pso算法MATLAB代码
时间: 2023-06-11 18:10:03 浏览: 44
以下是一个简单的PSO算法的MATLAB实现:
```
function [best_x, best_fval] = pso(fitness_func, dim, lb, ub, max_iter, swarm_size, w, c1, c2)
% PSO Particle Swarm Optimization algorithm
% fitness_func: the fitness function to optimize
% dim: the dimension of the search space
% lb: the lower bound of each dimension
% ub: the upper bound of each dimension
% max_iter: the maximum number of iterations
% swarm_size: the number of particles in the swarm
% w: the inertia weight
% c1: the cognitive parameter
% c2: the social parameter
% Initialize the swarm
swarm = lb + (ub-lb).*rand(swarm_size, dim);
swarm_vel = zeros(swarm_size, dim);
swarm_fitness = zeros(swarm_size, 1);
swarm_pbest = swarm;
swarm_pbest_fitness = swarm_fitness;
% Find the global best
[best_fval, gbest_idx] = min(swarm_pbest_fitness);
best_x = swarm_pbest(gbest_idx,:);
% Main loop
for iter=1:max_iter
% Update the velocity and position of each particle
for i=1:swarm_size
swarm_vel(i,:) = w*swarm_vel(i,:) ...
+ c1*rand(1,dim).*(swarm_pbest(i,:)-swarm(i,:)) ...
+ c2*rand(1,dim).*(best_x-swarm(i,:));
swarm(i,:) = swarm(i,:) + swarm_vel(i,:);
swarm(i,:) = max(swarm(i,:), lb);
swarm(i,:) = min(swarm(i,:), ub);
end
% Evaluate the fitness of each particle
for i=1:swarm_size
swarm_fitness(i) = fitness_func(swarm(i,:));
end
% Update the personal best of each particle
for i=1:swarm_size
if swarm_fitness(i) < swarm_pbest_fitness(i)
swarm_pbest(i,:) = swarm(i,:);
swarm_pbest_fitness(i) = swarm_fitness(i);
end
end
% Update the global best
[new_best_fval, new_gbest_idx] = min(swarm_pbest_fitness);
if new_best_fval < best_fval
best_fval = new_best_fval;
best_x = swarm_pbest(new_gbest_idx,:);
end
end
end
```
在使用该函数时,需要自己定义目标函数和输入参数。例如,假设要求解 Rosenbrock 函数,则可以这样调用该函数:
```
% Define the Rosenbrock function
fitness_func = @(x) 100*(x(2)-x(1)^2)^2 + (1-x(1))^2;
% Set the input parameters
dim = 2;
lb = [-5 -5];
ub = [5 5];
max_iter = 100;
swarm_size = 50;
w = 0.5;
c1 = 1;
c2 = 1;
% Call the PSO function
[best_x, best_fval] = pso(fitness_func, dim, lb, ub, max_iter, swarm_size, w, c1, c2);
```
相关推荐
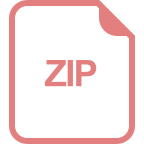













