在yolov5的外部写一个脚本,使用yolov5输出的检测结果,调用deepsort算法进行跟踪。具体应该怎么做
时间: 2024-05-06 12:20:28 浏览: 121
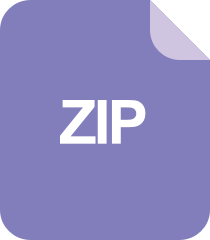
yolov5-使用YOLOv5在Android平台上实现获取USB摄像头进行图像目标检测算法.zip
以下是使用yolov5和deepsort算法进行目标跟踪的基本步骤:
1. 安装yolov5和deepsort算法的Python库。可以使用pip install命令安装,例如:
```
pip install yolov5
pip install deepsort
```
2. 使用yolov5进行目标检测,得到检测结果。可以使用yolov5提供的detect.py脚本,也可以自己编写Python代码进行调用。例如:
```
import torch
from yolov5.models.experimental import attempt_load
from yolov5.utils.general import non_max_suppression, scale_coords
from yolov5.utils.torch_utils import time_synchronized
# 加载模型
model = attempt_load('yolov5s.pt', map_location=torch.device('cpu'))
# 进行目标检测
img = torch.zeros((1, 3, 640, 640)) # 输入图片
img = img.float() / 255.0 # 归一化
t1 = time_synchronized()
pred = model(img, augment=False)[0] # 不使用增强
pred = non_max_suppression(pred, conf_thres=0.25, iou_thres=0.45)
t2 = time_synchronized()
print(f'Detection time: {t2 - t1:.3f}s')
print(f'Detection result: {pred}')
```
3. 将检测结果传入deepsort算法进行目标跟踪。可以使用deepsort提供的示例代码,也可以自己编写Python代码进行调用。例如:
```
from deepsort import DeepSort
from deepsort.detection import Detection
# 加载deepsort模型
deepsort = DeepSort("deep/checkpoint/ckpt.t7")
# 将检测结果转换为deepsort需要的格式
detections = []
for det in pred[0]:
x1, y1, x2, y2, conf, cls = det.cpu().numpy()
bbox = (x1, y1, x2 - x1, y2 - y1)
detections.append(Detection(bbox, conf, cls))
# 进行目标跟踪
outputs = deepsort.update(detections)
# 输出跟踪结果
for output in outputs:
bbox = output.tlwh
id = output.track_id
print(f'Track {id}: bbox={bbox}')
```
需要注意的是,以上代码仅为示例,实际应用中需要根据具体情况进行修改和优化。例如,可以将目标检测和目标跟踪的代码封装成一个函数,方便调用。还可以根据实际场景进行参数调优,例如目标检测的置信度阈值和IOU阈值,以及目标跟踪的相关参数。
阅读全文
相关推荐
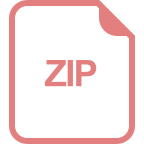
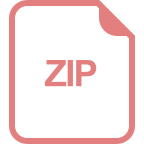















