
print(f"第 {line_number_to_read + 1} 行的内容: {next_line.strip()}")
else:
print("已经到达文件末尾,没有更多的行.")
用Python写一个程序 抓取文本文档里面的某些行
以下是一个示例程序,它可以抓取一个文本文件中包含特定关键字的行:
```python
# 打开文件
with open('textfile.txt', 'r') as f:
# 遍历每一行
for line in f:
# 如果行包含关键字,打印出来
if 'keyword' in line:
print(line.strip())
```
在这个程序中,我们首先使用 `with` 语句打开了一个文本文件。然后我们使用 `for` 循环遍历文件的每一行。对于每一行,我们检查该行是否包含关键字,如果是,我们就打印出来。注意,我们使用 `strip()` 方法去掉每行末尾的换行符。
你可以将 `keyword` 替换为你自己感兴趣的关键字,或者修改程序以匹配更复杂的模式。
相关推荐
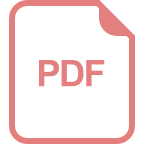
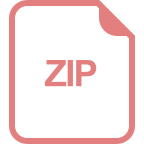
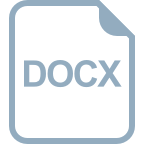
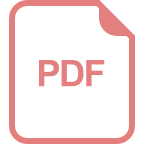
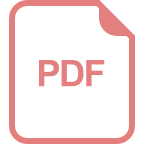
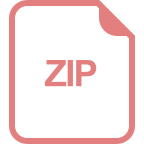
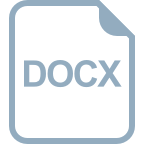
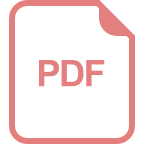
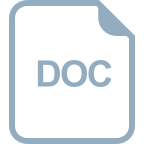
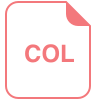
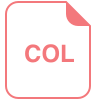
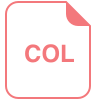
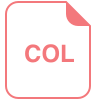