编写c语言程序,给出具体代码: 位图图像文件缩放 a) 涉及知识点:文件读写、结构体定义、内存管理、基本图像处理算法、命令行参数 b) 要求: 编写一个程序,可以在命令行输入参数,完成指定文件的缩放,并存储到新文件,命令行参数如下 zoom file1.bmp 200 file2.bmp 第一个参数为可执行程序名称,第二个参数为原始图像文件名,第三个参数为缩放比例(百分比),第四个参数为新文件名
时间: 2024-02-22 21:59:33 浏览: 95
以下是一个简单的C语言程序,用于实现位图图像文件的缩放功能。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义位图文件头结构体
typedef struct {
char type[2]; // 文件类型,固定为“BM”
int size; // 文件大小
short reserved1; // 保留字1,固定为0
short reserved2; // 保留字2,固定为0
int offset; // 数据偏移量
} BMPHeader;
// 定义位图信息头结构体
typedef struct {
int size; // 信息头大小,固定为40
int width; // 图像宽度
int height; // 图像高度
short planes; // 颜色平面数,固定为1
short bits; // 每个像素的位数
int compression; // 压缩方式,0表示不压缩
int imagesize; // 图像数据大小
int xresolution; // 水平分辨率,像素/米
int yresolution; // 垂直分辨率,像素/米
int ncolors; // 颜色数,0表示使用所有颜色
int importantcolors;// 重要颜色数,0表示所有颜色都重要
} BMPInfoHeader;
// 函数声明
void zoom(char* src_file, char* dst_file, int percent);
int main(int argc, char* argv[]) {
if (argc != 4) {
printf("usage: %s src_file percent dst_file\n", argv[0]);
printf(" src_file: the source BMP file to be zoomed\n");
printf(" percent: the percent to zoom, 100 means no zoom\n");
printf(" dst_file: the destination BMP file to save the zoomed image\n");
return 1;
}
char* src_file = argv[1];
int percent = atoi(argv[2]);
char* dst_file = argv[3];
zoom(src_file, dst_file, percent);
return 0;
}
void zoom(char* src_file, char* dst_file, int percent) {
FILE* fp1 = fopen(src_file, "rb");
if (fp1 == NULL) {
printf("Cannot open %s\n", src_file);
exit(1);
}
BMPHeader header;
BMPInfoHeader info_header;
// 读取BMP文件头
fread(&header, sizeof(header), 1, fp1);
// 读取BMP信息头
fread(&info_header, sizeof(info_header), 1, fp1);
// 计算缩放后的宽度和高度
int new_width = info_header.width * percent / 100;
int new_height = info_header.height * percent / 100;
// 计算缩放后的图像数据大小
int new_imagesize = new_width * new_height * info_header.bits / 8;
// 分配缓冲区
unsigned char* buffer1 = (unsigned char*)malloc(info_header.imagesize);
unsigned char* buffer2 = (unsigned char*)malloc(new_imagesize);
// 读取原始图像数据
fread(buffer1, info_header.imagesize, 1, fp1);
// 关闭文件
fclose(fp1);
// 缩放图像
int x, y, new_x, new_y;
for (y = 0; y < info_header.height; y++) {
for (x = 0; x < info_header.width; x++) {
// 计算缩放后的坐标
new_x = x * percent / 100;
new_y = y * percent / 100;
// 将像素点复制到缩放后的位置
memcpy(buffer2 + (new_y * new_width + new_x) * info_header.bits / 8,
buffer1 + (y * info_header.width + x) * info_header.bits / 8,
info_header.bits / 8);
}
}
// 写入缩放后的图像数据
FILE* fp2 = fopen(dst_file, "wb");
if (fp2 == NULL) {
printf("Cannot create %s\n", dst_file);
exit(1);
}
fwrite(&header, sizeof(header), 1, fp2);
fwrite(&info_header, sizeof(info_header), 1, fp2);
fwrite(buffer2, new_imagesize, 1, fp2);
// 关闭文件
fclose(fp2);
// 释放内存
free(buffer1);
free(buffer2);
}
```
这个程序可以通过命令行参数指定要缩放的源文件和缩放比例,以及保存缩放后的图像文件。注意,这个程序只支持24位真彩色位图文件,其他类型的位图文件可能无法正常处理。
阅读全文
相关推荐





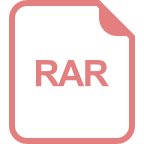
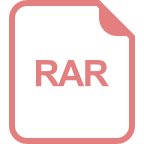












