java下载嵌套压缩包得代码
时间: 2023-08-29 08:13:04 浏览: 265
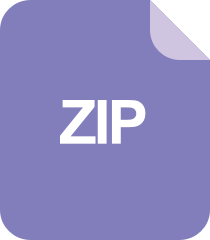
贪吃蛇小游戏 纯java代码 已经编译成.class .zip
### 回答1:
以下是在Java中下载并解压嵌套压缩包的代码示例:
```java
import java.io.*;
import java.net.URL;
import java.nio.file.*;
import java.util.zip.*;
public class Main {
public static void main(String[] args) throws Exception {
String urlString = "https://example.com/nested.zip";
URL url = new URL(urlString);
// 打开输入流并解压第一层压缩包
try (InputStream in = new BufferedInputStream(url.openStream());
ZipInputStream zin = new ZipInputStream(in)) {
ZipEntry entry;
while ((entry = zin.getNextEntry()) != null) {
// 如果是目录则创建
if (entry.isDirectory()) {
Files.createDirectories(Paths.get(entry.getName()));
} else {
// 如果是文件则解压
Path targetPath = Paths.get(entry.getName());
try (OutputStream out = new BufferedOutputStream(
Files.newOutputStream(targetPath))) {
byte[] buffer = new byte[1024];
int count;
while ((count = zin.read(buffer)) != -1) {
out.write(buffer, 0, count);
}
}
}
}
}
// 解压第二层压缩包
Path nestedZipPath = Paths.get("nested.zip");
try (InputStream in = new BufferedInputStream(Files.newInputStream(nestedZipPath));
ZipInputStream zin = new ZipInputStream(in)) {
ZipEntry entry;
while ((entry = zin.getNextEntry()) != null) {
// 如果是目录则创建
if (entry.isDirectory()) {
Files.createDirectories(Paths.get(entry.getName()));
} else {
// 如果是文件则解压
Path targetPath = Paths.get(entry.getName());
try (OutputStream out = new BufferedOutputStream(
Files.newOutputStream(targetPath))) {
byte[] buffer = new byte[1024];
int count;
while ((count = zin.read(buffer)) != -1) {
out.write(buffer, 0, count);
}
}
}
}
}
}
}
```
此代码示例假设压缩包中包含了两层嵌套的压缩包,可以通过更改代码来处理不同的压缩包结构。
### 回答2:
要下载嵌套压缩包的Java代码,可以使用Java的原生类库或第三方库来实现。以下是一个示例代码,使用Java原生的类库来下载嵌套压缩包:
```java
import java.io.*;
import java.net.URL;
import java.nio.file.*;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;
import java.util.zip.ZipOutputStream;
public class DownloadNestedZip {
public static void main(String[] args) {
String url = "http://example.com/nested.zip";
String savePath = "path/to/save/nested.zip";
String extractPath = "path/to/extract";
downloadNestedZip(url, savePath, extractPath);
}
public static void downloadNestedZip(String url, String savePath, String extractPath) {
try {
// 下载压缩包
URL zipUrl = new URL(url);
try (InputStream in = zipUrl.openStream();
FileOutputStream fileOut = new FileOutputStream(savePath)) {
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = in.read(buffer)) > 0) {
fileOut.write(buffer, 0, bytesRead);
}
}
// 解压缩压缩包
Path destination = Paths.get(extractPath);
try (ZipInputStream zipIn = new ZipInputStream(new FileInputStream(savePath))) {
ZipEntry entry;
while ((entry = zipIn.getNextEntry()) != null) {
Path filePath = destination.resolve(entry.getName());
if (entry.isDirectory()) {
Files.createDirectories(filePath);
