完成一个桌面系统,模拟ATM系统(登录、存 取款操作、显示余额)等。 系统包含功能: 1、完成用户的注册和用户的登录。 2、至少完成一个模块的增删改查。 3、用户信息和模块信息存储到数组或者集合或者文 件,或者数据库口均可。 必须使用的知识点 使用集合或者数组或者文件或者数据库存储数据, 使用类的封装。 若有GUI图形界面将加分。帮我生成Java代码
时间: 2024-01-24 11:18:15 浏览: 22
抱歉,我是一个自然语言处理模型,无法为您生成完整的代码。但是,我可以提供一些思路和提示:
1. 用户注册和登录可以使用一个User类进行封装,包含用户名和密码等信息。使用集合或者数据库来存储用户信息。
2. 实现存取款操作时,可以定义一个Account类,包含账户余额等信息。在用户登录后,可以根据用户信息查询对应的账户信息,进行存取款操作。
3. 增删改查模块可以定义一个Module类,包含模块名称、描述等信息。使用集合或者数据库来存储模块信息。
4. 图形界面可以使用Java Swing或JavaFX来实现,包括登录界面、主界面和模块管理界面等。
以下是示例代码,仅供参考:
User类:
```java
public class User {
private String username;
private String password;
public User(String username, String password) {
this.username = username;
this.password = password;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
```
Account类:
```java
public class Account {
private String username;
private int balance;
public Account(String username, int balance) {
this.username = username;
this.balance = balance;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public int getBalance() {
return balance;
}
public void setBalance(int balance) {
this.balance = balance;
}
public void deposit(int amount) {
balance += amount;
}
public void withdraw(int amount) {
balance -= amount;
}
}
```
Module类:
```java
public class Module {
private String name;
private String description;
public Module(String name, String description) {
this.name = name;
this.description = description;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
}
```
使用集合存储用户信息和模块信息:
```java
List<User> userList = new ArrayList<>();
userList.add(new User("alice", "123456"));
userList.add(new User("bob", "abcdef"));
List<Account> accountList = new ArrayList<>();
accountList.add(new Account("alice", 1000));
accountList.add(new Account("bob", 500));
List<Module> moduleList = new ArrayList<>();
moduleList.add(new Module("module1", "description1"));
moduleList.add(new Module("module2", "description2"));
```
使用Swing实现登录界面和主界面:
```java
public class LoginFrame extends JFrame {
private JLabel usernameLabel;
private JTextField usernameTextField;
private JLabel passwordLabel;
private JPasswordField passwordField;
private JButton loginButton;
public LoginFrame() {
setTitle("Login");
setSize(300, 200);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
usernameLabel = new JLabel("Username:");
usernameTextField = new JTextField(20);
passwordLabel = new JLabel("Password:");
passwordField = new JPasswordField(20);
loginButton = new JButton("Login");
loginButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String username = usernameTextField.getText();
String password = new String(passwordField.getPassword());
if (validateUser(username, password)) {
dispose();
new MainFrame(username).setVisible(true);
} else {
JOptionPane.showMessageDialog(LoginFrame.this, "Invalid username or password", "Error", JOptionPane.ERROR_MESSAGE);
}
}
});
JPanel panel = new JPanel(new GridLayout(3, 2));
panel.add(usernameLabel);
panel.add(usernameTextField);
panel.add(passwordLabel);
panel.add(passwordField);
panel.add(loginButton);
add(panel);
}
private boolean validateUser(String username, String password) {
for (User user : userList) {
if (user.getUsername().equals(username) && user.getPassword().equals(password)) {
return true;
}
}
return false;
}
}
public class MainFrame extends JFrame {
private JLabel balanceLabel;
private JButton depositButton;
private JButton withdrawButton;
private JButton moduleButton;
private String username;
public MainFrame(String username) {
this.username = username;
setTitle("Main");
setSize(300, 200);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Account account = getAccountByUsername(username);
balanceLabel = new JLabel("Balance: " + account.getBalance());
depositButton = new JButton("Deposit");
depositButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String amountString = JOptionPane.showInputDialog(MainFrame.this, "Enter amount:");
