写一个pytorch版本的CSPGhostNet的代码
时间: 2024-03-08 18:47:39 浏览: 67
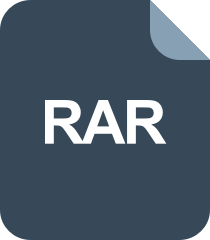
pytorch环境下ResNet示例代码
以下是基于PyTorch实现的CSPGhostNet模型代码示例:
```python
import torch
import torch.nn as nn
class GhostModule(nn.Module):
def __init__(self, in_channels, out_channels, kernel_size=1, ratio=2, dw_size=3, stride=1, relu=True):
super(GhostModule, self).__init__()
self.primary_conv = nn.Sequential(
nn.Conv2d(in_channels, out_channels // ratio, kernel_size, stride, kernel_size // 2, bias=False),
nn.BatchNorm2d(out_channels // ratio),
nn.ReLU(inplace=True) if relu else nn.Sequential()
)
self.cheap_operation = nn.Sequential(
nn.Conv2d(out_channels // ratio, out_channels // ratio, dw_size, 1, dw_size // 2, groups=out_channels // ratio, bias=False),
nn.BatchNorm2d(out_channels // ratio),
nn.ReLU(inplace=True) if relu else nn.Sequential(),
nn.Conv2d(out_channels // ratio, out_channels // ratio, kernel_size=1, bias=False),
nn.BatchNorm2d(out_channels // ratio),
nn.ReLU(inplace=True) if relu else nn.Sequential()
)
self.fusion_conv = nn.Conv2d(out_channels // ratio, out_channels, 1, 1, 0, bias=False)
self.bn = nn.BatchNorm2d(out_channels)
self.relu = nn.ReLU(inplace=True) if relu else nn.Sequential()
def forward(self, x):
primary_out = self.primary_conv(x)
cheap_out = self.cheap_operation(primary_out)
fusion_out = self.fusion_conv(cheap_out)
out = self.bn(fusion_out + primary_out)
return self.relu(out)
class CSPGhostNet(nn.Module):
def __init__(self, num_classes=1000, width_mult=1.0):
super(CSPGhostNet, self).__init__()
self.init_channel = int(16 * width_mult)
self.features = nn.Sequential(
nn.Conv2d(3, self.init_channel, kernel_size=3, stride=2, padding=1, bias=False),
nn.BatchNorm2d(self.init_channel),
nn.ReLU(inplace=True),
GhostModule(self.init_channel, int(16 * width_mult), kernel_size=3, stride=1, relu=True),
nn.Conv2d(int(16 * width_mult), int(24 * width_mult), kernel_size=3, stride=2, padding=1, bias=False),
nn.BatchNorm2d(int(24 * width_mult)),
nn.ReLU(inplace=True),
GhostModule(int(24 * width_mult), int(24 * width_mult), kernel_size=3, stride=1, relu=True),
GhostModule(int(24 * width_mult), int(24 * width_mult), kernel_size=3, stride=1, relu=True),
nn.Conv2d(int(24 * width_mult), int(40 * width_mult), kernel_size=1, stride=1, padding=0, bias=False),
nn.BatchNorm2d(int(40 * width_mult)),
nn.ReLU(inplace=True),
GhostModule(int(40 * width_mult), int(40 * width_mult), kernel_size=3, stride=1, relu=True),
nn.Conv2d(int(40 * width_mult), int(80 * width_mult), kernel_size=1, stride=1, padding=0, bias=False),
nn.BatchNorm2d(int(80 * width_mult)),
nn.ReLU(inplace=True),
GhostModule(int(80 * width_mult), int(80 * width_mult), kernel_size=3, stride=1, relu=True),
GhostModule(int(80 * width_mult), int(80 * width_mult), kernel_size=3, stride=1, relu=True),
