el-upload 上传文件 利用cryptojs 分块计算文件sha256
时间: 2023-11-02 13:01:22 浏览: 43
可以使用以下代码来利用cryptojs分块计算文件的sha256值:
```javascript
import CryptoJS from 'crypto-js';
// 定义分块大小
const CHUNK_SIZE = 1024 * 1024; // 1MB
// 计算文件sha256值
function calculateFileHash(file) {
return new Promise((resolve, reject) => {
const fileSize = file.size;
const chunkCount = Math.ceil(fileSize / CHUNK_SIZE);
let currentChunk = 0;
let sha256 = CryptoJS.algo.SHA256.create();
const fileReader = new FileReader();
fileReader.onload = function(e) {
const chunkData = CryptoJS.lib.WordArray.create(e.target.result);
sha256.update(chunkData);
currentChunk++;
if (currentChunk < chunkCount) {
processNextChunk();
} else {
const hash = sha256.finalize().toString();
resolve(hash);
}
};
fileReader.onerror = function(e) {
reject(e);
};
function processNextChunk() {
const start = currentChunk * CHUNK_SIZE;
const end = Math.min(start + CHUNK_SIZE, fileSize);
const blob = file.slice(start, end);
fileReader.readAsArrayBuffer(blob);
}
processNextChunk();
});
}
// 调用示例
const fileInput = document.getElementById('file-input');
fileInput.addEventListener('change', async () => {
const file = fileInput.files[0];
const hash = await calculateFileHash(file);
console.log('File SHA256:', hash);
});
```
这段代码定义了一个`calculateFileHash`函数,该函数接受一个文件对象作为参数,使用`FileReader`对文件进行分块读取,并在每个分块上使用CryptoJS计算SHA256哈希值。最终将所有分块的哈希值合并起来得到文件的SHA256值。在示例中,我们将该函数与一个文件选择框绑定,当用户选择文件后,就会自动计算文件的SHA256值并输出到控制台上。
相关推荐
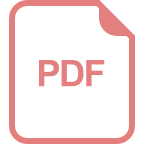
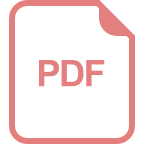