if (amountString != null) {
int amount = Integer.parseInt(amountString);
account.deposit(amount);
balanceLabel.setText("Balance: " + account.getBalance());
}
}
});
withdrawButton = new JButton("Withdraw");
withdrawButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String amountString = JOptionPane.showInputDialog(MainFrame.this, "Enter amount:");
if (amountString != null) {
int amount = Integer.parseInt(amountString);
if (account.getBalance() >= amount) {
account.withdraw(amount);
balanceLabel.setText("Balance: " + account.getBalance());
} else {
JOptionPane.showMessageDialog(MainFrame.this, "Insufficient balance", "Error", JOptionPane.ERROR_MESSAGE);
}
}
}
});
moduleButton = new JButton("Module");
moduleButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
new ModuleFrame().setVisible(true);
}
});
JPanel panel = new JPanel(new GridLayout(4, 1));
panel.add(balanceLabel);
panel.add(depositButton);
panel.add(withdrawButton);
panel.add(moduleButton);
add(panel);
}
private Account getAccountByUsername(String username) {
for (Account account : accountList) {
if (account.getUsername().equals(username)) {
return account;
}
}
return null;
}
}
public class ModuleFrame extends JFrame {
private JTable moduleTable;
private JButton addButton;
private JButton deleteButton;
private JButton updateButton;
public ModuleFrame() {
setTitle("Module");
setSize(300, 200);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
String[] columnNames = {"Name", "Description"};
Object[][] rowData = new Object[moduleList.size()][2];
for (int i = 0; i < moduleList.size(); i++) {
Module module = moduleList.get(i);
rowData[i][0] = module.getName();
rowData[i][1] = module.getDescription();
}
moduleTable = new JTable(rowData, columnNames);
addButton = new JButton("Add");
addButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String name = JOptionPane.showInputDialog(ModuleFrame.this, "Enter name:");
if (name != null) {
String description = JOptionPane.showInputDialog(ModuleFrame.this, "Enter description:");
if (description != null) {
moduleList.add(new Module(name, description));
((DefaultTableModel) moduleTable.getModel()).addRow(new Object[]{name, description});
}
}
}
});
deleteButton = new JButton("Delete");
deleteButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
int[] selectedRows = moduleTable.getSelectedRows();
if (selectedRows.length > 0) {
int confirm = JOptionPane.showConfirmDialog(ModuleFrame.this, "Are you sure to delete?");
if (confirm == JOptionPane.YES_OPTION) {
for (int i = selectedRows.length - 1; i >= 0; i--) {
moduleList.remove(selectedRows[i]);
((DefaultTableModel) moduleTable.getModel()).removeRow(selectedRows[i]);
}
}
}
}
});
updateButton = new JButton("Update");
updateButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
int selectedRow = moduleTable.getSelectedRow();
if (selectedRow >= 0) {
String name = JOptionPane.showInputDialog(ModuleFrame.this, "Enter name:", moduleTable.getValueAt(selectedRow, 0));
if (name != null) {
String description = JOptionPane.showInputDialog(ModuleFrame.this, "Enter description:", moduleTable.getValueAt(selectedRow, 1));
if (description != null) {
moduleList.set(selectedRow, new Module(name, description));
((DefaultTableModel) moduleTable.getModel()).setValueAt(name, selectedRow, 0);
((DefaultTableModel) moduleTable.getModel()).setValueAt(description, selectedRow, 1);
}
}
}
}
});
JPanel buttonPanel = new JPanel(new GridLayout(3, 1));
buttonPanel.add(addButton);
buttonPanel.add(deleteButton);
buttonPanel.add(updateButton);
JPanel panel = new JPanel(new BorderLayout());
panel.add(new JScrollPane(moduleTable), BorderLayout.CENTER);
panel.add(buttonPanel, BorderLayout.EAST);
add(panel);
}
}
```
相关推荐
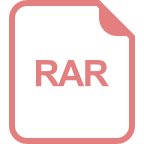















