Write a python script to solve the following problem:Given two sorted arrays nums1 and nums2 of size m and n respectively, return the median of the two sorted arrays. The overall run time complexity should be O(log (m+n))
时间: 2023-03-15 15:55:41 浏览: 126
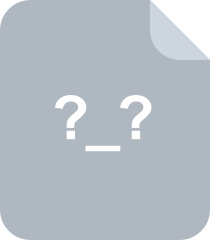
median-of-two-sorted-arrays
这是一个可以用二分法解决的问题。我们可以使用以下步骤来解决这个问题:
1. 将两个数组合并成一个有序数组
2. 计算出这个数组的中位数
下面是一个 Python 的解决方案:
```
import math
def findMedianSortedArrays(nums1, nums2):
# 将两个数组合并成一个有序数组
nums = nums1 + nums2
nums.sort()
# 计算中位数
n = len(nums)
if n % 2 == 0:
# 如果数组长度是偶数,则返回中间两个数的平均值
return (nums[n//2] + nums[n//2 - 1]) / 2
else:
# 如果数组长度是奇数,则返回中间数
return nums[math.floor(n/2)]
```
请注意,这个解决方案的复杂度是 O(m + n),而不是 O(log (m + n))。如果要达到 O(log (m + n)) 的复杂度,可以使用二分法来查找中位数。
阅读全文
相关推荐
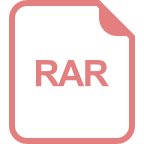
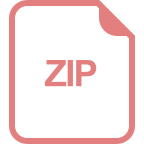
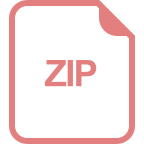
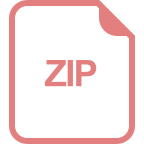
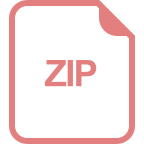
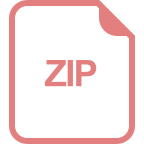
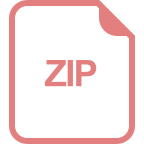
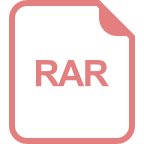
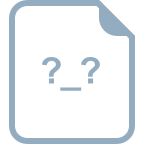
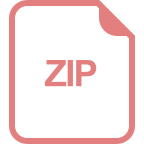
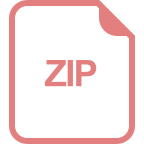
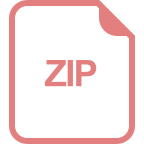
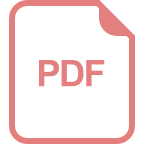
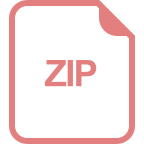
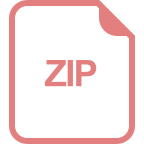