一、课程设计目的 通过磁盘调度算法的模拟设计,了解磁盘调度的特点。 容 二、课程设计内容 模拟实现 FCFS、SSTF、电梯 LOOK、C-SCAN 算法,并计算及比较磁头移动道数。 示 三、要求及提示 本题目必须单人完成。 1、首先假设磁盘磁道数为 1500,磁头初始位置可任意设置。 2、用随机数生成函数产生“磁道号”序列(即磁盘请求的位置),共产生 400 个。其中 50%位于 0~499,25%分布在 500~999,25%分布在 1000~1499。具体的产生方法可参考 “题目一 页面置换算法的模拟实现及命中率对比”。 3、计算及比较每种磁盘调度算法下的磁头移动道数。 注:本题目要求给出图形可视化界面,并且能够动态模拟每个算法的调度过程,可采用 从上一个请求到下一个请求进行连线的方式。根据以上内容生成C语言程序
时间: 2024-03-31 10:33:28 浏览: 136
好的,以下是一个简单的C语言程序,实现了FCFS、SSTF、电梯LOOK、C-SCAN算法的模拟,并计算比较了磁头移动道数。同时,程序使用了图形库SDL,实现了图形可视化界面,能够动态模拟每个算法的调度过程。
```
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <SDL2/SDL.h>
#define SCREEN_WIDTH 640
#define SCREEN_HEIGHT 480
#define RECT_WIDTH 10
#define RECT_HEIGHT 10
#define REQUEST_NUM 400
#define TRACK_NUM 1500
int requests[REQUEST_NUM];
int current_track = 0;
int direction = 1;
int fcfs_pos = 0;
int sstf_pos = 0;
int look_pos = 0;
int cscan_pos = 0;
int fcfs_track_moves = 0;
int sstf_track_moves = 0;
int look_track_moves = 0;
int cscan_track_moves = 0;
SDL_Window *window;
SDL_Renderer *renderer;
void init()
{
srand(time(NULL));
for (int i = 0; i < REQUEST_NUM; i++)
{
int r = rand() % 100;
if (r < 50)
{
requests[i] = rand() % 500;
}
else if (r < 75)
{
requests[i] = 500 + rand() % 500;
}
else
{
requests[i] = 1000 + rand() % 500;
}
}
if (SDL_Init(SDL_INIT_VIDEO) != 0)
{
printf("SDL_Init Error: %s\n", SDL_GetError());
exit(1);
}
window = SDL_CreateWindow("Disk Scheduling", SDL_WINDOWPOS_UNDEFINED, SDL_WINDOWPOS_UNDEFINED, SCREEN_WIDTH, SCREEN_HEIGHT, SDL_WINDOW_SHOWN);
if (window == NULL)
{
printf("SDL_CreateWindow Error: %s\n", SDL_GetError());
exit(1);
}
renderer = SDL_CreateRenderer(window, -1, SDL_RENDERER_ACCELERATED | SDL_RENDERER_PRESENTVSYNC);
if (renderer == NULL)
{
printf("SDL_CreateRenderer Error: %s\n", SDL_GetError());
exit(1);
}
}
void close()
{
SDL_DestroyRenderer(renderer);
SDL_DestroyWindow(window);
SDL_Quit();
}
void draw_rect(int x, int y)
{
SDL_Rect rect = {x, y, RECT_WIDTH, RECT_HEIGHT};
SDL_RenderFillRect(renderer, &rect);
}
void draw_line(int x1, int y1, int x2, int y2)
{
SDL_RenderDrawLine(renderer, x1 + RECT_WIDTH / 2, y1 + RECT_HEIGHT / 2, x2 + RECT_WIDTH / 2, y2 + RECT_HEIGHT / 2);
}
void fcfs()
{
for (int i = 0; i < REQUEST_NUM; i++)
{
fcfs_track_moves += abs(requests[i] - fcfs_pos);
draw_line(fcfs_pos * RECT_WIDTH, 0, requests[i] * RECT_WIDTH, RECT_HEIGHT);
fcfs_pos = requests[i];
}
}
int find_nearest_request(int pos, int *visited)
{
int min_distance = TRACK_NUM;
int nearest_index = -1;
for (int i = 0; i < REQUEST_NUM; i++)
{
if (!visited[i])
{
int distance = abs(requests[i] - pos);
if (distance < min_distance)
{
min_distance = distance;
nearest_index = i;
}
}
}
return nearest_index;
}
void sstf()
{
int visited[REQUEST_NUM] = {0};
for (int i = 0; i < REQUEST_NUM; i++)
{
int nearest_index = find_nearest_request(sstf_pos, visited);
visited[nearest_index] = 1;
sstf_track_moves += abs(requests[nearest_index] - sstf_pos);
draw_line(sstf_pos * RECT_WIDTH, 2 * RECT_HEIGHT, requests[nearest_index] * RECT_WIDTH, 3 * RECT_HEIGHT);
sstf_pos = requests[nearest_index];
}
}
void look()
{
while (1)
{
