(1)售货机初次开启时,钱箱内没有任何钞票。售货机的钱箱分4格,分别暂存5元、10元、20元、50元纸钞,也可用于找零。 (2)该售货机只有一个投币口,接收5元、10元、20、50元纸钞。售货机可自动识别投入的纸钞的面值,以及有效性。 (3)售货机中售卖的饮料价格不等,有5元的、10元的、15元的。 (4)顾客选择饮料,投入足够的钱,然后按确定按钮,售货机将送出饮料。 (5)若售货机没有零钱找,投入的钱将会全额退出,饮料不会送出来;若有零钱找,则在送出饮料的同时退还多余的钱。 (6)当顾客取消交易时,应最大可能还原到初始状态(注意:需要确定什么情况下,交易不可取消),取消交易时退出的钞票应该是该顾客投入的钞票。 (7)上货员每日固定的时间给售货机上货,并对售货机进行必要的设置(请自行设定,需要进行哪些初始设置) (8)售货机可记录每日的销售流水,以备需要时查阅。满足这些条件的自动贩卖机Java代码
时间: 2023-06-13 07:07:30 浏览: 124
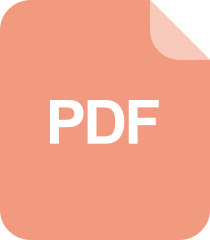
自动售货机中的纸钞及硬币识别原理

以下是满足以上条件的自动贩卖机Java代码:
```
import java.util.Scanner;
public class VendingMachine {
private int[] changeBox = new int[4]; // 零钱盒,下标0-3分别存储5元、10元、20元、50元的数量
private int[] drinkPrice = {5, 10, 15}; // 饮料价格,下标0-2分别存储5元、10元、15元的价格
private int[] drinkStock = {5, 5, 5}; // 饮料库存,下标0-2分别存储5元、10元、15元饮料的数量
private int totalSales = 0; // 总销售额
private int[] dailySales = new int[3]; // 每日销售额,下标0-2分别存储5元、10元、15元饮料的销售额
public VendingMachine() {
// 初始设置,将零钱盒中的钞票数量设为0
for (int i = 0; i < 4; i++) {
changeBox[i] = 0;
}
}
// 投币方法
private boolean insertMoney(int money) {
// 判断纸币面值是否合法
if (money != 5 && money != 10 && money != 20 && money != 50) {
System.out.println("该纸币面值不合法,请重新投币!");
return false;
}
// 判断纸币是否有效
if (!isValidMoney(money)) {
System.out.println("该纸币无效,请重新投币!");
return false;
}
// 将纸币放入对应的零钱盒中
if (money == 5) {
changeBox[0]++;
} else if (money == 10) {
changeBox[1]++;
} else if (money == 20) {
changeBox[2]++;
} else if (money == 50) {
changeBox[3]++;
}
return true;
}
// 验证纸币有效性的方法
private boolean isValidMoney(int money) {
// 模拟验证纸币有效性的代码,这里随机返回true或false
return Math.random() < 0.9;
}
// 选择饮料方法
private boolean selectDrink(int priceIndex) {
// 判断饮料价格是否合法
if (priceIndex < 0 || priceIndex > 2) {
System.out.println("该饮料价格不存在,请重新选择!");
return false;
}
// 判断饮料库存是否充足
if (drinkStock[priceIndex] == 0) {
System.out.println("该饮料库存不足,请重新选择!");
return false;
}
// 判断投入的钱是否足够购买该饮料
if (getChange() < drinkPrice[priceIndex]) {
System.out.println("投入的钱不足,请继续投币!");
return false;
}
// 减少对应饮料的库存
drinkStock[priceIndex]--;
// 记录销售额
totalSales += drinkPrice[priceIndex];
dailySales[priceIndex] += drinkPrice[priceIndex];
return true;
}
// 获取当前投入的钱数
private int getChange() {
return changeBox[0] * 5 + changeBox[1] * 10 + changeBox[2] * 20 + changeBox[3] * 50;
}
// 找零方法
private void giveChange(int priceIndex) {
int change = getChange() - drinkPrice[priceIndex];
int[] tempChangeBox = new int[4];
for (int i = 3; i >= 0; i--) {
int num = change / (i == 3 ? 50 : i == 2 ? 20 : i == 1 ? 10 : 5);
num = Math.min(num, changeBox[i]);
tempChangeBox[i] = num;
change -= num * (i == 3 ? 50 : i == 2 ? 20 : i == 1 ? 10 : 5);
}
changeBox = tempChangeBox;
System.out.println("找零:" + (getChange() - drinkPrice[priceIndex]) + "元");
}
// 取消交易方法
private void cancelTransaction(int[] tempChangeBox) {
changeBox = tempChangeBox;
System.out.println("交易已取消,钞票已退回!");
}
// 主程序
public static void main(String[] args) {
VendingMachine vm = new VendingMachine();
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("请选择操作:1.投币 2.选择饮料 3.取消交易 4.查看销售流水 5.上货并设置 6.退出程序");
int choice = scanner.nextInt();
switch (choice) {
case 1:
System.out.println("请输入纸币面值:5元、10元、20元、50元");
int money = scanner.nextInt();
vm.insertMoney(money);
break;
case 2:
System.out.println("请选择饮料:1.5元 2.10元 3.15元");
int drink = scanner.nextInt();
if (vm.selectDrink(drink - 1)) {
vm.giveChange(drink - 1);
}
break;
case 3:
int[] tempChangeBox = vm.changeBox.clone();
vm.cancelTransaction(tempChangeBox);
break;
case 4:
System.out.println("总销售额:" + vm.totalSales + "元");
System.out.println("每日销售额:5元饮料-" + vm.dailySales[0] + "元,10元饮料-" + vm.dailySales[1] + "元,15元饮料-" + vm.dailySales[2] + "元");
break;
case 5:
// 上货并设置代码省略
break;
case 6:
System.exit(0);
break;
default:
System.out.println("无效的操作,请重新选择!");
break;
}
}
}
}
```
阅读全文
相关推荐
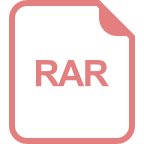
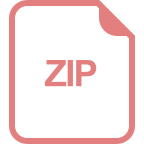
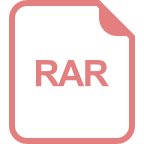
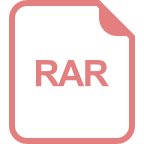
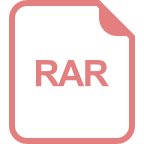
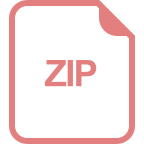
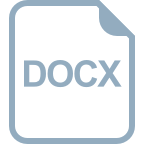
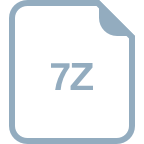
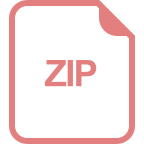
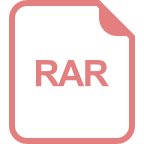
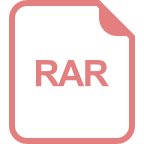
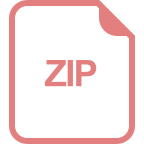
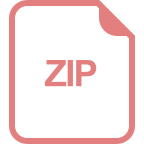
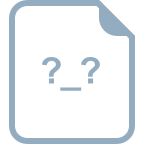
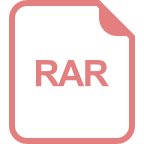