springboot如何把list存入数据库,写出mapper
时间: 2023-11-16 08:15:45 浏览: 71
在Spring Boot中,我们可以使用MyBatis作为ORM框架来实现数据库操作。要将List存入数据库,我们需要在Mapper中编写SQL语句,然后在Service中调用Mapper中的方法进行操作。
以下是一个简单的示例,假设我们要将一个List<Student>存入数据库:
1. 创建实体类Student,包含学生的id、姓名、年龄等属性,并生成对应的Getter和Setter方法。
```java
public class Student {
private int id;
private String name;
private int age;
// Getter和Setter方法省略
}
```
2. 创建对应的Mapper接口StudentMapper,编写SQL语句。
```java
@Mapper
public interface StudentMapper {
@Insert("INSERT INTO student (id, name, age) VALUES (#{id}, #{name}, #{age})")
void insertStudent(Student student);
@Insert("<script>" +
"INSERT INTO student (id, name, age) VALUES " +
"<foreach collection=\"list\" item=\"item\" separator=\",\">" +
"(#{item.id}, #{item.name}, #{item.age})" +
"</foreach>" +
"</script>")
void insertStudentList(List<Student> studentList);
}
```
3. 在Service中调用Mapper中的方法进行操作。
```java
@Service
public class StudentService {
@Autowired
private StudentMapper studentMapper;
public void insertStudent(Student student) {
studentMapper.insertStudent(student);
}
public void insertStudentList(List<Student> studentList) {
studentMapper.insertStudentList(studentList);
}
}
```
在Mapper中,我们使用了MyBatis提供的foreach标签,将List中的每个元素都插入到数据库中。在Service中,我们直接调用Mapper中的方法,将List存入数据库即可。
需要注意的是,在Mapper接口上添加@Mapper注解,使得Spring Boot能够自动扫描并生成对应的Mapper实现类。同时,为了使用MyBatis的foreach标签,我们需要在Mapper接口中添加@Param注解,指定参数名为list。
相关推荐
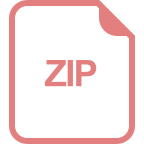
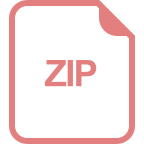
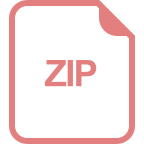