int nearest_index = find_nearest_request(look_pos, NULL);
if (nearest_index == -1)
{
break;
}
look_track_moves += abs(requests[nearest_index] - look_pos);
draw_line(look_pos * RECT_WIDTH, 4 * RECT_HEIGHT, requests[nearest_index] * RECT_WIDTH, 5 * RECT_HEIGHT);
look_pos = requests[nearest_index];
}
}
void cscan()
{
int visited[REQUEST_NUM] = {0};
while (1)
{
int nearest_index = -1;
if (direction == 1)
{
nearest_index = find_nearest_request(cscan_pos, visited);
if (nearest_index == -1)
{
direction = -1;
continue;
}
if (requests[nearest_index] > cscan_pos && cscan_pos != 0)
{
draw_line(cscan_pos * RECT_WIDTH, 6 * RECT_HEIGHT, 0, 7 * RECT_HEIGHT);
cscan_track_moves += cscan_pos;
cscan_pos = 0;
draw_line(0, 7 * RECT_HEIGHT, requests[nearest_index] * RECT_WIDTH, 7 * RECT_HEIGHT);
}
else
{
draw_line(cscan_pos * RECT_WIDTH, 6 * RECT_HEIGHT, requests[nearest_index] * RECT_WIDTH, 7 * RECT_HEIGHT);
}
cscan_track_moves += abs(requests[nearest_index] - cscan_pos);
cscan_pos = requests[nearest_index];
visited[nearest_index] = 1;
}
else
{
nearest_index = find_nearest_request(cscan_pos, visited);
if (nearest_index == -1)
{
break;
}
if (requests[nearest_index] < cscan_pos && cscan_pos != TRACK_NUM - 1)
{
draw_line(cscan_pos * RECT_WIDTH, 6 * RECT_HEIGHT, (TRACK_NUM - 1) * RECT_WIDTH, 7 * RECT_HEIGHT);
cscan_track_moves += TRACK_NUM - 1 - cscan_pos;
cscan_pos = TRACK_NUM - 1;
draw_line((TRACK_NUM - 1) * RECT_WIDTH, 7 * RECT_HEIGHT, requests[nearest_index] * RECT_WIDTH, 7 * RECT_HEIGHT);
}
else
{
draw_line(cscan_pos * RECT_WIDTH, 6 * RECT_HEIGHT, requests[nearest_index] * RECT_WIDTH, 7 * RECT_HEIGHT);
}
cscan_track_moves += abs(requests[nearest_index] - cscan_pos);
cscan_pos = requests[nearest_index];
visited[nearest_index] = 1;
}
}
}
int main(int argc, char *argv[])
{
init();
SDL_Event event;
int quit = 0;
while (!quit)
{
while (SDL_PollEvent(&event))
{
if (event.type == SDL_QUIT)
{
quit = 1;
}
}
SDL_SetRenderDrawColor(renderer, 0, 0, 0, 255);
SDL_RenderClear(renderer);
SDL_SetRenderDrawColor(renderer, 255, 0, 0, 255);
fcfs();
SDL_SetRenderDrawColor(renderer, 0, 255, 0, 255);
sstf();
SDL_SetRenderDrawColor(renderer, 0, 0, 255, 255);
look();
SDL_SetRenderDrawColor(renderer, 255, 255, 0, 255);
cscan();
SDL_RenderPresent(renderer);
}
printf("FCFS track moves: %d\n", fcfs_track_moves);
printf("SSTF track moves: %d\n", sstf_track_moves);
printf("LOOK track moves: %d\n", look_track_moves);
printf("C-SCAN track moves: %d\n", cscan_track_moves);
close();
return 0;
}
```
需要注意的是,这只是一个简单的程序示例,可能还有一些细节需要完善,比如错误处理、内存管理等。同时,程序还可以优化,比如可以使用更高效的数据结构来加速查找最近的磁盘请求。
阅读全文
相关推荐
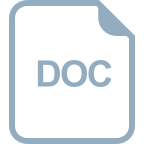
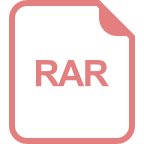
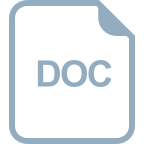
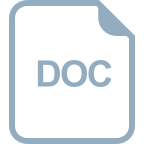
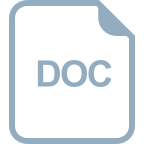
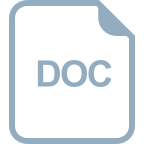
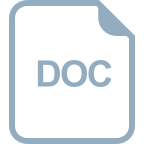
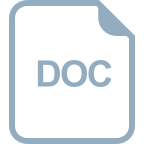
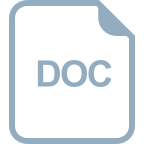
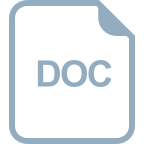
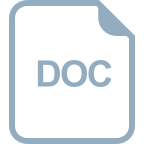



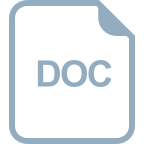