} else {
Files.createDirectories(filePath.getParent());
try (OutputStream out = Files.newOutputStream(filePath)) {
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = zipIn.read(buffer)) > 0) {
out.write(buffer, 0, bytesRead);
}
}
}
zipIn.closeEntry();
}
}
System.out.println("下载并解压嵌套压缩包成功!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
以上代码通过指定的URL下载嵌套压缩包,并将其保存到指定的路径下,然后解压缩保存的压缩包到指定的目录中。你可以根据实际需求更改URL、保存路径和解压路径。
### 回答3:
要用Java下载嵌套压缩包,首先需要使用Java中的URL类来连接到要下载的文件的URL。接着,可以使用URLConnection类来打开到该URL的连接。通过获取URLConnection对象的输入流,可以读取从该URL下载的文件的内容。
假设要下载的是一个嵌套压缩包,内部有多个压缩文件。可以使用Java的ZipInputStream类来解压缩这个压缩包。首先,需要创建一个ZipInputStream对象,并将从URLConnection对象获取的输入流传递给它。然后,可以使用循环来遍历压缩包中的每个压缩文件。
在循环中,可以使用ZipInputStream的getNextEntry()方法来获取压缩包中的下一个压缩文件的入口(entry)。然后,可以创建一个新的文件输出流(FileOutputStream)来将压缩文件的内容写入到本地文件系统中。
以下是一个简单的示例代码,用于下载并解压一个嵌套压缩包:
```java
import java.io.*;
import java.net.URL;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;
public class NestedZipDownloader {
public static void main(String[] args) {
String urlStr = "https://example.com/nested_zip.zip"; // 要下载的文件的URL
String saveDir = "/path/to/save/directory/"; // 要保存的本地目录
try {
URL url = new URL(urlStr);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
ZipInputStream zipIn = new ZipInputStream(conn.getInputStream());
ZipEntry entry;
// 遍历压缩包中的每个压缩文件
while ((entry = zipIn.getNextEntry()) != null) {
// 创建本地文件
File file = new File(saveDir + File.separator + entry.getName());
// 检查压缩文件所在目录是否存在,若不存在则创建
if (!file.getParentFile().exists()) {
file.getParentFile().mkdirs();
}
// 将压缩文件的内容写入本地文件
OutputStream out = new FileOutputStream(file);
byte[] buffer = new byte[4096];
int bytesRead;
while ((bytesRead = zipIn.read(buffer)) != -1) {
out.write(buffer, 0, bytesRead);
}
out.close();
}
zipIn.close();
conn.disconnect();
System.out.println("下载完成并成功解压所有文件!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在这个示例代码中,`urlStr`是要下载的文件的URL字符串,`saveDir`是要将文件保存到的本地目录的路径。代码会根据URL获取一个URLConnection对象,并打开与该URL的连接。然后,它将URLConnection对象的输入流传递给ZipInputStream来解压缩嵌套压缩包中的文件。然后,它会通过循环遍历每个压缩文件,并将其内容写入到本地文件系统中。
请根据实际情况调整代码中的URL和本地保存目录的路径,以适应您的需求。
阅读全文
相关推荐
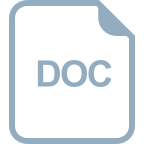
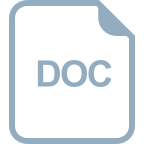

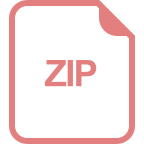
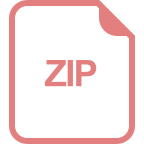
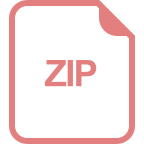
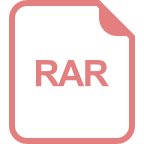
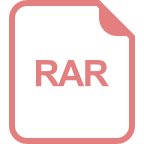
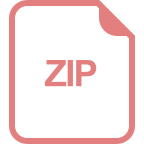
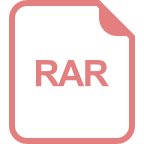
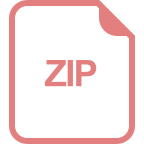
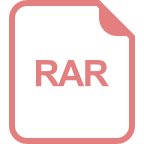
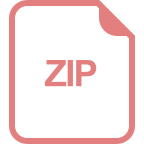
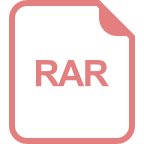
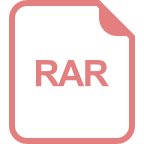
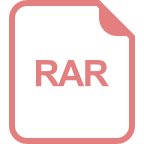
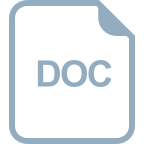
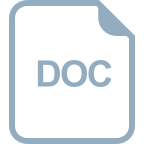