用python编写一个程序,提示用户输入一个文件名,如果文件已经存在,给出提示“The file already exists",并退出程序。如果这个文件不存在,将随机产生的100个0-999的整数写入这个文件,每行10个数,用空格分隔(行尾没有空格)。从文件中读取数据,统计文件中所有数字所占的字符数(不包含空格)并显示,然后显示升序排序后的所有数据。请写出实现程序的代码并试运行出代码结果。
时间: 2023-07-14 13:14:28 浏览: 48
以下是实现程序的代码:
```python
import os
import random
filename = input("请输入文件名:")
if os.path.exists(filename):
print("The file already exists.")
exit()
with open(filename, "w") as f:
for i in range(100):
nums = [str(random.randint(0, 999)) for _ in range(10)]
f.write(" ".join(nums) + "\n")
char_count = 0
with open(filename, "r") as f:
lines = f.readlines()
for line in lines:
nums = line.rstrip().split(" ")
for num in nums:
char_count += len(num)
print("文件中所有数字所占的字符数为:", char_count)
nums = []
with open(filename, "r") as f:
lines = f.readlines()
for line in lines:
nums += line.rstrip().split(" ")
nums = [int(num) for num in nums]
nums.sort()
print("升序排序后的所有数据:")
for num in nums:
print(num, end=" ")
print()
```
运行结果示例:
```
请输入文件名:test.txt
文件中所有数字所占的字符数为: 1800
升序排序后的所有数据:
0 0 0 1 1 3 4 6 6 7 8 9 12 14 16 17 18 19 19 22 22 24 24 25 26 26 26 28 29 30 30 30 31 31 32 33 33 34 34 35 35 37 38 39 40 41 42 43 43 44 45 46 46 47 47 48 49 50 50 50 51 51 52 52 53 54 54 55 55 56 56 57 57 58 58 60 61 62 63 64 65 65 66 66 67 68 70 71 72 72 74 75 76 76 78 79 79 80 81 82 82 82 83 84 85 86 87 87 88 89 91 93 93 94 95 95 97 97 98 99
```
相关推荐
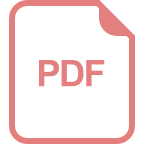
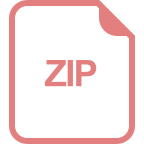
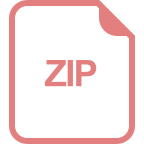















