C#遍历句柄下所有子句柄
时间: 2024-02-05 15:41:02 浏览: 19
在C#中,可以使用Win32 API函数来遍历窗口句柄下的所有子句柄。以下是一个示例代码,可以遍历窗口句柄下的所有子句柄:
```
using System;
using System.Runtime.InteropServices;
class Program
{
[DllImport("user32.dll")]
static extern IntPtr GetWindow(IntPtr hWnd, uint uCmd);
[DllImport("user32.dll")]
static extern IntPtr GetWindowThreadProcessId(IntPtr hWnd, out uint lpdwProcessId);
[DllImport("user32.dll")]
static extern bool EnumWindows(EnumWindowsProc lpEnumFunc, IntPtr lParam);
[DllImport("user32.dll")]
static extern bool EnumChildWindows(IntPtr hWndParent, EnumWindowsProc lpEnumFunc, IntPtr lParam);
delegate bool EnumWindowsProc(IntPtr hWnd, IntPtr lParam);
static bool EnumChildWindowsCallback(IntPtr hWnd, IntPtr lParam)
{
uint processId;
GetWindowThreadProcessId(hWnd, out processId);
Console.WriteLine("Found child window handle: 0x{0:X} (Process ID: {1})", hWnd.ToInt32(), processId);
return true;
}
static bool EnumWindowsCallback(IntPtr hWnd, IntPtr lParam)
{
EnumChildWindows(hWnd, EnumChildWindowsCallback, IntPtr.Zero);
return true;
}
static void Main(string[] args)
{
IntPtr desktopHandle = GetWindow(IntPtr.Zero, 5); // 5 == GW_CHILD
EnumWindows(EnumWindowsCallback, desktopHandle);
Console.ReadLine();
}
}
```
在这个示例代码中,使用了以下三个Win32 API函数:
- GetWindow:获取与给定窗口具有指定关系的窗口句柄。在本例中,使用GW_CHILD参数获取窗口句柄下的第一个子句柄。
- GetWindowThreadProcessId:获取窗口所属的进程ID。
- EnumChildWindows:枚举指定父窗口的子窗口,通过回调函数EnumChildWindowsCallback来处理每个子窗口的句柄。
在Main函数中,首先获取桌面窗口的第一个子窗口句柄,然后调用EnumWindows函数,通过回调函数EnumWindowsCallback来枚举所有子窗口的句柄,并通过回调函数EnumChildWindowsCallback来处理每个子窗口的句柄。
当找到一个子窗口时,会调用EnumChildWindowsCallback函数,输出子窗口的句柄和所属进程的ID。
相关推荐
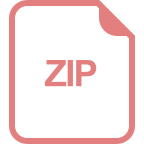














