提供c++代码的opus解码字符串的代码
时间: 2024-06-08 11:09:36 浏览: 21
以下是使用 Opus 库在 C++ 中解码字符串的代码示例:
```c++
#include <opus/opus.h>
#include <string>
std::string decodeOpus(const uint8_t* compressedData, size_t dataSize) {
OpusDecoder* decoder = opus_decoder_create(48000, 2, nullptr);
if (!decoder) {
throw std::runtime_error("Failed to create Opus decoder");
}
const int OPUS_FRAME_SIZE = 960;
const int MAX_SAMPLES_PER_FRAME = OPUS_FRAME_SIZE * 2; // 2 channels
const int MAX_SAMPLES_PER_PACKET = MAX_SAMPLES_PER_FRAME * 60; // 60 frames per packet
const int MAX_OUTPUT_SAMPLES = MAX_SAMPLES_PER_PACKET * 2; // 2 channels
int outputBuffer[MAX_OUTPUT_SAMPLES];
int frameSize = opus_decoder_get_size(2);
if (frameSize < 0) {
opus_decoder_destroy(decoder);
throw std::runtime_error("Failed to get Opus decoder frame size");
}
int decodedSamples = opus_decode(decoder, compressedData, dataSize, outputBuffer, MAX_OUTPUT_SAMPLES, 0);
if (decodedSamples < 0) {
opus_decoder_destroy(decoder);
throw std::runtime_error("Failed to decode Opus data");
}
std::string decodedString(reinterpret_cast<const char*>(outputBuffer), decodedSamples * sizeof(int16_t));
opus_decoder_destroy(decoder);
return decodedString;
}
```
解码过程中的关键步骤:
1. 创建 Opus 解码器实例:`OpusDecoder* decoder = opus_decoder_create(48000, 2, nullptr);`
2. 定义解码缓冲区:`int outputBuffer[MAX_OUTPUT_SAMPLES];`
3. 调用 `opus_decode` 函数解码:`int decodedSamples = opus_decode(decoder, compressedData, dataSize, outputBuffer, MAX_OUTPUT_SAMPLES, 0);`
4. 将解码后的数据转换成字符串:`std::string decodedString(reinterpret_cast<const char*>(outputBuffer), decodedSamples * sizeof(int16_t));`
其中,`compressedData` 是包含 Opus 压缩数据的指针,`dataSize` 是数据大小。函数返回解码后的字符串。
相关推荐
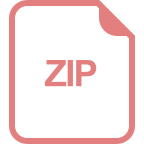














