public class listener_of_delete implements ActionListener{ public void actionPerformed(ActionEvent e){ String id = jtId.getText(); DBUtil db = new DBUtil(); String sql = "delete from information_of_grade where id = '"+id+"'"; try { db.getConnection(); db.executeUpdate(sql,null); } catch(Exception ee) { System.out.println(ee.toString()); } finally { db.closeAll(); } JOptionPane.showMessageDialog(null, "删除考生信息"); } } public class AdministrationListener implements ActionListener{ public void actionPerformed(ActionEvent e) { Frame_Of_Delete_Information.this.setVisible(false); new MainFrame_Of_Administration(); } } public void setBak(){ ((JPanel)this.getContentPane()).setOpaque(false); } public static void main(String agrs[]) { new Frame_Of_Delete_Information(); }
时间: 2024-03-26 09:37:22 浏览: 56
这是一段Java代码,包含三个类listener_of_delete、AdministrationListener和Frame_Of_Delete_Information。其中listener_of_delete是一个实现了ActionListener接口的类,当执行动作时,会从文本框中获取id,并从数据库中删除id对应的信息。AdministrationListener是另一个实现了ActionListener接口的类,当执行动作时,会关闭当前窗口并打开一个新的窗口。最后的main函数创建了一个Frame_Of_Delete_Information对象。请问这些类的作用是什么?
相关问题
下面这段代码有什么问题,如何改正import javax.swing.*; import java.awt.*; import java.awt.event.*; class InnerButton{ JFrame frame; JButton b; public static void main(String[] args){ InnerButton gui = new InnerButton(); gui.go(); } public void go(){ frame = new JFrame(); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); b = new JButton("A"); b.addActionListener(); frame.getContentPane().add(BorderLayout.SOUTH,b); frame.setSize(200,100); frame.setVisible(true); } class BListener extends ActionListener{ public void actionPerformed(ActionEvent e){ if(b.getText().equals("A")){ b.setText("B"); } else{ b.setText("A"); } } } }
这段代码存在两个问题:
1. b.addActionListener()方法调用时未传递参数,导致没有为按钮b添加事件监听器。
2. BListener类没有被使用,也就是说,按钮b并没有使用BListener类作为其事件监听器。
要改正这些问题,需要在b.addActionListener()方法中传递一个BListener类的实例,同时将BListener类的实例设置为按钮b的事件监听器。修改后的代码如下所示:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
class InnerButton{
JFrame frame;
JButton b;
public static void main(String[] args){
InnerButton gui = new InnerButton();
gui.go();
}
public void go(){
frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
b = new JButton("A");
b.addActionListener(new BListener()); // 添加事件监听器
frame.getContentPane().add(BorderLayout.SOUTH,b);
frame.setSize(200,100);
frame.setVisible(true);
}
class BListener implements ActionListener{
public void actionPerformed(ActionEvent e){
if(b.getText().equals("A")){
b.setText("B");
}
else{
b.setText("A");
}
}
}
}
```
在修改后的代码中,我们使用了b.addActionListener(new BListener())语句为按钮b添加了事件监听器,并将BListener类的实例作为参数传递给了addActionListener()方法。同时,我们将BListener类设置为按钮b的事件监听器,这样当按钮被点击时,就会执行BListener类中的actionPerformed()方法中的代码。
修改以下代码,使得对于table的若干对键值输入由文件一键读入:package Practise; import javax.swing.*; import java.awt.*; import java.awt.event.*; import java.util.HashMap; class Listener implements ActionListener{ Window window; Listener(Window window){ this.window = window; } @Override public void actionPerformed(ActionEvent e) { if(window.table.containsKey(window.text1.getText())) { window.text2.setText(window.table.get(window.text1.getText())); } else window.text2.setText("Can not translate!"); } } class Window extends JFrame{ JTextField text1 = new JTextField(10); JTextField text2 = new JTextField(10); JButton button1 = new JButton("Translate"); HashMap<String,String> table; Listener listener = new Listener(this); Window(HashMap<String,String> table){ this.table = table; setBounds(160,100,400,300); add(text1); add(button1); button1.addActionListener(listener); add(text2); setDefaultCloseOperation(3); setLayout(new FlowLayout()); setVisible(true); } } public class practise5 { public static void main(String[] args){ HashMap<String,String> table = new HashMap<String, String>(); table.put("one","1"); table.put("two","2"); table.put("three","3"); Window window = new Window(table); } }
以下是修改后的代码:
```java
package Practise;
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.io.File;
import java.io.FileNotFoundException;
import java.util.HashMap;
import java.util.Scanner;
class Listener implements ActionListener {
Window window;
Listener(Window window) {
this.window = window;
}
@Override
public void actionPerformed(ActionEvent e) {
if (window.table.containsKey(window.text1.getText())) {
window.text2.setText(window.table.get(window.text1.getText()));
} else
window.text2.setText("Can not translate!");
}
}
class Window extends JFrame {
JTextField text1 = new JTextField(10);
JTextField text2 = new JTextField(10);
JButton button1 = new JButton("Translate");
HashMap<String, String> table;
Listener listener = new Listener(this);
Window(HashMap<String, String> table) {
this.table = table;
setBounds(160, 100, 400, 300);
add(text1);
add(button1);
button1.addActionListener(listener);
add(text2);
setDefaultCloseOperation(3);
setLayout(new FlowLayout());
setVisible(true);
}
public void readFrom(String fileName) throws FileNotFoundException {
Scanner scanner = new Scanner(new File(fileName));
while (scanner.hasNextLine()) {
String[] pair = scanner.nextLine().split(",");
if (pair.length == 2) {
table.put(pair[0], pair[1]);
}
}
scanner.close();
}
}
public class Practise5 {
public static void main(String[] args) {
HashMap<String, String> table = new HashMap<String, String>();
try {
Window window = new Window(table);
window.readFrom("input.txt");
} catch (FileNotFoundException e) {
System.out.println("File not found!");
}
}
}
```
修改后的代码中,我们添加了一个 `readFrom()` 方法,该方法接受一个文件名作为输入,从文件中读取键值对并将其存储在 `HashMap` 中。
在 `main()` 方法中,我们首先创建了一个空的 `HashMap`,然后创建了一个 `Window` 对象,并调用 `readFrom()` 方法从文件中读取键值对并存储到 `HashMap` 中。最终,我们将 `HashMap` 传递给 `Window` 对象,并显示窗口。
阅读全文
相关推荐








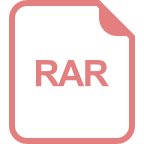





