Delegate的paint函数中画俩个radio到item上
时间: 2023-11-28 22:51:21 浏览: 54
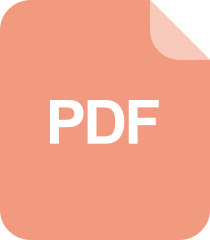
C#使用委托(delegate)实现在两个form之间传递数据的方法
如果您是在使用 WinForms 开发桌面应用程序,可以通过在 `DrawItem` 事件中绘制 `RadioButton` 控件来实现在 `Delegate` 的 `Paint` 函数中画俩个 `RadioButton` 到 `item` 上。
首先,在您的 `Delegate` 类的构造函数中为要绘制的 `RadioButton` 创建实例,并将其添加到您要处理的 `item` 控件中。例如,假设您要在 `ListBox` 中的每个 `item` 上绘制两个 `RadioButton`,并且您已经将 `ListBox` 控件的 `DrawMode` 属性设置为 `DrawMode.OwnerDrawVariable`,则可以在您的 `Delegate` 类的构造函数中添加以下代码:
```csharp
public MyDrawItemDelegate()
{
radioButton1 = new RadioButton();
radioButton2 = new RadioButton();
radioButton1.Text = "RadioButton 1";
radioButton2.Text = "RadioButton 2";
}
private RadioButton radioButton1;
private RadioButton radioButton2;
```
然后,在 `DrawItem` 事件中,您可以使用 `Graphics` 对象的 `DrawString` 方法和 `RadioButton` 对象的 `DrawToBitmap` 方法来绘制 `RadioButton` 控件。例如,以下是一个简单的示例:
```csharp
public void listBox1_DrawItem(object sender, DrawItemEventArgs e)
{
e.DrawBackground();
e.DrawFocusRectangle();
if (e.Index >= 0 && e.Index < listBox1.Items.Count)
{
string itemText = listBox1.Items[e.Index].ToString();
SizeF textSize = e.Graphics.MeasureString(itemText, e.Font);
PointF textLocation = new PointF(e.Bounds.Left, e.Bounds.Top);
// 绘制文本
e.Graphics.DrawString(itemText, e.Font, Brushes.Black, textLocation);
// 绘制第一个RadioButton
radioButton1.Location = new Point((int)textSize.Width + 10, e.Bounds.Top + 2);
Bitmap radioButton1Bitmap = new Bitmap(radioButton1.Width, radioButton1.Height);
radioButton1.DrawToBitmap(radioButton1Bitmap, radioButton1.ClientRectangle);
e.Graphics.DrawImage(radioButton1Bitmap, (int)textSize.Width + 10, e.Bounds.Top + 2);
// 绘制第二个RadioButton
radioButton2.Location = new Point((int)textSize.Width + 10 + radioButton1.Width + 5, e.Bounds.Top + 2);
Bitmap radioButton2Bitmap = new Bitmap(radioButton2.Width, radioButton2.Height);
radioButton2.DrawToBitmap(radioButton2Bitmap, radioButton2.ClientRectangle);
e.Graphics.DrawImage(radioButton2Bitmap, (int)textSize.Width + 10 + radioButton1.Width + 5, e.Bounds.Top + 2);
}
}
```
在上面的代码中,我们首先绘制了 `item` 的文本,然后分别绘制了两个 `RadioButton` 控件。对于每个 `RadioButton`,我们设置其位置,并使用 `DrawToBitmap` 方法将其绘制到一个 `Bitmap` 对象中,然后将该 `Bitmap` 对象绘制到 `Graphics` 对象中。
请注意,上面的代码仅提供了一个简单的示例,并不包括处理 `RadioButton` 的选中状态等功能。如果您需要更复杂的功能,请根据您的具体需求进行修改。
阅读全文
相关推荐
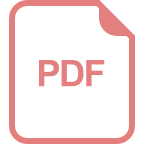
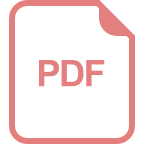
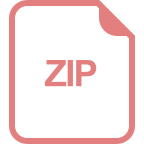
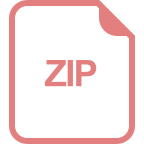
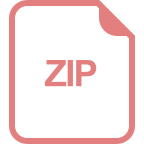
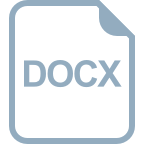
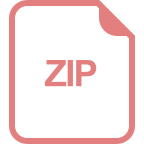
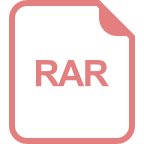
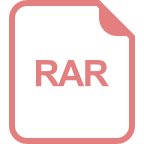
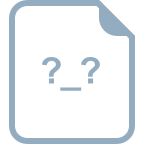
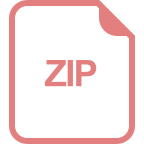
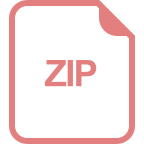
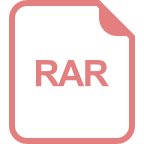
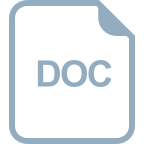
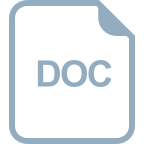
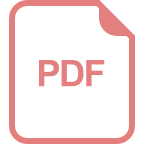
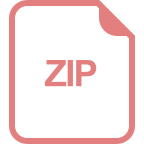