MFC如何将多张图片上传到 http://169.254.1.10:8090/api/v1/~bali/ABC0123456789
时间: 2023-10-05 08:07:10 浏览: 152
MFC可以通过使用WinINet API来实现HTTP上传文件。以下是一个基本示例代码,可以上传多张图片到指定的URL:
```c++
#include <afxinet.h>
void PostFilesToUrl(const CString& strUrl, const CStringArray& arrFilePaths)
{
HINTERNET hInternet = InternetOpen(_T("MFC WinINet"), INTERNET_OPEN_TYPE_PRECONFIG, NULL, NULL, 0);
if (!hInternet)
return;
HINTERNET hConnect = InternetConnect(hInternet, _T("169.254.1.10"), 8090, NULL, NULL, INTERNET_SERVICE_HTTP, 0, 0);
if (!hConnect)
{
InternetCloseHandle(hInternet);
return;
}
HINTERNET hRequest = HttpOpenRequest(hConnect, _T("POST"), _T("/api/v1/~bali/ABC0123456789"), NULL, NULL, NULL, 0, 0);
if (!hRequest)
{
InternetCloseHandle(hConnect);
InternetCloseHandle(hInternet);
return;
}
CString strBoundary = _T("----Boundary-") + COleDateTime::GetCurrentTime().Format(_T("%Y%m%d%H%M%S"));
CString strContentType = _T("multipart/form-data; boundary=");
strContentType += strBoundary;
CString strHeaders = _T("Content-Type: ");
strHeaders += strContentType;
if (!HttpSendRequest(hRequest, strHeaders, strHeaders.GetLength(), NULL, 0))
{
InternetCloseHandle(hRequest);
InternetCloseHandle(hConnect);
InternetCloseHandle(hInternet);
return;
}
DWORD dwBytesWritten = 0;
for (int i = 0; i < arrFilePaths.GetCount(); i++)
{
CString strFilePath = arrFilePaths[i];
CString strFileName = strFilePath.Right(strFilePath.GetLength() - strFilePath.ReverseFind('\\') - 1);
CString strHeader = _T("\r\n--") + strBoundary + _T("\r\n");
strHeader += _T("Content-Disposition: form-data; name=\"file\"; filename=\"");
strHeader += strFileName + _T("\"\r\n");
strHeader += _T("Content-Type: application/octet-stream\r\n\r\n");
HANDLE hFile = CreateFile(strFilePath, GENERIC_READ, FILE_SHARE_READ, NULL, OPEN_EXISTING, FILE_ATTRIBUTE_NORMAL, NULL);
if (hFile == INVALID_HANDLE_VALUE)
continue;
DWORD dwFileSize = GetFileSize(hFile, NULL);
if (!HttpWriteFile(hRequest, strHeader, strHeader.GetLength(), &dwBytesWritten, NULL))
{
CloseHandle(hFile);
continue;
}
char* pBuffer = new char[dwFileSize];
DWORD dwBytesRead = 0;
if (!ReadFile(hFile, pBuffer, dwFileSize, &dwBytesRead, NULL))
{
CloseHandle(hFile);
delete[] pBuffer;
continue;
}
if (!HttpWriteFile(hRequest, pBuffer, dwFileSize, &dwBytesWritten, NULL))
{
CloseHandle(hFile);
delete[] pBuffer;
continue;
}
delete[] pBuffer;
CloseHandle(hFile);
}
CString strFooter = _T("\r\n--") + strBoundary + _T("--\r\n");
HttpWriteFile(hRequest, strFooter, strFooter.GetLength(), &dwBytesWritten, NULL);
InternetCloseHandle(hRequest);
InternetCloseHandle(hConnect);
InternetCloseHandle(hInternet);
}
```
使用示例:
```c++
CStringArray arrFilePaths;
arrFilePaths.Add(_T("C:\\image1.jpg"));
arrFilePaths.Add(_T("C:\\image2.jpg"));
PostFilesToUrl(_T("http://169.254.1.10:8090/api/v1/~bali/ABC0123456789"), arrFilePaths);
```
这将上传`C:\image1.jpg`和`C:\image2.jpg`两张图片到指定URL。注意修改URL和文件路径为你自己的。
阅读全文
相关推荐
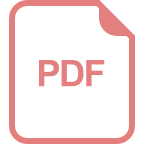
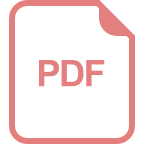
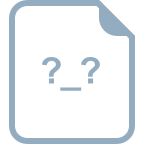















