用C语言写代码编程实现: 从键盘输入年份和月份,计算从该年1月1日到当月月底的总天数。 输入格式: 输入某年某月,如:2021 11 输出格式: 从该年1月1日到当月月底的总天数,最后换行。 输入样例: 2021 11 输出样例: 334
时间: 2024-01-24 21:16:44 浏览: 31
以下是用C语言实现的代码:
```c
#include <stdio.h>
int is_leap_year(int year) {
// 判断是否为闰年
if (year % 4 == 0 && year % 100 != 0 || year % 400 == 0) {
return 1;
} else {
return 0;
}
}
int main() {
int year, month;
int days_in_month[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
int total_days = 0;
scanf("%d %d", &year, &month);
// 计算从 1 月到当前月份的总天数
for (int i = 0; i < month - 1; i++) {
total_days += days_in_month[i];
if (i == 1 && is_leap_year(year)) { // 如果是闰年且当前月份是 2 月
total_days++; // 2 月加一天
}
}
printf("%d\n", total_days);
return 0;
}
```
代码思路:
1. 首先定义了一个 `is_leap_year()` 函数,用于判断是否为闰年。
2. 定义 `days_in_month` 数组,存储每个月的天数。
3. 从键盘输入年份和月份。
4. 循环计算从 1 月到当前月份的总天数。如果当前月份是 2 月,还需要判断是否为闰年,如果是闰年则 2 月加一天。
5. 输出总天数。
注意:以上代码中,我们假设输入的年份和月份都是合法的,因此没有做输入验证。如果输入的年份或月份非法,程序可能会出现错误。
相关推荐
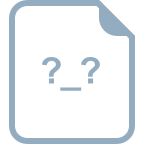
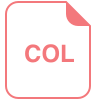
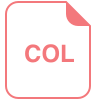
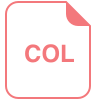
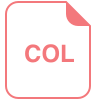
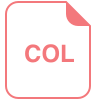