GhostModule(int(80 * width_mult), int(80 * width_mult), kernel_size=3, stride=1, relu=True),
GhostModule(int(80 * width_mult), int(112 * width_mult), kernel_size=1, stride=1, relu=True),
nn.Conv2d(int(112 * width_mult), int(160 * width_mult), kernel_size=1, stride=1, padding=0, bias=False),
nn.BatchNorm2d(int(160 * width_mult)),
nn.ReLU(inplace=True),
GhostModule(int(160 * width_mult), int(160 * width_mult), kernel_size=3, stride=1, relu=True),
GhostModule(int(160 * width_mult), int(160 * width_mult), kernel_size=3, stride=2, relu=True),
GhostModule(int(160 * width_mult), int(160 * width_mult), kernel_size=3, stride=1, relu=True),
GhostModule(int(160 * width_mult), int(192 * width_mult), kernel_size=1, stride=1, relu=True),
GhostModule(int(192 * width_mult), int(192 * width_mult), kernel_size=3, stride=1, relu=True),
GhostModule(int(192 * width_mult), int(192 * width_mult), kernel_size=3, stride=1, relu=True),
GhostModule(int(192 * width_mult), int(192 * width_mult), kernel_size=3, stride=1, relu=True),
GhostModule(int(192 * width_mult), int(240 * width_mult), kernel_size=1, stride=1, relu=True),
GhostModule(int(240 * width_mult), int(240 * width_mult), kernel_size=3, stride=1, relu=True),
GhostModule(int(240 * width_mult), int(240 * width_mult), kernel_size=3, stride=1, relu=True),
GhostModule(int(240 * width_mult), int(240 * width_mult), kernel_size=3, stride=1, relu=True),
GhostModule(int(240 * width_mult), int(200 * width_mult), kernel_size=1, stride=1, relu=True),
nn.Conv2d(int(200 * width_mult), int(1280 * width_mult), kernel_size=1, stride=1, padding=0, bias=False),
nn.BatchNorm2d(int(1280 * width_mult)),
nn.ReLU(inplace=True),
)
self.avgpool = nn.AdaptiveAvgPool2d((1, 1))
self.fc = nn.Linear(int(1280 * width_mult), num_classes)
def forward(self, x):
x = self.features(x)
x = self.avgpool(x)
x = x.view(x.size(0), -1)
x = self.fc(x)
return x
```
CSPGhostNet模型是GhostNet系列中的一种变体,它使用了CSP(cross stage partial)结构来加速模型训练和推理速度。该模型使用了Ghost Module作为基本构建模块,其中包含了一个主要的卷积层和一个轻量级的操作。在模型中,使用了多个CSP结构和特征层的跨层连接,以提高模型性能。通过设置不同的width_mult参数,可以在保持模型精度的情况下,调整模型大小。
阅读全文
相关推荐
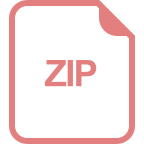
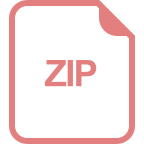
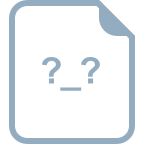
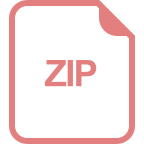
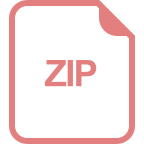
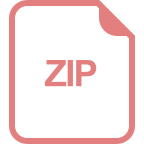
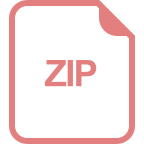
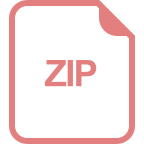
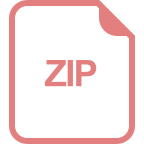
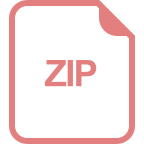
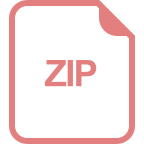
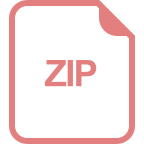
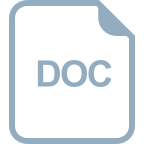
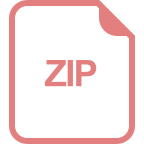
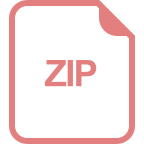
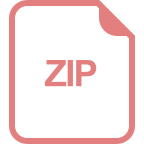