振动信号频谱分析代码python
时间: 2023-11-04 17:00:31 浏览: 58
很高兴为您提供振动信号频谱分析代码的Python实现!以下是代码示例:
```python
import numpy as np
import matplotlib.pyplot as plt
# 输入振动信号数据
sig = np.loadtxt('vibration_data.txt')
# 计算振动信号的快速傅里叶变换(FFT)
fft_sig = np.fft.fft(sig)
# 计算频率
freq = np.fft.fftfreq(sig.size, d=1/1000)
# 绘制频谱图
plt.plot(freq, np.abs(fft_sig))
plt.xlabel('Frequency (Hz)')
plt.ylabel('Amplitude')
plt.show()
```
请注意,此代码示例仅供参考,并且可能需要根据您的具体需求进行调整和修改。
相关问题
频谱分析_滚动轴承的频谱分析代码
滚动轴承的频谱分析代码需要用到信号处理的知识,以下是一个简单的示例代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 读取数据
data = np.loadtxt('data.txt')
# 定义采样频率
fs = 10000
# 傅里叶变换
freqs = np.fft.fftfreq(len(data), 1/fs)
fft = np.fft.fft(data)
# 取振动信号频率范围内的振动信号分量
freq_range = (0, 2000)
mask = (freqs >= freq_range[0]) & (freqs <= freq_range[1])
freqs = freqs[mask]
fft = fft[mask]
# 绘制频谱图
plt.plot(freqs, np.abs(fft))
plt.xlabel('Frequency (Hz)')
plt.ylabel('Amplitude')
plt.show()
```
其中,`data.txt` 是存储振动数据的文本文件,每行为一个采样点的振动信号值。`fs` 是采样频率。通过傅里叶变换将时域信号转换为频域信号,取出振动信号频率范围内的分量,并绘制频谱图。可以根据实际情况调整频率范围和绘图参数。
倒频谱齿轮故障诊断python代码
倒频谱齿轮故障诊断是一种齿轮故障诊断的方法,它利用倒频谱原理分析齿轮系统的振动信号,通过提取频率分量和相位信息来判断齿轮故障的类型和程度。以下是一个用Python实现倒频谱齿轮故障诊断的示例代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 模拟齿轮系统振动信号
fs = 1000 # 采样频率
t = np.arange(0, 1, 1 / fs)
f1 = 50 # 齿轮齿数差对应的频率
f2 = 200 # 故障对应的频率
x = np.sin(2 * np.pi * t * f1) + 0.5 * np.sin(2 * np.pi * t * f2)
# 进行FFT变换
N = len(x)
X = np.fft.fft(x)
X_mag = np.abs(X[:N//2])
f = np.linspace(0, fs/2, N//2)
# 对振动信号进行谱减法
threshold = 0.2 # 谱减法阈值
X_subtracted = np.where(X_mag > threshold, X_mag - threshold, 0)
# 进行倒频谱变换
X_inv = np.conj(X_subtracted[::-1])
# 进行逆FFT变换
x_inv = np.fft.ifft(X_inv)
x_inv = np.real(x_inv)
# 绘图显示结果
plt.figure()
plt.subplot(2, 1, 1)
plt.plot(t, x)
plt.xlabel('Time (s)')
plt.ylabel('Amplitude')
plt.title('Gear Vibration Signal')
plt.subplot(2, 1, 2)
plt.plot(t, x_inv)
plt.xlabel('Time (s)')
plt.ylabel('Amplitude')
plt.title('Inverse Frequency Spectrum Signal')
plt.tight_layout()
plt.show()
```
运行上述代码,将生成两幅子图。第一幅子图为齿轮系统振动信号波形图,第二幅子图为倒频谱变换后的信号波形图。倒频谱变换能够将频谱中的高频分量消除,突出低频成分,有助于故障分析人员判断齿轮故障类型以及故障程度。
相关推荐
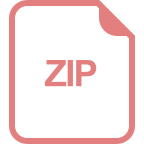
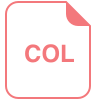
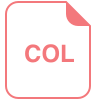
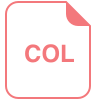
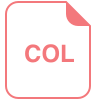
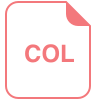







