分析一下an.setOnMouseClicked(e -> { List<CheckBox> list = (List<CheckBox>) (Object) vbox.getChildren(); final StringBuilder str = new StringBuilder("已选择:"); list.forEach(item -> { if (item.isSelected()) { str.append(item.getText() + ","); } }); System.out.println(str); });
时间: 2023-06-15 20:05:07 浏览: 76
这段代码是一个事件监听器,当鼠标点击an这个控件时,会执行其中的代码。
首先,它通过vbox.getChildren()获取到vbox这个容器中的所有子节点,然后将它们强制转换为List<CheckBox>类型的列表。
接着,它创建了一个StringBuilder对象str,用于拼接所选中的CheckBox的文本内容。
然后,它使用forEach循环遍历list中的所有CheckBox,如果某个CheckBox被选中,就将它的文本内容添加到str中。
最后,它将str打印到控制台。这样就可以实现多选框的监听和输出了。
相关问题
实现一个界面:包含文本输入框、RadioButton、CheckBox和按钮组件,文本输入框要能够对输入文本进行位数校验提示(文本7-10位),点击按钮,能够把组件的内容显示到消息框中。
好的,这是一个比较简单的界面实现问题。您可以使用Java Swing或JavaFX等GUI库来实现该界面。以下是一个JavaFX实现的示例代码:
```java
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.CheckBox;
import javafx.scene.control.RadioButton;
import javafx.scene.control.TextField;
import javafx.scene.control.ToggleGroup;
import javafx.scene.layout.HBox;
import javafx.scene.layout.VBox;
import javafx.scene.text.Text;
import javafx.stage.Stage;
public class MyGUI extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
// 创建文本输入框
TextField textField = new TextField();
textField.setPromptText("请输入7-10位文本");
// 创建RadioButton
RadioButton radioButton1 = new RadioButton("选项1");
RadioButton radioButton2 = new RadioButton("选项2");
RadioButton radioButton3 = new RadioButton("选项3");
ToggleGroup toggleGroup = new ToggleGroup();
radioButton1.setToggleGroup(toggleGroup);
radioButton2.setToggleGroup(toggleGroup);
radioButton3.setToggleGroup(toggleGroup);
// 创建CheckBox
CheckBox checkBox1 = new CheckBox("选项1");
CheckBox checkBox2 = new CheckBox("选项2");
CheckBox checkBox3 = new CheckBox("选项3");
// 创建按钮
Button button = new Button("提交");
button.setOnAction(event -> {
// 获取文本输入框的内容
String text = textField.getText();
// 校验文本输入框的内容
if (text.length() < 7 || text.length() > 10) {
new Text("请输入7-10位文本").show();
return;
}
// 获取RadioButton的选中项
RadioButton selectedRadioButton = (RadioButton) toggleGroup.getSelectedToggle();
String radioButtonValue = selectedRadioButton == null ? "" : selectedRadioButton.getText();
// 获取CheckBox的选中项
String checkBoxValue = "";
if (checkBox1.isSelected()) {
checkBoxValue += checkBox1.getText() + " ";
}
if (checkBox2.isSelected()) {
checkBoxValue += checkBox2.getText() + " ";
}
if (checkBox3.isSelected()) {
checkBoxValue += checkBox3.getText() + " ";
}
// 将组件的内容显示到消息框中
System.out.println("文本输入框的内容:" + text);
System.out.println("RadioButton的选中项:" + radioButtonValue);
System.out.println("CheckBox的选中项:" + checkBoxValue);
});
// 创建布局
VBox vBox = new VBox();
vBox.setSpacing(10);
vBox.setPadding(new Insets(10));
vBox.setAlignment(Pos.CENTER);
vBox.getChildren().addAll(textField, radioButton1, radioButton2, radioButton3, checkBox1, checkBox2, checkBox3, button);
// 创建场景
Scene scene = new Scene(vBox, 400, 400);
// 设置舞台
primaryStage.setTitle("MyGUI");
primaryStage.setScene(scene);
primaryStage.show();
}
}
```
这个界面包含了一个文本输入框、三个RadioButton、三个CheckBox和一个按钮组件。文本输入框能够对输入文本进行位数校验提示(文本7-10位),点击按钮,能够把组件的内容显示到消息框中。
