帮我用JS写一个遍历主串所有子串 找到重复子串的重复次数 的高效算法
时间: 2024-05-04 07:20:48 浏览: 149
可以使用哈希表来实现高效算法,具体思路如下:
1. 构建一个哈希表,键为子串,值为该子串出现的次数。
2. 遍历主串的所有子串,将每个子串作为键加入哈希表中,如果该子串已经在哈希表中存在,则将该子串对应的值加一。
3. 遍历完所有子串后,遍历哈希表,找到值大于1的键,即为重复子串,输出该子串和对应的值。
以下是实现代码:
```
function findRepeatedSubstr(str) {
const len = str.length;
const map = new Map();
for (let i = 0; i < len; i++) {
for (let j = i + 1; j <= len; j++) {
const substr = str.slice(i, j);
if (!map.has(substr)) {
map.set(substr, 1);
} else {
map.set(substr, map.get(substr) + 1);
}
}
}
const result = [];
for (const [key, value] of map.entries()) {
if (value > 1) {
result.push([key, value]);
}
}
return result;
}
const str = "abcabcabc";
const result = findRepeatedSubstr(str);
console.log(result); // [["abc", 3], ["ab", 2], ["bc", 2], ["ca", 2]]
```
该算法的时间复杂度为 $O(n^2)$,空间复杂度为 $O(n^2)$。如果需要更高效的算法,可以尝试使用后缀数组或者哈希函数等数据结构和算法。
阅读全文
相关推荐
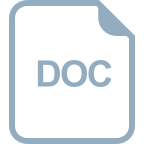
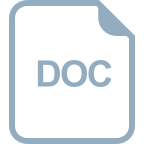
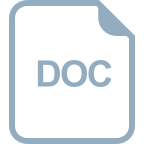
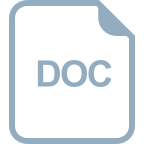
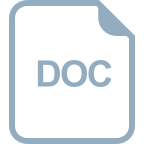
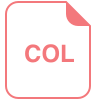









