考虑物流成本最低的施工场地布局优化算法,并用遗传算法求解,给出python代码示例
时间: 2023-11-21 07:27:26 浏览: 46
针对物流成本最低的施工场地布局优化问题,可以采用遗传算法进行求解。遗传算法是一种基于生物进化过程的优化算法,通过模拟遗传、变异、选择等过程,寻找最优解。下面是一份Python代码示例,实现了针对施工场地布局的遗传算法优化。
首先,我们需要定义遗传算法中所使用的基本元素:
- 个体:表示一种布局方案,由多个基因组成。
- 基因:表示一个工作站点,包括工作站点的位置和加工时间等信息。
- 种群:由多个个体组成,表示待优化的所有布局方案。
- 适应度函数:用于评估每个个体的适应度,这里采用物流成本作为适应度的评价指标。
- 选择算子:用于从种群中选择一定数量的个体作为下一代个体的父代。
- 交叉算子:用于对父代进行交叉,产生新的个体。
- 变异算子:用于对个体进行变异,产生新的个体。
然后,我们可以按照以下步骤实现遗传算法优化:
1. 初始化种群:随机生成多个个体作为初始种群。
2. 计算适应度:对每个个体进行评估,计算其物流成本。
3. 选择:根据适应度选择一定数量的个体作为下一代个体的父代。
4. 交叉:对父代进行交叉,产生新的个体。
5. 变异:对个体进行变异,产生新的个体。
6. 重复步骤2-5,直到满足停止条件。
下面是一份简单的Python代码示例,实现了上述步骤:
```python
import random
# 工作站点列表
stations = [('A', 3, 2), ('B', 5, 4), ('C', 2, 1), ('D', 4, 3), ('E', 1, 2)]
# 种群大小
POPULATION_SIZE = 50
# 迭代次数
GENERATIONS = 100
# 交叉概率
CROSSOVER_RATE = 0.8
# 变异概率
MUTATION_RATE = 0.2
# 变异范围
MUTATION_RANGE = 2
# 计算物流成本
def calculate_cost(layout):
cost = 0
for i in range(len(layout) - 1):
distance = abs(layout[i][1] - layout[i + 1][0])
cost += distance * layout[i][2]
return cost
# 初始化种群
def create_population(size):
population = []
for i in range(size):
layout = []
for j in range(len(stations)):
position = random.uniform(0, 10)
layout.append((position, stations[j][1], stations[j][2]))
population.append(layout)
return population
# 随机选择
def random_selection(population, fitness):
total_fitness = sum(fitness)
r = random.uniform(0, total_fitness)
index = 0
while r > 0:
r -= fitness[index]
index += 1
index -= 1
return population[index]
# 选择
def selection(population, fitness, size):
new_population = []
for i in range(size):
parent1 = random_selection(population, fitness)
parent2 = random_selection(population, fitness)
new_population.append(parent1 if fitness[population.index(parent1)] > fitness[population.index(parent2)] else parent2)
return new_population
# 交叉
def crossover(parent1, parent2):
if random.random() < CROSSOVER_RATE:
point = random.randint(0, len(parent1) - 1)
child1 = parent1[:point] + parent2[point:]
child2 = parent2[:point] + parent1[point:]
return child1, child2
else:
return parent1, parent2
# 变异
def mutation(layout):
if random.random() < MUTATION_RATE:
index = random.randint(0, len(layout) - 1)
layout[index] = (layout[index][0] + random.uniform(-MUTATION_RANGE, MUTATION_RANGE), layout[index][1], layout[index][2])
return layout
# 遗传算法优化
def genetic_algorithm():
population = create_population(POPULATION_SIZE)
for i in range(GENERATIONS):
fitness = [1 / calculate_cost(layout) for layout in population]
population = selection(population, fitness, POPULATION_SIZE)
for j in range(len(population)):
parent1 = population[j]
parent2 = random_selection(population, fitness)
child1, child2 = crossover(parent1, parent2)
population[j] = mutation(child1)
population.append(mutation(child2))
return max(population, key=lambda x: 1 / calculate_cost(x))
# 输出最优的布局方案
best_layout = genetic_algorithm()
print(best_layout)
```
在上述代码中,我们先定义了工作站点列表和一些遗传算法相关的参数,然后实现了计算物流成本的函数、初始化种群的函数、随机选择、选择、交叉、变异等函数,并最终实现了遗传算法优化函数。在遗传算法优化函数中,我们先对种群进行选择,然后对每个个体进行交叉和变异,最终得到下一代种群,重复这个过程直到满足停止条件,最后输出最优的布局方案。
相关推荐
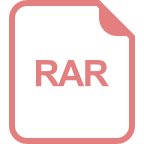
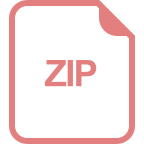














