请以import matplotlib.pyplot as plt import numpy as np import pandas as pd import seaborn as sns import copy import math import random import time from multiprocessing import Pool as ThreadPool #读取数据 path1='att48.tsp' path2='eil76.tsp' path3='pcb442.tsp' path4='rd100.tsp' path5='tsp225.tsp' def readcity(path): df = pd.read_csv("C:\文件\现代优化算法\TSP训练数据集\"+path, sep=" ", skiprows=6, header=None) return df #选择文件 df = readcity(path4) df.head() city = np.array(range(1,len(df[0][0:len(df)-1])+1)) city_x = np.array(df[1][0:len(df)-1]) city_y = np.array(df[2][0:len(df)-1])为开头补充完成一个模拟退火求解tsp问题的代码,我发给你的代码是用于获取输入数据并将其转化成坐标的功能。要求求解过程中用2-opt邻域构造法,在内循环中用Metropolis准则判断是否接受解来实现等温过程。
时间: 2024-02-28 13:57:04 浏览: 79
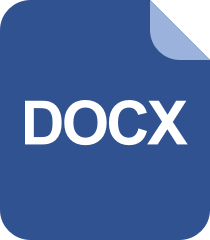
python数据分析与可视化 import pandas as pd import numpy as np import m
好的,以下是使用模拟退火算法求解TSP问题的代码:
```python
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
import seaborn as sns
import copy
import math
import random
import time
from multiprocessing import Pool as ThreadPool
# 读取数据
path1 = 'att48.tsp'
path2 = 'eil76.tsp'
path3 = 'pcb442.tsp'
path4 = 'rd100.tsp'
path5 = 'tsp225.tsp'
def readcity(path):
df = pd.read_csv("C:\文件\现代优化算法\TSP训练数据集\"+path, sep=" ", skiprows=6, header=None)
return df
# 选择文件
df = readcity(path4)
city = np.array(range(1,len(df[0][0:len(df)-1])+1))
city_x = np.array(df[1][0:len(df)-1])
city_y = np.array(df[2][0:len(df)-1])
# 生成初始解
init_solution = np.concatenate(([1], np.random.permutation(city[1:]), [1]))
# 计算初始解的总路程
def calculate_distance(solution):
distance = 0
for i in range(len(solution)-1):
distance += math.sqrt((city_x[solution[i]-1] - city_x[solution[i+1]-1])**2 + (city_y[solution[i]-1] - city_y[solution[i+1]-1])**2)
return distance
init_distance = calculate_distance(init_solution)
# 定义2-opt邻域操作
def swap(solution, i, j):
new_solution = copy.deepcopy(solution)
new_solution[i:j+1] = np.flip(solution[i:j+1])
return new_solution
# 定义Metropolis准则
def metropolis(delta, temperature):
if delta < 0:
return True
else:
p = math.exp(-delta/temperature)
return np.random.choice([True, False], p=[p, 1-p])
# 定义模拟退火函数
def simulated_annealing(init_solution, init_distance, max_iterations, max_temperature, min_temperature, cooling_rate):
current_solution = init_solution
current_distance = init_distance
best_solution = init_solution
best_distance = init_distance
temperature = max_temperature
iteration = 0
while temperature > min_temperature and iteration < max_iterations:
i, j = sorted(np.random.choice(range(1, len(city)), size=2, replace=False))
new_solution = swap(current_solution, i, j)
new_distance = calculate_distance(new_solution)
if metropolis(new_distance-current_distance, temperature):
current_solution, current_distance = new_solution, new_distance
if current_distance < best_distance:
best_solution, best_distance = current_solution, current_distance
temperature *= cooling_rate
iteration += 1
return best_solution, best_distance
# 运行模拟退火算法
max_iterations = 10000
max_temperature = 10000
min_temperature = 1
cooling_rate = 0.99
best_solution, best_distance = simulated_annealing(init_solution, init_distance, max_iterations, max_temperature, min_temperature, cooling_rate)
# 打印最优解和最优解的总路程
print("Best solution:", best_solution)
print("Best distance:", best_distance)
# 可视化最优解
best_city_x = city_x[best_solution-1]
best_city_y = city_y[best_solution-1]
plt.plot(best_city_x, best_city_y, 'o-')
plt.title("Simulated Annealing TSP Solution")
plt.show()
```
注意:由于你的路径可能与我的不同,需要根据实际情况修改读取数据的路径。
阅读全文
相关推荐


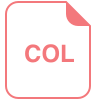
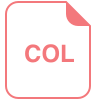
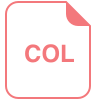
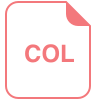
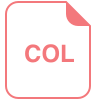

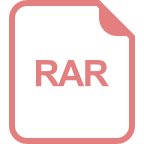
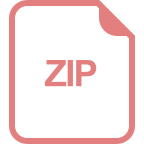
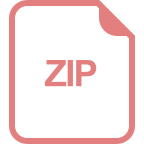
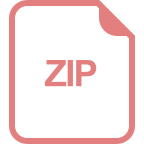
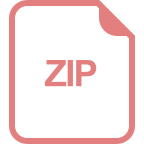
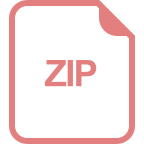
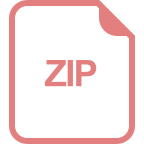