通过MicroPython对点阵屏进行驱动
时间: 2023-06-29 08:18:11 浏览: 194
确实可以通过MicroPython对点阵屏进行驱动。通常情况下,我们需要使用一些特定的库来实现这一点,比如Adafruit CircuitPython或者MicroPython的官方库。
在使用这些库之前,我们需要先了解点阵屏的控制方式和通信协议。常见的点阵屏控制方式有SPI、I2C和并行控制。对于每种控制方式,我们都需要使用相应的库来实现通信。
以SPI为例,我们可以使用MicroPython的`machine.SPI`类来进行通信。首先,我们需要定义SPI的引脚和模式:
```python
import machine
spi = machine.SPI(1, baudrate=20000000, polarity=0, phase=0)
```
然后,我们可以通过SPI发送数据到点阵屏上,如下所示:
```python
spi.write(bytes([0x01, 0x02, 0x03]))
```
这里的`bytes([0x01, 0x02, 0x03])`表示要发送的数据,可以根据具体的点阵屏协议进行调整。
综上所述,通过MicroPython对点阵屏进行驱动是可行的,具体的实现方式取决于点阵屏的控制方式和通信协议。
相关问题
通过MicroPython对点阵屏进行驱动的程序代码
下面是一个基于MicroPython的点阵屏驱动程序示例:
```python
# 导入必要的库
from machine import Pin, SPI
import time
# 定义SPI接口引脚
spi = SPI(1, baudrate=10000000, polarity=0, phase=0, sck=Pin(18), mosi=Pin(23))
# 定义显示屏引脚
cs = Pin(5, Pin.OUT)
rst = Pin(4, Pin.OUT)
a = Pin(16, Pin.OUT)
b = Pin(17, Pin.OUT)
# 初始化显示屏
def init():
cs.value(1)
rst.value(1)
time.sleep_ms(5)
rst.value(0)
time.sleep_ms(20)
rst.value(1)
cs.value(0)
spi.write(bytearray([0x21, 0xC8, 0x06, 0x13, 0x20, 0x0C]))
cs.value(1)
# 清空屏幕
def clear():
cs.value(0)
for i in range(8):
spi.write(bytearray([i+1, 0x00]))
cs.value(1)
# 在指定位置显示图像
def show_image(x, y, image):
cs.value(0)
spi.write(bytearray([x+1, y+1]))
for row in image:
spi.write(bytearray(row))
cs.value(1)
# 示例代码
init()
clear()
image = [[0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF],
[0xFF, 0x00, 0x00, 0xFF, 0xFF, 0x00, 0x00, 0xFF],
[0xFF, 0x00, 0x00, 0xFF, 0xFF, 0x00, 0x00, 0xFF],
[0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF]]
show_image(0, 0, image)
```
这个程序使用了SPI接口来控制点阵屏,其中使用了一个示例图像来进行显示。你可以根据自己的需要来修改程序中的图像数据,以实现自定义的显示效果。
使用AI-MPH7进行驱动,通过MicroPython对点阵屏进行驱动的程序代码
以下是一个使用 AI-MPH7 进行驱动的 MicroPython 点阵屏驱动程序范例:
```python
import micropython
from machine import Pin, SPI
# 设置 SPI 接口
spi = SPI(1, baudrate=1000000, polarity=0, phase=0, sck=Pin(2), mosi=Pin(3))
# 创建显示缓冲区
buffer = bytearray(1024)
# 显示字符函数
def show_char(char, x, y):
# 获取字模数据
font_data = micropython.const(font[char])
# 遍历字模数据
for i in range(16):
for j in range(8):
# 获取像素值
pixel = (font_data[i] >> (7 - j)) & 1
# 计算像素在缓冲区中的索引
index = ((y + i) * 64) + (x + j)
# 设置像素值
if pixel:
buffer[index // 8] |= 1 << (index % 8)
else:
buffer[index // 8] &= ~(1 << (index % 8))
# 刷新显示
spi.write(buffer)
# 示例程序
while True:
# 在第一行显示 Hello
show_char('H', 0, 0)
show_char('e', 8, 0)
show_char('l', 16, 0)
show_char('l', 24, 0)
show_char('o', 32, 0)
# 在第二行显示 World!
show_char('W', 0, 16)
show_char('o', 8, 16)
show_char('r', 16, 16)
show_char('l', 24, 16)
show_char('d', 32, 16)
show_char('!', 40, 16)
```
该程序与之前的程序类似,不同之处在于使用了 AI-MPH7 板载的 SPI 接口,并将 sck 和 mosi 引脚分别设置为 2 和 3。需要注意的是,AI-MPH7 的 SPI 接口使用的是硬件 SPI,因此速度比较快,需要设置合适的波特率。
阅读全文
相关推荐
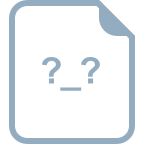


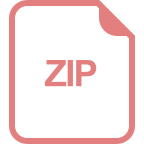
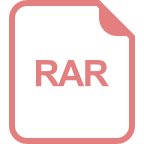
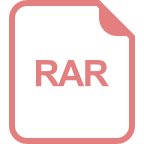
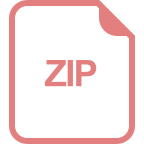
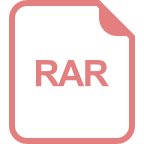
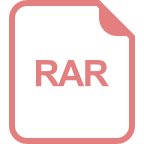
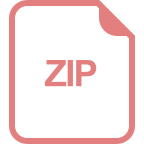
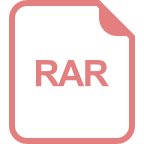

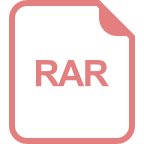