php socket laravel,Laravel整合PHPSocket.Io实现web消息推送
时间: 2024-05-07 13:20:04 浏览: 5
要在 Laravel 中整合 PHPSocket.io 实现 web 消息推送,你需要遵循以下步骤:
1. 安装 PHPSocket.io
你可以使用 Composer 包管理器安装 PHPSocket.io。在终端中,导航到 Laravel 项目目录并运行以下命令:
```
composer require elephant-io/elephant.io
```
2. 配置 PHPSocket.io
在 Laravel 项目中,你需要在 `config/app.php` 文件中添加以下行:
```php
'aliases' => [
// ...
'ElephantIO' => 'ElephantIO\Engine\SocketIO\Version1X',
],
```
3. 创建 PHPSocket.io 服务提供者
在 Laravel 项目中,你需要创建 PHPSocket.io 服务提供者。运行以下命令来创建:
```
php artisan make:provider SocketIoServiceProvider
```
在 `SocketIoServiceProvider` 类中,你需要添加以下内容:
```php
use ElephantIO\Client;
use ElephantIO\Engine\SocketIO\Version1X;
public function register()
{
$this->app->singleton(Client::class, function ($app) {
$client = new Client(new Version1X('http://localhost:3000'));
return $client;
});
}
```
此代码将在 Laravel 应用程序中注册 `Client` 类的单例实例。
4. 创建推送事件
在 Laravel 项目中,你需要创建推送事件。运行以下命令来创建:
```
php artisan make:event PushNotification
```
在 `PushNotification` 类中,你需要添加以下内容:
```php
public $message;
public function __construct($message)
{
$this->message = $message;
}
public function broadcastOn()
{
return new PrivateChannel('push-notification');
}
```
此代码将创建一个名为 `PushNotification` 的事件类,并在构造函数中接受要推送的消息。`broadcastOn` 方法指定了事件应该广播到的频道。
5. 创建事件监听器
在 Laravel 项目中,你需要创建事件监听器。运行以下命令来创建:
```
php artisan make:listener PushNotificationListener
```
在 `PushNotificationListener` 类中,你需要添加以下内容:
```php
use ElephantIO\Client;
protected $socket;
public function __construct(Client $socket)
{
$this->socket = $socket;
}
public function handle(PushNotification $event)
{
$this->socket->initialize();
$this->socket->emit('push-notification', ['message' => $event->message]);
$this->socket->close();
}
```
此代码将创建一个名为 `PushNotificationListener` 的事件监听器,并在构造函数中注入 `Client` 实例。`handle` 方法将使用 `Client` 类向 Socket.io 服务器发送消息。
6. 注册事件和监听器
在 Laravel 项目中,你需要在 `EventServiceProvider` 类中注册事件和监听器。在 `EventServiceProvider` 类中,你需要添加以下内容:
```php
protected $listen = [
PushNotification::class => [
PushNotificationListener::class,
],
];
```
此代码将注册 `PushNotification` 事件和 `PushNotificationListener` 监听器。
7. 发送推送消息
在 Laravel 项目中,你可以使用以下代码发送推送消息:
```php
event(new PushNotification('Hello world!'));
```
这将触发 `PushNotification` 事件,并将消息发送到 Socket.io 服务器。然后,`PushNotificationListener` 监听器将从 Socket.io 服务器接收到消息并发送到连接到服务器的所有客户端。
以上是整合 PHPSocket.io 实现 web 消息推送的步骤。
相关推荐
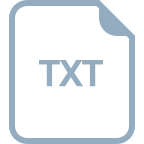
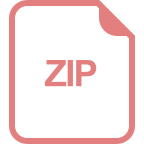














