校验BigDecimal大小
时间: 2023-07-18 17:24:13 浏览: 131
要比较两个 BigDecimal 类型的大小,可以使用 compareTo 方法。该方法返回一个整数值,表示当前 BigDecimal 对象与方法参数比较的大小关系。如果当前对象小于方法参数,则返回负整数;如果当前对象等于方法参数,则返回 0;如果当前对象大于方法参数,则返回正整数。
示例代码如下:
```java
BigDecimal a = new BigDecimal("1.23");
BigDecimal b = new BigDecimal("4.56");
int result = a.compareTo(b);
if(result < 0) {
System.out.println("a 小于 b");
} else if(result == 0) {
System.out.println("a 等于 b");
} else {
System.out.println("a 大于 b");
}
```
在上面的示例中,a 和 b 分别表示两个 BigDecimal 对象,通过调用 a 的 compareTo 方法并传入 b 作为参数,可以得到它们之间的大小关系。最后根据返回值的正负,判断 a 和 b 的大小关系并输出相应的结果。
相关问题
public String asyncNotify(String notifyData) { //1.签名校验 PayResponse payResponse = bestPayService.asyncNotify(notifyData); log.info("异步通知 : notifyData={}", payResponse); //2.金额校验(从数据库查订单) //较为严重(正常情况下是不会发生的)发出警告:钉钉,短信 PayInfoDAO payInfo = payInfoMapper.selectByOrderNo(Long.parseLong(payResponse.getOrderId())); if (payInfo == null) { throw new RuntimeException("通过orderNo查询到的结果为null"); } //如果订单支付状态不是"已支付" if (!payInfo.getPlatformStatus().equals(OrderStatusEnum.SUCCESS.name())) { //Double类型比较大小,精度。1.00 1.0 if (payInfo.getPayAmount().compareTo(BigDecimal.valueOf(payResponse.getOrderAmount())) != 0) { //告警 // throw new RuntimeException("异步通知中的金额和数据库里的不一致,orderNo=" + payResponse.getOrderId()); try { throw new Exception("异步通知中的金额和数据库里的不一致,orderNo=" + payResponse.getOrderId()); } catch (Exception e) { e.printStackTrace(); } } //3. 修改订单支付状态 payInfo.setPlatformStatus(OrderStatusEnum.SUCCESS.name()); payInfo.setPlatformNumber(payResponse.getOutTradeNo()); payInfoMapper.updateByPrimaryKeySelective(payInfo); } //TODO pay发送MQ消息,mall接受MQ int i = sysUserService.updateSysUserByUsername(payInfo.getUserId()); if (i <= 0) { //throw new RuntimeException("更改状态错误"); try { throw new Exception("更改状态错误"); } catch (Exception e) { e.printStackTrace(); } } if (payResponse.getPayPlatformEnum() == BestPayPlatformEnum.WX) { //4.告诉微信不要再通知 return "<xml>\n" + " <return_code><![CDATA[SUCCESS]]></return_code>\n" + " <return_msg><![CDATA[OK]]></return_msg>\n" + "</xml>"; } else if (payResponse.getPayPlatformEnum() == BestPayPlatformEnum.ALIPAY) { //return "success"; return "SUCCESS"; } throw new RuntimeException("异步通知中错误的平台"); } 这个代码有没有什么错误
这段代码存在一些问题:
1. 在发生金额校验不一致时,使用了`try-catch`语句,但是在`catch`块中只是打印了异常堆栈信息,没有进行任何处理,这可能会导致问题被忽略。
2. 在订单支付状态不为"已支付"时,如果金额校验一致,也会更新订单状态,这可能会导致订单状态被错误地修改为"已支付"。
针对这些问题,可以进行以下改进:
1. 在发生金额校验不一致时,不应该使用`try-catch`语句,而是应该直接抛出异常,以便及时发现并处理问题。
2. 在订单支付状态不为"已支付"时,应该只有在金额校验一致的情况下才能更新订单状态。
下面是修改后的代码:
```java
public String asyncNotify(String notifyData) {
//1.签名校验
PayResponse payResponse = bestPayService.asyncNotify(notifyData);
log.info("异步通知 : notifyData={}", payResponse);
//2.金额校验(从数据库查订单)
PayInfoDAO payInfo = payInfoMapper.selectByOrderNo(Long.parseLong(payResponse.getOrderId()));
if (payInfo == null) {
throw new RuntimeException("通过orderNo查询到的结果为null");
}
//如果订单支付状态不是"已支付"
if (!payInfo.getPlatformStatus().equals(OrderStatusEnum.SUCCESS.name())) {
//Double类型比较大小,精度。1.00 1.0
if (payInfo.getPayAmount().compareTo(BigDecimal.valueOf(payResponse.getOrderAmount())) != 0) {
//告警
throw new RuntimeException("异步通知中的金额和数据库里的不一致,orderNo=" + payResponse.getOrderId());
}
//3. 修改订单支付状态
payInfo.setPlatformStatus(OrderStatusEnum.SUCCESS.name());
payInfo.setPlatformNumber(payResponse.getOutTradeNo());
payInfoMapper.updateByPrimaryKeySelective(payInfo);
//TODO pay发送MQ消息,mall接受MQ
int i = sysUserService.updateSysUserByUsername(payInfo.getUserId());
if (i <= 0) {
throw new RuntimeException("更改状态错误");
}
}
if (payResponse.getPayPlatformEnum() == BestPayPlatformEnum.WX) {
//4.告诉微信不要再通知
return "<xml>\n" + " <return_code><![CDATA[SUCCESS]]></return_code>\n" + " <return_msg><![CDATA[OK]]></return_msg>\n" + "</xml>";
} else if (payResponse.getPayPlatformEnum() == BestPayPlatformEnum.ALIPAY) {
return "SUCCESS";
}
throw new RuntimeException("异步通知中错误的平台");
}
```
阅读全文
相关推荐
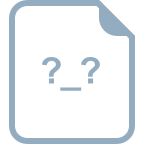
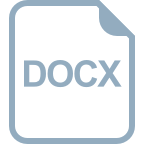
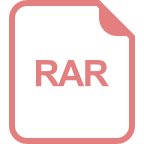
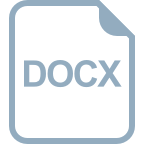
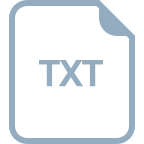
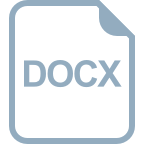
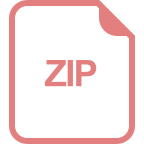
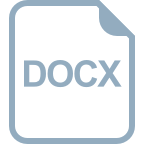
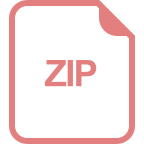
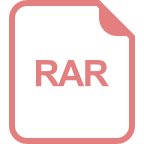
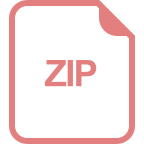
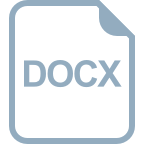
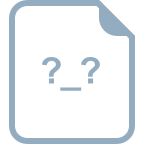