package LZA; public class CRC8 { private static final int POLYNOMIAL = 0x31; private static final int INITIAL_VALUE = 0x00; private static final int FINAL_XOR_VALUE = 0x00; public static int calculateCRC(byte[] data) { int remainder = INITIAL_VALUE; for (byte b : data) { remainder ^= b; for (int bit = 0; bit < 8; bit++) { if ((remainder & 0x80) != 0) { remainder = (remainder << 1) ^ POLYNOMIAL; } else { remainder <<= 1; } } } return remainder ^ FINAL_XOR_VALUE; } }为这段代码生成注释
时间: 2024-02-14 19:14:20 浏览: 76
/**
* CRC8 类用于计算给定字节数组的 CRC8 值。
*/
package LZA;
public class CRC8 {
private static final int POLYNOMIAL = 0x31; // CRC8 多项式
private static final int INITIAL_VALUE = 0x00; // CRC8 初始值
private static final int FINAL_XOR_VALUE = 0x00; // CRC8 最终异或值
/**
* 计算给定字节数组的 CRC8 值。
*
* @param data 给定字节数组
* @return CRC8 值
*/
public static int calculateCRC(byte[] data) {
int remainder = INITIAL_VALUE; // 初始化余数为 CRC8 初始值
// 遍历每一个字节
for (byte b : data) {
remainder ^= b; // 将余数与当前字节进行异或操作
// 遍历当前字节的每一位
for (int bit = 0; bit < 8; bit++) {
if ((remainder & 0x80) != 0) { // 如果余数的最高位为 1
remainder = (remainder << 1) ^ POLYNOMIAL; // 将余数左移一位并异或 CRC8 多项式
} else {
remainder <<= 1; // 否则将余数左移一位
}
}
}
return remainder ^ FINAL_XOR_VALUE; // 返回 CRC8 值
}
}
阅读全文
相关推荐
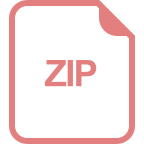
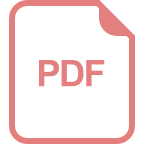
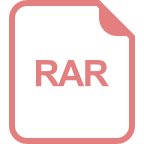
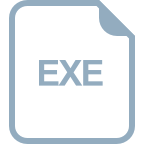

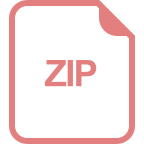
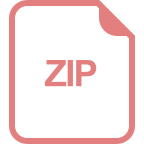
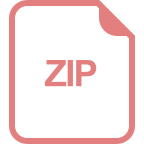
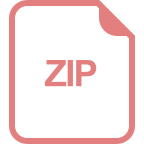
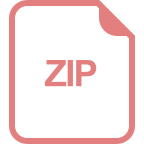