qt5回车读取spinbox内容
时间: 2023-07-18 18:05:24 浏览: 265
你可以使用 `QSpinBox` 的 `value()` 函数来获取它的当前值。此外,你可以使用 `QSpinBox` 的 `editingFinished()` 信号来捕获用户完成编辑并按下回车键的事件。以下是一个示例:
```cpp
// 创建一个 SpinBox 对象
QSpinBox* spinBox = new QSpinBox(parent);
// 连接 editingFinished() 信号到槽函数
connect(spinBox, &QSpinBox::editingFinished, this, [=]() {
int value = spinBox->value(); // 获取 SpinBox 的当前值
// 处理获取到的值
});
```
在上面的示例中,当用户完成编辑并按下回车键时,将调用 Lambda 表达式中的代码。`spinBox->value()` 将返回 SpinBox 的当前值,你可以在 Lambda 表达式中处理该值。
相关问题
qt5回车发送spinbox内容
你可以使用Qt的信号槽机制,将spinbox的valueChanged()信号连接到发送按钮的clicked()信号上,这样当用户点击回车键时,spinbox的值会被自动发送。
以下是一个示例代码:
```cpp
// 创建SpinBox和PushButton
QSpinBox *spinBox = new QSpinBox(this);
QPushButton *sendButton = new QPushButton("Send", this);
// 连接信号槽
connect(spinBox, static_cast<void(QSpinBox::*)(int)>(&QSpinBox::valueChanged), [=](int value){
// 将SpinBox的值设置为按钮的快捷键
sendButton->setShortcut(QKeySequence(QString::number(value)));
});
connect(sendButton, &QPushButton::clicked, [=](){
QString text = spinBox->text();
// 发送文本
});
```
在这个示例中,当SpinBox的值改变时,我们将其设置为发送按钮的快捷键。然后,当用户按下回车键时,按钮就会被触发,发送SpinBox的值。
qt中, 读取SpinBox的值,并保存到一个文件中
以下是将SpinBox的值读取并保存到文件中的示例代码:
```python
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QSpinBox, QPushButton, QVBoxLayout
class Example(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.spinbox = QSpinBox(self)
self.spinbox.setMinimum(1)
self.spinbox.setMaximum(100)
self.spinbox.setValue(50)
save_btn = QPushButton('Save', self)
save_btn.clicked.connect(self.save_value)
vbox = QVBoxLayout()
vbox.addWidget(self.spinbox)
vbox.addWidget(save_btn)
self.setLayout(vbox)
self.setGeometry(300, 300, 250, 150)
self.setWindowTitle('SpinBox Example')
self.show()
def save_value(self):
value = self.spinbox.value()
with open('value.txt', 'w') as f:
f.write(str(value))
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = Example()
sys.exit(app.exec_())
```
在这个例子中,我们创建了一个SpinBox和一个保存按钮。当用户单击保存按钮时,我们使用SpinBox的value()方法来获取其值,并将其写入名为"value.txt"的文件中。
阅读全文
相关推荐
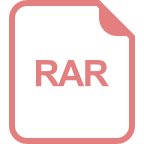
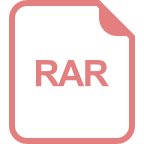
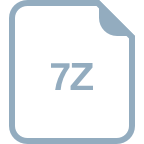













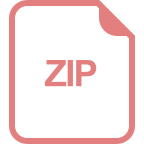