帮我用PHP写一个增删改查的源码程序,前后端分离
时间: 2023-10-30 11:06:04 浏览: 43
当可以!以下是一个简单的增删改查的源码程序,使用PHP语言和MySQL数据库,采用前后端分离的方式。
后端代码:
连接数据库:
```php
<?php
$hostname = "localhost"; // 数据库服务器地址
$username = "用户名"; // 数据库用户名
$password = "密码"; // 数据库密码
$database = "数据库名"; // 数据库名
// 创建连接
$conn = mysqli_connect($hostname, $username, $password, $database);
// 检查连接是否成功
if (!$conn) {
die("连接失败: " . mysqli_connect_error());
}
?>
```
增加数据:
```php
<?php
// 获取请求的JSON数据
$data = json_decode(file_get_contents('php://input'), true);
// 获取表单数据
$name = $data['name'];
$email = $data['email'];
$phone = $data['phone'];
// SQL 插入语句
$sql = "INSERT INTO contacts (name, email, phone) VALUES ('$name', '$email', '$phone')";
if (mysqli_query($conn, $sql)) {
$response = array('success' => true, 'message' => '数据添加成功!');
echo json_encode($response);
} else {
$response = array('success' => false, 'message' => '数据添加失败!');
echo json_encode($response);
}
mysqli_close($conn);
?>
```
删除数据:
```php
<?php
// 获取要删除的数据的 ID
$id = $_GET['id'];
// SQL 删除语句
$sql = "DELETE FROM contacts WHERE id=$id";
if (mysqli_query($conn, $sql)) {
$response = array('success' => true, 'message' => '数据删除成功!');
echo json_encode($response);
} else {
$response = array('success' => false, 'message' => '数据删除失败!');
echo json_encode($response);
}
mysqli_close($conn);
?>
```
修改数据:
```php
<?php
// 获取请求的JSON数据
$data = json_decode(file_get_contents('php://input'), true);
// 获取表单数据和要修改的数据的 ID
$id = $data['id'];
$name = $data['name'];
$email = $data['email'];
$phone = $data['phone'];
// SQL 更新语句
$sql = "UPDATE contacts SET name='$name', email='$email', phone='$phone' WHERE id=$id";
if (mysqli_query($conn, $sql)) {
$response = array('success' => true, 'message' => '数据更新成功!');
echo json_encode($response);
} else {
$response = array('success' => false, 'message' => '数据更新失败!');
echo json_encode($response);
}
mysqli_close($conn);
?>
```
查询数据:
```php
<?php
// SQL 查询语句
$sql = "SELECT * FROM contacts";
$result = mysqli_query($conn, $sql);
if (mysqli_num_rows($result) > 0) {
// 输出每行数据
$rows = array();
while($row = mysqli_fetch_assoc($result)) {
$rows[] = $row;
}
echo json_encode($rows);
} else {
$response = array('success' => false, 'message' => '没有数据!');
echo json_encode($response);
}
mysqli_close($conn);
?>
```
前端代码:
HTML:
```html
<!DOCTYPE html>
<html>
<head>
<title>增删改查</title>
</head>
<body>
<h1>联系人列表</h1>
<table>
<thead>
<tr>
<th>ID</th>
<th>姓名</th>
<th>电子邮件</th>
<th>电话</th>
<th>操作</th>
</tr>
</thead>
<tbody id="tableBody"></tbody>
</table>
<h1>添加联系人</h1>
<form id="addForm">
<label for="name">姓名</label>
<input type="text" name="name" id="name">
<br>
<label for="email">电子邮件</label>
<input type="text" name="email" id="email">
<br>
<label for="phone">电话</label>
<input type="text" name="phone" id="phone">
<br>
<button type="submit">添加</button>
</form>
<h1>修改联系人</h1>
<form id="editForm">
<input type="hidden" name="id" id="editId">
<label for="editName">姓名</label>
<input type="text" name="name" id="editName">
<br>
<label for="editEmail">电子邮件</label>
<input type="text" name="email" id="editEmail">
<br>
<label for="editPhone">电话</label>
<input type="text" name="phone" id="editPhone">
<br>
<button type="submit">保存</button>
</form>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script>
<script>
var app = new Vue({
el: '#app',
data: {
contacts: [],
editContact: null
},
mounted: function() {
this.refreshContacts();
},
methods: {
refreshContacts: function() {
axios.get('api/contacts.php')
.then(function(response) {
app.contacts = response.data;
})
.catch(function(error) {
console.log(error);
});
},
addContact: function() {
var name = document.getElementById('name').value;
var email = document.getElementById('email').value;
var phone = document.getElementById('phone').value;
axios.post('api/add-contact.php', {
name: name,
email: email,
phone: phone
})
.then(function(response) {
alert(response.data.message);
app.refreshContacts();
document.getElementById('addForm').reset();
})
.catch(function(error) {
console.log(error);
});
},
deleteContact: function(id) {
if (confirm('确定要删除吗?')) {
axios.get('api/delete-contact.php?id=' + id)
.then(function(response) {
alert(response.data.message);
app.refreshContacts();
})
.catch(function(error) {
console.log(error);
});
}
},
editContactForm: function(contact) {
this.editContact = contact;
document.getElementById('editId').value = contact.id;
document.getElementById('editName').value = contact.name;
document.getElementById('editEmail').value = contact.email;
document.getElementById('editPhone').value = contact.phone;
},
editContactSubmit: function() {
var id = document.getElementById('editId').value;
var name = document.getElementById('editName').value;
var email = document.getElementById('editEmail').value;
var phone = document.getElementById('editPhone').value;
axios.post('api/edit-contact.php', {
id: id,
name: name,
email: email,
phone: phone
})
.then(function(response) {
alert(response.data.message);
app.refreshContacts();
app.editContact = null;
document.getElementById('editForm').reset();
})
.catch(function(error) {
console.log(error);
});
}
}
});
</script>
</body>
</html>
```
注意:需要使用Vue.js和Axios.js库。
希望这个简单的增删改查的源码程序能够帮助到你!
相关推荐
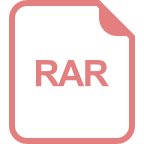
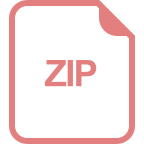














