【Django项目开发】自动生成接口文档
时间: 2023-10-14 20:04:52 浏览: 187
在 Django 项目中,可以使用第三方库 `django-rest-swagger` 自动生成接口文档。
以下是具体的步骤:
1. 安装 `django-rest-swagger`
```bash
pip install django-rest-swagger
```
2. 将 `rest_framework` 和 `rest_framework_swagger` 添加到 Django 项目的 `INSTALLED_APPS` 中:
```python
INSTALLED_APPS = [
# ...
'rest_framework',
'rest_framework_swagger',
# ...
]
```
3. 在 Django 项目的 `urls.py` 中添加 Swagger API URL:
```python
from rest_framework_swagger.views import get_swagger_view
schema_view = get_swagger_view(title='API 文档')
urlpatterns = [
# ...
url(r'^api-docs/$', schema_view),
# ...
]
```
4. 在需要生成文档的 API 视图中添加 `swagger_auto_schema` 装饰器:
```python
from rest_framework.decorators import api_view
from rest_framework.response import Response
from rest_framework import status
from rest_framework_swagger import swagger_auto_schema
@api_view(['GET'])
@swagger_auto_schema(
operation_description='获取用户信息',
responses={
status.HTTP_200_OK: '返回用户信息',
status.HTTP_404_NOT_FOUND: '用户不存在'
}
)
def get_user(request, user_id):
try:
user = User.objects.get(id=user_id)
except User.DoesNotExist:
return Response({'error': 'User does not exist'}, status=status.HTTP_404_NOT_FOUND)
return Response({
'id': user.id,
'username': user.username,
'email': user.email
}, status=status.HTTP_200_OK)
```
5. 运行 Django 项目,在浏览器中访问 Swagger API URL:`http://localhost:8000/api-docs/`,即可查看生成的接口文档。
注意事项:
- 如果需要在文档中显示请求参数、响应数据的数据类型和描述信息,需要在对应的 API 视图中使用 `serializer_class` 属性指定序列化器。例如:
```python
@api_view(['POST'])
@swagger_auto_schema(
operation_description='创建用户',
request_body=UserSerializer,
responses={
status.HTTP_201_CREATED: '用户创建成功',
status.HTTP_400_BAD_REQUEST: '请求参数不合法'
}
)
def create_user(request):
serializer = UserSerializer(data=request.data)
if serializer.is_valid():
serializer.save()
return Response(serializer.data, status=status.HTTP_201_CREATED)
else:
return Response(serializer.errors, status=status.HTTP_400_BAD_REQUEST)
```
- `swagger_auto_schema` 装饰器还支持其他参数,例如 `tags`、`operation_id` 等,可以根据实际需求进行设置。详细文档可以参考 `django-rest-swagger` 的官方文档。
阅读全文
相关推荐




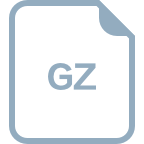










