更复杂的python烟花代码
时间: 2023-08-31 09:39:27 浏览: 109
### 回答1:
以下是一个更复杂的 Python 烟花代码示例:
```python
import random
import math
import turtle
# Set up the turtle
t = turtle.Turtle()
t.speed(0)
t.penup()
t.hideturtle()
# Set up the screen
s = turtle.Screen()
s.bgcolor("black")
s.title("Fireworks")
# Define a function to create a spark at a given position
def spark(x, y, color):
t.penup()
t.goto(x, y)
t.dot(3, color)
# Define a function to create a firework at a given position
def firework(x, y, color):
t.penup()
t.goto(x, y)
t.color(color)
t.dot(5)
for i in range(20):
angle = random.uniform(0, 2*math.pi)
distance = random.uniform(0, 50)
x2 = x + math.cos(angle)*distance
y2 = y + math.sin(angle)*distance
spark(x2, y2, color)
# Create a firework when the user clicks the mouse
def create_firework(x, y):
firework(x, y, (random.random(), random.random(), random.random()))
s.onscreenclick(create_firework)
s.mainloop()
```
这个示例使用了 Python 的 turtle 库来绘制烟花。它定义了两个函数来创建烟花:spark() 和 firework()。spark() 函数在给定坐标处绘制一个小火花,而 firework() 函数在给定坐标处绘制一个大烟花并在周围绘制许多小火花。
最后,该代码还定义了 create_firework() 函数,该函数在用户单击鼠标时创建一个烟花。
### 回答2:
下面是一个更复杂的Python烟花代码示例:
```python
import turtle
import random
import time
# 初始化画布和乌龟
canvas = turtle.Screen()
canvas.bgcolor("black")
canvas.setup(width=800, height=600)
canvas.tracer(0)
pen = turtle.Turtle()
pen.hideturtle()
pen.speed(0)
pen.up()
# 定义烟花爆炸的函数
def explode(x, y, color):
pen.color(color)
pen.goto(x, y)
pen.down()
num_particles = random.randint(30, 50) # 爆炸的粒子数量
for _ in range(num_particles):
pen.setheading(random.randint(0, 360))
pen.fd(random.randint(50, 120))
pen.up()
pen.goto(x, y)
pen.down()
pen.up()
# 定义烟花升起的函数
def launch():
pen.color("white")
x = random.randint(-canvas.window_width() // 2, canvas.window_width() // 2)
y = -canvas.window_height() // 2
pen.goto(x, y)
pen.down()
# 升起
while pen.ycor() < canvas.window_height() // 2:
pen.fd(random.randint(50, 200))
pen.setheading(random.randint(0, 360))
pen.up()
explode(x, pen.ycor(), random.choice(["red", "orange", "yellow", "green", "blue", "purple"]))
# 主循环
while True:
canvas.update()
time.sleep(0.05)
if random.random() < 0.1: # 有一定概率生成烟花
launch()
```
这个代码使用了`turtle`库来绘制烟花的效果。它先定义了一个`explode`函数来绘制烟花爆炸的效果,接着定义了一个`launch`函数来模拟烟花的升起和爆炸过程。然后在主循环中,随机生成烟花并调用`launch`函数来制造烟花效果。通过调整参数可以调整烟花的复杂程度和效果。
相关推荐
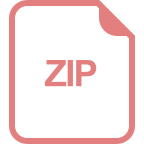
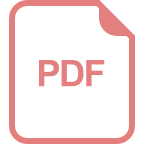











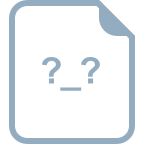