RGB和HSV互相转换c++的代码
时间: 2024-05-02 15:17:03 浏览: 12
以下是RGB和HSV互相转换的C++代码:
```c++
#include <iostream>
#include <cmath>
using namespace std;
void rgbToHsv(float r, float g, float b, float& h, float& s, float& v) {
float cmax = max(max(r, g), b);
float cmin = min(min(r, g), b);
float delta = cmax - cmin;
if (delta == 0) {
h = 0;
} else if (cmax == r) {
h = 60 * fmod((g - b) / delta, 6);
} else if (cmax == g) {
h = 60 * ((b - r) / delta + 2);
} else {
h = 60 * ((r - g) / delta + 4);
}
if (cmax == 0) {
s = 0;
} else {
s = delta / cmax;
}
v = cmax;
}
void hsvToRgb(float h, float s, float v, float& r, float& g, float& b) {
int i = floor(h / 60.0);
float f = h / 60.0 - i;
float p = v * (1 - s);
float q = v * (1 - f * s);
float t = v * (1 - (1 - f) * s);
switch (i) {
case 0:
r = v; g = t; b = p;
break;
case 1:
r = q; g = v; b = p;
break;
case 2:
r = p; g = v; b = t;
break;
case 3:
r = p; g = q; b = v;
break;
case 4:
r = t; g = p; b = v;
break;
default:
r = v; g = p; b = q;
break;
}
}
int main() {
float r = 0.5f, g = 0.5f, b = 0.5f; // RGB值
float h, s, v;
rgbToHsv(r, g, b, h, s, v);
cout << "RGB(" << r << ", " << g << ", " << b << ") -> "
<< "HSV(" << h << ", " << s << ", " << v << ")" << endl;
hsvToRgb(h, s, v, r, g, b);
cout << "HSV(" << h << ", " << s << ", " << v << ") -> "
<< "RGB(" << r << ", " << g << ", " << b << ")" << endl;
return 0;
}
```
其中,`rgbToHsv`函数用于将RGB值转换为HSV值,`hsvToRgb`函数用于将HSV值转换为RGB值。在主函数中,我们可以测试转换结果。
相关推荐
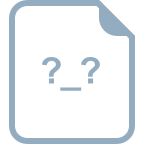














