Springboot2.7之后如何配置SpringSecurity的jwt配置
时间: 2024-02-18 16:30:32 浏览: 26
Spring Boot 2.7 还没有发布,可能是您想说的是 Spring Boot 2.4。
在 Spring Boot 2.4 中,配置 Spring Security 和 JWT 的步骤如下:
1. 添加依赖
在 pom.xml 文件中添加 Spring Security 和 JWT 的依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt-api</artifactId>
<version>0.11.2</version>
</dependency>
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt-impl</artifactId>
<version>0.11.2</version>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt-jackson</artifactId>
<version>0.11.2</version>
<scope>runtime</scope>
</dependency>
```
2. 配置 Spring Security
在 Spring Security 的配置类中,添加以下代码:
```java
@Configuration
@EnableWebSecurity
public class WebSecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private JwtTokenFilter jwtTokenFilter;
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.authorizeRequests()
.antMatchers("/api/authenticate").permitAll()
.anyRequest().authenticated()
.and()
.sessionManagement().sessionCreationPolicy(SessionCreationPolicy.STATELESS)
.and()
.addFilterBefore(jwtTokenFilter, UsernamePasswordAuthenticationFilter.class);
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user").password(passwordEncoder().encode("password")).roles("USER");
}
}
```
这个配置类中,我们首先禁用了 CSRF 防护,然后配置了访问控制,允许 `/api/authenticate` 路径的请求不需要认证,其他路径的请求需要进行认证。同时,我们还配置了使用 JWT 进行认证,通过 `JwtTokenFilter` 过滤器来实现。
3. 配置 JWT
在 JWT 的配置类中,添加以下代码:
```java
@Configuration
public class JwtConfig {
@Value("${jwt.secret}")
private String secret;
@Bean
public JwtParser jwtParser() {
return Jwts.parser().setSigningKey(secret);
}
@Bean
public JwtEncoder jwtEncoder() {
return Jwts.builder().setSigningKey(secret).build();
}
@Bean
public JwtTokenFilter jwtTokenFilter() {
return new JwtTokenFilter(jwtParser());
}
}
```
在这个配置类中,我们首先从配置文件中读取了 JWT 的密钥,然后配置了 `JwtParser` 和 `JwtEncoder` 对象,用于解析和生成 JWT。最后,我们还配置了 `JwtTokenFilter` 过滤器。这个过滤器用于在每个请求中解析 JWT,并将解析后的信息传递给 Spring Security 进行认证。
4. 编写 JwtTokenFilter
最后,我们需要编写 `JwtTokenFilter` 过滤器。这个过滤器用于在每个请求中解析 JWT,并将解析后的信息传递给 Spring Security 进行认证。具体代码如下:
```java
public class JwtTokenFilter extends OncePerRequestFilter {
private final JwtParser jwtParser;
public JwtTokenFilter(JwtParser jwtParser) {
this.jwtParser = jwtParser;
}
@Override
protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain chain) throws ServletException, IOException {
String header = request.getHeader("Authorization");
if (header != null && header.startsWith("Bearer ")) {
String token = header.substring(7);
try {
Jws<Claims> claimsJws = jwtParser.parseClaimsJws(token);
String username = claimsJws.getBody().getSubject();
List<SimpleGrantedAuthority> authorities = new ArrayList<>();
authorities.add(new SimpleGrantedAuthority("ROLE_USER"));
UsernamePasswordAuthenticationToken authenticationToken = new UsernamePasswordAuthenticationToken(username, null, authorities);
SecurityContextHolder.getContext().setAuthentication(authenticationToken);
} catch (Exception e) {
SecurityContextHolder.clearContext();
}
}
chain.doFilter(request, response);
}
}
```
在这个过滤器中,我们首先从请求头中获取 JWT,然后使用 `JwtParser` 对象对 JWT 进行解析。如果解析成功,我们就从 JWT 中获取用户名,并创建一个 `UsernamePasswordAuthenticationToken` 对象,用于在 Spring Security 中进行认证。最后,我们将认证信息保存到 `SecurityContextHolder` 中,以便于后续的处理。如果解析失败,我们就清空 `SecurityContextHolder` 中的认证信息。
相关推荐
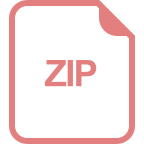
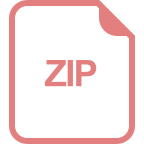














