linux串口输出十六进制编程
时间: 2024-01-10 15:04:22 浏览: 44
在 Linux 中,可以使用 C 语言中的串口读写函数和十六进制转换函数来实现串口输出十六进制编码的功能。下面是一个示例程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
#define SERIAL_PORT "/dev/ttyS0" // 串口设备文件路径
int main(void) {
int fd;
struct termios options;
// 打开串口
fd = open(SERIAL_PORT, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd < 0) {
perror("open");
return -1;
}
// 配置串口
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag &= ~CRTSCTS;
options.c_cflag |= CREAD | CLOCAL;
options.c_iflag &= ~(IXON | IXOFF | IXANY);
options.c_iflag &= ~(ICANON | ECHO | ECHOE | ISIG);
options.c_oflag &= ~OPOST;
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
options.c_cc[VMIN] = 1;
options.c_cc[VTIME] = 0;
tcsetattr(fd, TCSANOW, &options);
// 发送十六进制数据
char hex_str[] = "1a2b3c4d5e";
unsigned char hex_data[6];
int i;
for (i = 0; i < 6; i++) {
sscanf(hex_str + i * 2, "%2hhx", &hex_data[i]);
}
write(fd, hex_data, 6);
// 关闭串口
close(fd);
return 0;
}
```
在上面的示例程序中,首先定义了串口设备文件路径 `SERIAL_PORT`,然后通过 `open` 函数打开串口设备文件并设置相关属性。接着,将需要发送的十六进制字符串转换为十六进制数据并通过 `write` 函数发送出去。最后,通过 `close` 函数关闭串口。
相关推荐
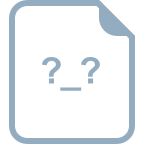














