java微信支付后端代码
时间: 2023-10-17 19:05:46 浏览: 88
以下是使用Java实现微信支付后端代码的示例:
1. 创建统一下单请求对象
```java
public class UnifiedOrderRequest {
private String appid; // 公众账号ID
private String mch_id; // 商户号
private String nonce_str; // 随机字符串
private String body; // 商品描述
private String out_trade_no; // 商户订单号
private int total_fee; // 标价金额,单位为分
private String spbill_create_ip; // 终端IP
private String notify_url; // 通知地址
private String trade_type; // 交易类型
private String openid; // 用户标识
// 省略 getter 和 setter 方法
}
```
2. 创建统一下单响应对象
```java
public class UnifiedOrderResponse {
private String return_code; // 返回状态码
private String return_msg; // 返回信息
private String appid; // 公众账号ID
private String mch_id; // 商户号
private String nonce_str; // 随机字符串
private String sign; // 签名
private String result_code; // 业务结果
private String prepay_id; // 预支付交易会话标识
private String trade_type; // 交易类型
private String code_url; // 二维码链接
// 省略 getter 和 setter 方法
}
```
3. 发送统一下单请求
```java
public class WechatPayService {
private static final String UNIFIED_ORDER_URL = "https://api.mch.weixin.qq.com/pay/unifiedorder";
public UnifiedOrderResponse unifiedOrder(UnifiedOrderRequest request) throws Exception {
String xml = buildUnifiedOrderXml(request);
String responseXml = postXml(UNIFIED_ORDER_URL, xml);
return parseUnifiedOrderXml(responseXml);
}
private String buildUnifiedOrderXml(UnifiedOrderRequest request) throws Exception {
Map<String, Object> data = new HashMap<>();
data.put("appid", request.getAppid());
data.put("mch_id", request.getMch_id());
data.put("nonce_str", request.getNonce_str());
data.put("body", request.getBody());
data.put("out_trade_no", request.getOut_trade_no());
data.put("total_fee", request.getTotal_fee());
data.put("spbill_create_ip", request.getSpbill_create_ip());
data.put("notify_url", request.getNotify_url());
data.put("trade_type", request.getTrade_type());
data.put("openid", request.getOpenid());
data.put("sign", WechatPayUtil.sign(data, "商户密钥"));
return WechatPayUtil.mapToXml(data);
}
private UnifiedOrderResponse parseUnifiedOrderXml(String xml) throws Exception {
Map<String, String> data = WechatPayUtil.xmlToMap(xml);
UnifiedOrderResponse response = new UnifiedOrderResponse();
response.setReturn_code(data.get("return_code"));
response.setReturn_msg(data.get("return_msg"));
response.setAppid(data.get("appid"));
response.setMch_id(data.get("mch_id"));
response.setNonce_str(data.get("nonce_str"));
response.setSign(data.get("sign"));
response.setResult_code(data.get("result_code"));
response.setPrepay_id(data.get("prepay_id"));
response.setTrade_type(data.get("trade_type"));
response.setCode_url(data.get("code_url"));
return response;
}
private String postXml(String url, String xml) throws Exception {
HttpClient httpClient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost(url);
httpPost.setEntity(new StringEntity(xml, ContentType.APPLICATION_XML));
HttpResponse response = httpClient.execute(httpPost);
HttpEntity entity = response.getEntity();
String responseXml = EntityUtils.toString(entity);
EntityUtils.consume(entity);
return responseXml;
}
}
```
4. 处理支付结果通知
```java
public class WechatPayNotifyService {
public void handleNotify(HttpServletRequest request, HttpServletResponse response, String merchantKey) throws Exception {
String xml = IOUtils.toString(request.getInputStream(), StandardCharsets.UTF_8);
Map<String, String> data = WechatPayUtil.xmlToMap(xml);
if (WechatPayUtil.verifySign(data, merchantKey)) {
if ("SUCCESS".equals(data.get("return_code")) && "SUCCESS".equals(data.get("result_code"))) {
// 支付成功,处理业务逻辑
}
String responseXml = buildNotifyResponseXml("SUCCESS", "OK");
response.getWriter().write(responseXml);
} else {
String responseXml = buildNotifyResponseXml("FAIL", "签名验证失败");
response.getWriter().write(responseXml);
}
}
private String buildNotifyResponseXml(String returnCode, String returnMsg) {
Map<String, String> data = new HashMap<>();
data.put("return_code", returnCode);
data.put("return_msg", returnMsg);
return WechatPayUtil.mapToXml(data);
}
}
```
以上代码仅为示例,实际使用时需要根据具体业务需求进行适当调整。同时,为了确保交易的安全性,建议使用 HTTPS 协议进行通信,并对敏感信息进行加密处理。
阅读全文
相关推荐
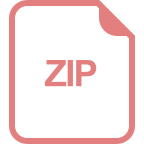
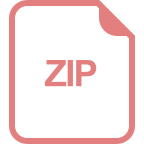
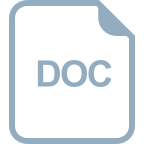
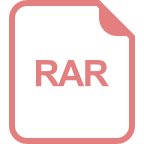
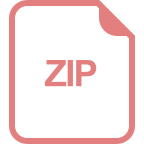
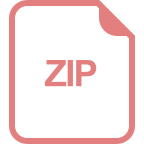
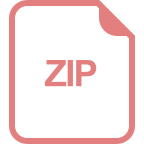
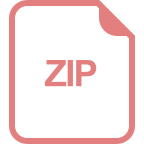
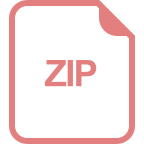
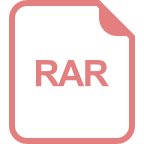
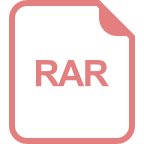
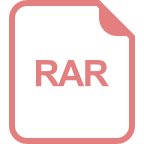
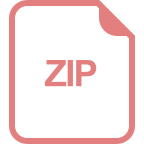
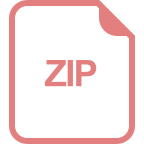
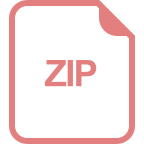
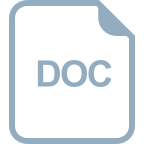