(1)file_search.c实现指定路径下文件名中包含特定字符串(字符串自拟)的文件夹或者文件的搜索,要求能够统计文件名或者文件的数量;
时间: 2024-05-12 18:17:11 浏览: 197
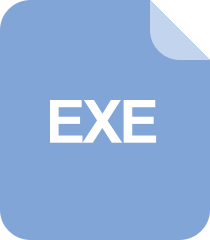
搜索文件夹下包含指定字符串的文件
好的,您的问题是如何在指定路径下搜索文件名包含特定字符串的文件夹或者文件,并统计它们的数量。
这个问题可以通过使用C语言的标准库函数和系统调用来解决。下面是一个简单的代码示例,可以在Linux系统上编译和运行:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <dirent.h>
#include <sys/stat.h>
// 搜索指定路径下文件名包含特定字符串的文件夹或者文件
void search_files(const char* path, const char* substr, int* file_count)
{
DIR* dir = opendir(path);
if (dir == NULL) {
printf("Error: failed to open directory %s\n", path);
return;
}
struct dirent* entry;
while ((entry = readdir(dir))) {
const char* name = entry->d_name;
if (strcmp(name, ".") == 0 || strcmp(name, "..") == 0) {
continue;
}
size_t len = strlen(name);
char subpath[1024];
snprintf(subpath, sizeof(subpath), "%s/%s", path, name);
struct stat st;
if (lstat(subpath, &st) < 0) {
continue;
}
// 如果文件名包含指定的字符串,或者是符号链接并且链接目标文件名包含指定的字符串,则统计数量
if ((strstr(name, substr) != NULL) ||
(S_ISLNK(st.st_mode) && (len > 0) && (name[len-1] != '.') && (strstr(realpath(subpath, NULL), substr) != NULL))) {
(*file_count)++;
if (S_ISDIR(st.st_mode)) { // 如果是文件夹,则递归搜索
search_files(subpath, substr, file_count);
}
}
}
closedir(dir);
}
int main(int argc, char* argv[])
{
if (argc != 3) {
printf("Usage: %s path substr\n", argv[0]);
return 1;
}
const char* path = argv[1];
const char* substr = argv[2];
int file_count = 0;
search_files(path, substr, &file_count);
printf("Found %d files or directories.\n", file_count);
return 0;
}
```
该程序会接受两个命令行参数:路径和要搜索的字符串。它会对指定路径下的所有文件和文件夹进行递归搜索,如果文件名包含指定的字符串,则统计数量。注意,如果文件是符号链接,并且链接目标文件名包含指定的字符串,也会被计算在内。
阅读全文
相关推荐








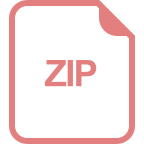






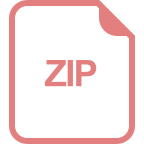