请编写代码,实现TextView、Edittext、Button、ImageButton、CheckBox、RadioButton、Spinner和ListView的界面显示,并完成对Button、ImageButton、RadioButton、Spinner和ListView的鼠标监听事件
为了方便起见,我将使用JavaFX框架来实现这个界面。以下是一个简单的示例代码:
```java
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class UIExample extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
// 创建一个垂直布局
VBox root = new VBox();
root.setAlignment(Pos.CENTER);
root.setSpacing(10);
// 创建一个TextView
Label textView = new Label("TextView");
textView.setStyle("-fx-font-size: 16pt;");
// 创建一个EditText
TextField editText = new TextField();
editText.setPrefWidth(300);
// 创建一个Button
Button button = new Button("Button");
button.setPrefWidth(100);
button.setOnAction(event -> {
System.out.println("Button clicked!");
});
// 创建一个ImageButton
Image image = new Image("https://via.placeholder.com/50");
ImageView imageView = new ImageView(image);
Button imageButton = new Button("", imageView);
imageButton.setPrefWidth(50);
imageButton.setOnAction(event -> {
System.out.println("ImageButton clicked!");
});
// 创建一个CheckBox
CheckBox checkBox = new CheckBox("CheckBox");
checkBox.setOnAction(event -> {
System.out.println("CheckBox state changed: " + checkBox.isSelected());
});
// 创建一个RadioButton
RadioButton radioButton1 = new RadioButton("RadioButton1");
RadioButton radioButton2 = new RadioButton("RadioButton2");
ToggleGroup radioGroup = new ToggleGroup();
radioButton1.setToggleGroup(radioGroup);
radioButton2.setToggleGroup(radioGroup);
radioGroup.selectedToggleProperty().addListener((observable, oldValue, newValue) -> {
RadioButton selectedRadio = (RadioButton) newValue;
System.out.println("RadioButton selected: " + selectedRadio.getText());
});
// 创建一个Spinner
Spinner<Integer> spinner = new Spinner<>(0, 10, 5);
spinner.valueProperty().addListener((observable, oldValue, newValue) -> {
System.out.println("Spinner value changed: " + newValue);
});
// 创建一个ListView
ListView<String> listView = new ListView<>();
listView.getItems().addAll("Item 1", "Item 2", "Item 3");
listView.setOnMouseClicked(event -> {
String selectedItem = listView.getSelectionModel().getSelectedItem();
System.out.println("ListView item selected: " + selectedItem);
});
// 将所有控件添加到布局中
root.getChildren().addAll(textView, editText, button, imageButton, checkBox, radioButton1, radioButton2, spinner, listView);
root.setPadding(new Insets(10));
// 创建一个场景并显示
Scene scene = new Scene(root, 400, 400);
primaryStage.setScene(scene);
primaryStage.setTitle("UI Example");
primaryStage.show();
}
}
```
在这个示例代码中,我们创建了一个垂直布局,并向其中添加了TextView、EditText、Button、ImageButton、CheckBox、RadioButton、Spinner和ListView控件。我们还为Button、ImageButton、RadioButton、Spinner和ListView添加了鼠标监听事件,当用户点击它们时,它们会触发相应的事件处理程序。
阅读全文
相关推荐
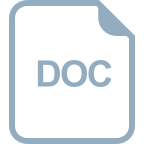
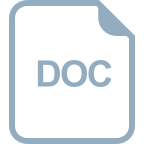
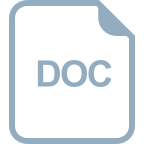
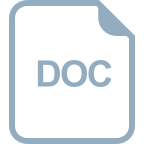
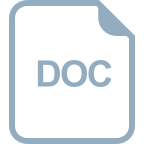
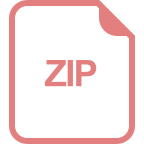
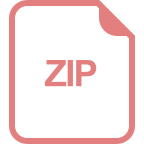
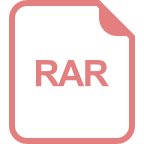
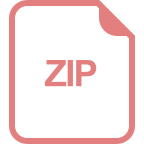
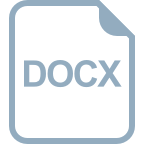