pandas刷新excel
时间: 2023-07-09 20:50:53 浏览: 66
你可以使用 Pandas 库来读写 Excel 文件。如果你想刷新 Excel 文件,可以通过先读取 Excel 文件,修改数据,然后再将修改后的数据写入 Excel 文件来实现。以下是一个例子:
```python
import pandas as pd
# 读取 Excel 文件
df = pd.read_excel('example.xlsx')
# 修改数据
df.loc[df['Name'] == 'Alice', 'Age'] = 30
# 将修改后的数据写入 Excel 文件
with pd.ExcelWriter('example.xlsx') as writer:
df.to_excel(writer, index=False)
```
这个例子中,我们首先使用 `pd.read_excel()` 方法读取了名为 `example.xlsx` 的 Excel 文件,并将其存储在变量 `df` 中。然后,我们使用 Pandas 的数据切片功能来修改数据。最后,我们使用 `pd.ExcelWriter()` 方法来创建一个 Excel 文件写入器,并使用 `df.to_excel()` 方法将修改后的数据写入 Excel 文件中。
相关问题
用python将excel指定单元格写入TXT并实时刷新
您好,这个问题可以使用Python的pandas库来实现。首先,使用pandas的read_excel函数读取Excel文件,然后使用iloc函数指定单元格位置,将其写入TXT文件。最后,使用while循环实现实时刷新。具体代码如下:
```python
import pandas as pd
import time
# 读取Excel文件
df = pd.read_excel('example.xlsx')
# 指定单元格位置
cell_value = df.iloc[, ]
# 写入TXT文件
with open('example.txt', 'w') as f:
f.write(str(cell_value))
# 实时刷新
while True:
# 指定单元格位置
cell_value = df.iloc[, ]
# 写入TXT文件
with open('example.txt', 'w') as f:
f.write(str(cell_value))
# 等待1秒
time.sleep(1)
```
希望能对您有所帮助!
帮我用Python写一段代码,刷新本地的Excel文件,刷新之后把该Excel通过outlook发送出去
### 回答1:
当然,我可以帮你写一段Python代码,实现你的需求。你需要使用pandas库和win32com库来实现Excel文件的刷新和通过Outlook发送文件。
首先,你需要在终端或者命令行中输入以下命令来安装所需的库:
```
pip install pandas
pip install pywin32
```
然后,你可以使用以下代码来实现你的需求:
```python
import pandas as pd
import win32com.client as win32
# 读取Excel文件
df = pd.read_excel('path/to/your/excel/file.xlsx')
# 在这里进行Excel文件的刷新操作,比如修改数据或者添加新数据
# 保存Excel文件
df.to_excel('path/to/your/excel/file.xlsx', index=False)
# 发送邮件
outlook = win32.Dispatch('outlook.application')
mail = outlook.CreateItem(0)
mail.To = 'recipient@example.com'
mail.Subject = 'Your subject here'
mail.Body = 'Your email body here'
attachment = 'path/to/your/excel/file.xlsx'
mail.Attachments.Add(attachment)
mail.Send()
```
请注意将代码中的`'path/to/your/excel/file.xlsx'`和`'recipient@example.com'`替换为实际的文件路径和收件人邮箱地址。
### 回答2:
使用Python中的`openpyxl`库可以读取和修改Excel文件,使用`smtplib`库可以发送邮件。
首先,我们需要安装所需的库:
```
pip install openpyxl
pip install smtplib
```
接下来,我们可以编写如下代码来刷新Excel文件:
```python
import openpyxl
# 打开Excel文件
wb = openpyxl.load_workbook('example.xlsx')
# 选择需要刷新的工作表
ws = wb['Sheet1']
# 刷新单元格内容
ws['A1'] = 'Hello, World!'
# 保存修改后的Excel文件
wb.save('example.xlsx')
```
然后,我们可以编写如下代码来使用Outlook发送Excel文件:
```python
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.base import MIMEBase
from email import encoders
def send_email():
from_addr = 'your_email@example.com' # 发件人邮箱
to_addr = 'recipient@example.com' # 收件人邮箱
subject = 'Excel 文件' # 邮件主题
body = '请查收附件中的 Excel 文件。' # 邮件正文
attachment = 'example.xlsx' # 附件文件
# 创建邮件对象
msg = MIMEMultipart()
msg['From'] = from_addr
msg['To'] = to_addr
msg['Subject'] = subject
# 添加正文
msg.attach(MIMEText(body, 'plain'))
# 添加附件
part = MIMEBase('application', 'octet-stream')
part.set_payload(open(attachment, 'rb').read())
encoders.encode_base64(part)
part.add_header('Content-Disposition', 'attachment', filename=attachment)
msg.attach(part)
# 发送邮件
server = smtplib.SMTP('smtp.example.com', 587) # 邮件服务器和端口
server.starttls()
server.login(from_addr, 'your_password') # 发件人邮箱和密码
server.send_message(msg)
server.quit()
send_email()
```
确保将上述代码中的`example.xlsx`替换为要刷新和发送的实际Excel文件名,将`your_email@example.com`替换为发件人邮箱,将`recipient@example.com`替换为收件人邮箱,将`smtp.example.com`替换为您使用的邮件服务器地址,并填写发件人邮箱的密码。
最后,运行上述代码即可刷新Excel文件并将其作为附件通过Outlook发送出去。
### 回答3:
用Python刷新本地的Excel文件,然后将刷新后的Excel通过Outlook发送出去,可以使用以下代码实现:
```python
import win32com.client as win32
import pandas as pd
# 刷新Excel文件
def refresh_excel_file(excel_file_path):
excel = win32.Dispatch('Excel.Application')
workbook = excel.Workbooks.Open(excel_file_path)
workbook.RefreshAll()
workbook.Save()
workbook.Close()
excel.Quit()
# 发送Excel文件
def send_excel_file(excel_file_path, email_recipients):
outlook = win32.Dispatch('Outlook.Application')
mail = outlook.CreateItem(0)
mail.Subject = '刷新后的Excel文件'
mail.Body = '请查收附件中的Excel文件。'
mail.Recipients.Add(email_recipients)
mail.Attachments.Add(excel_file_path)
mail.Send()
# 主函数
def main():
excel_file_path = 'C:/path/to/excel_file.xlsx' # Excel文件的路径
email_recipients = 'example@example.com' # 邮件接收人的地址
# 刷新Excel文件
refresh_excel_file(excel_file_path)
# 发送Excel文件
send_excel_file(excel_file_path, email_recipients)
print('Excel刷新并已发送成功')
if __name__ == '__main__':
main()
```
请注意,运行此代码需要先安装相应的库,可以使用`pip install pywin32 pandas`命令进行安装。将代码中的`excel_file_path`替换为实际的Excel文件路径,`email_recipients`替换为实际的邮件接收人地址。执行代码后,将会自动刷新Excel文件并通过Outlook发送邮件,邮件主题为"刷新后的Excel文件",邮件正文为"请查收附件中的Excel文件。"
相关推荐







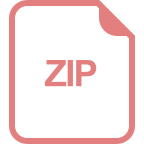
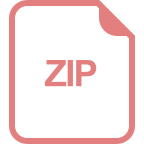
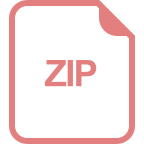
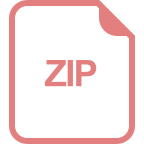
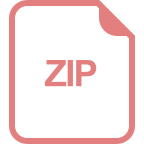