用js帮我写一段打印标签的代码
时间: 2023-05-26 16:03:32 浏览: 49
以下是一个简单的打印标签的代码示例。它使用JavaScript的window.print()方法来打印页面。
```html
<!DOCTYPE html>
<html>
<head>
<title>打印标签示例</title>
</head>
<body>
<h1>打印标签示例</h1>
<p>这是一段需要打印的内容。</p>
<button onclick="window.print()">打印标签</button>
</body>
</html>
```
在该示例中,我们引入了一个按钮元素,它可以触发JavaScript函数 `window.print()`,该函数用于打开浏览器的打印对话框,并输出当前页面的内容。
相关问题
写一段比较基础的前端代码
当然,我可以为您提供一段基础的前端代码。以下是一个简单的HTML文件示例:
```html
<!DOCTYPE html>
<html>
<head>
<title>基础前端页面</title>
<style>
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 20px;
}
h1 {
color: #333;
}
p {
color: #666;
}
</style>
</head>
<body>
<h1>欢迎来到我的网页!</h1>
<p>这是一个基础的前端页面示例。</p>
<script>
// 在这里可以添加一些JavaScript代码
console.log("Hello, world!");
</script>
</body>
</html>
```
这段代码创建了一个简单的HTML页面,其中包含一个标题(h1)和一个段落(p)。页面中还包含一些基本的CSS样式,并且在JavaScript标签中打印了一条消息到控制台。您可以根据需要修改和扩展此代码。希望对您有所帮助!如果有更多问题,请随时提问。
用python写一段代码,要求提取网页的文本特征。
### 回答1:
好的,以下是用 Python 提取网页文本特征的代码:
```python
import requests
from bs4 import BeautifulSoup
url = "要提取特征的网页链接"
response = requests.get(url)
soup = BeautifulSoup(response.text, "html.parser")
text = soup.get_text()
print(text)
```
这段代码使用 requests 库获取网页内容,然后使用 BeautifulSoup 库解析 HTML 文档,并提取其中的文本内容。最后,将提取到的文本内容打印出来。
请注意,在使用这段代码时,需要安装 requests 和 BeautifulSoup 库。可以使用以下命令进行安装:
```
pip install requests
pip install beautifulsoup4
```
### 回答2:
要提取网页的文本特征,可以使用Python中的BeautifulSoup库和正则表达式模块re。
首先,我们需要使用requests库发送HTTP请求获取网页内容。然后,使用BeautifulSoup库对网页内容进行解析,提取出所有的文本内容。
下面是一段示例代码:
```python
import requests
from bs4 import BeautifulSoup
import re
def extract_text_features(url):
# 发送HTTP请求获取网页内容
response = requests.get(url)
# 使用BeautifulSoup解析网页内容
soup = BeautifulSoup(response.content, 'html.parser')
# 去除script和style标签,并获取纯文本内容
for script in soup(["script", "style"]):
script.extract()
text = soup.get_text()
# 去除多余空格和换行符
text = re.sub(r'\s+', ' ', text)
# 进一步清洗文本,可以根据需求进行预处理,如清除特殊字符、停用词等
# 返回提取的文本特征
return text
# 测试代码
url = "https://www.example.com"
text_features = extract_text_features(url)
print(text_features)
```
在该示例代码中,我们首先使用requests库发送HTTP请求获取网页内容,并使用BeautifulSoup库解析网页内容。然后,通过去除script和style标签,我们获取了网页的纯文本内容。最后,使用正则表达式re.sub函数将多余空格和换行符替换成一个空格。可以根据需求进一步对提取的文本进行清洗和预处理。最后,我们打印出提取的文本特征。
以上就是使用Python提取网页文本特征的一段示例代码。具体的文本特征提取方式可以根据实际需求进行定制和扩展。
### 回答3:
import requests
from bs4 import BeautifulSoup
import re
import nltk
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
def extract_text_features(url):
# 发送HTTP GET请求,获取网页内容
response = requests.get(url)
html_content = response.text
# 使用BeautifulSoup解析网页内容
soup = BeautifulSoup(html_content, 'html.parser')
# 去除HTML标签和JavaScript代码
text = soup.get_text(strip=True)
# 使用正则表达式去除特殊字符和数字
text = re.sub('[^a-zA-Z]+', ' ', text)
# 将文本转换为小写
text = text.lower()
# 分词
tokens = word_tokenize(text)
# 去除停用词
stop_words = set(stopwords.words('english'))
tokens = [token for token in tokens if token not in stop_words]
# 统计词频
word_freq = nltk.FreqDist(tokens)
# 提取最常见的前10个词作为文本特征
top_words = word_freq.most_common(10)
# 输出文本特征
for word, freq in top_words:
print(f"Word: {word}, Frequency: {freq}")
# 测试
url = 'https://www.example.com'
extract_text_features(url)
相关推荐
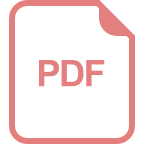
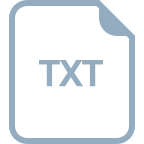
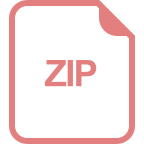











