to_string头文件
时间: 2023-08-03 14:06:06 浏览: 227
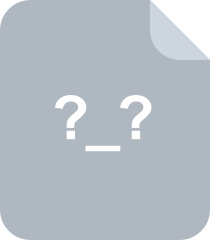
String类实现的头文件

`to_string` 是 C++11 中新增的一个函数,可以将数字类型转换为字符串类型。它的头文件为 `<string>`。
用法示例:
```c++
#include <iostream>
#include <string>
int main() {
int num = 123;
std::string str = std::to_string(num);
std::cout << str << std::endl; // 输出 "123"
return 0;
}
```
阅读全文
相关推荐














